How to detect operating system on the client machine using JavaScript ?
Last Updated :
24 May, 2023
To detect the operating system on the client machine, one can simply use navigator.appVersion or navigator.userAgent property. The Navigator appVersion property is a read-only property and it returns a string that represents the version information of the browser.
Syntax
navigator.appVersion
Example 1: This example uses the navigator.appVersion property to display the operating system name.
html
<!DOCTYPE html>
< html >
< head >
< title >
How to detect operating system on the
client machine using JavaScript ?
</ title >
</ head >
< body style = "text-align:center;" >
< h1 style = "color:green;" >GeeksforGeeks</ h1 >
< button ondblclick = "operatingSytem()" >
Return Operating System Name
</ button >
< p id = "OS" ></ p >
< script >
function operatingSytem() {
let OSName = "Unknown OS";
if (navigator.appVersion.indexOf("Win") != -1) OSName = "Windows";
if (navigator.appVersion.indexOf("Mac") != -1) OSName = "MacOS";
if (navigator.appVersion.indexOf("X11") != -1) OSName = "UNIX";
if (navigator.appVersion.indexOf("Linux") != -1) OSName = "Linux";
// Display the OS name
document.getElementById("OS").innerHTML = OSName;
}
</ script >
</ body >
</ html >
|
Output:
Before Click on the Button:
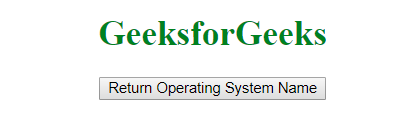
After Click on the Button:
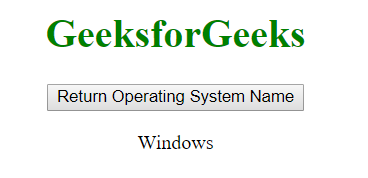
Example 2: This example uses the navigator.appVersion property to display all the properties of the client machine.
html
<!DOCTYPE html>
< html >
< head >
< title >
How to detect operating system on the
client machine using JavaScript ?
</ title >
</ head >
< body style = "text-align:center;" >
< h1 style = "color:green;" >GeeksforGeeks</ h1 >
< button ondblclick = "version()" >
Return OS Version
</ button >
< p id = "OS" ></ p >
< script >
function version() {
let os = navigator.appVersion;
// Display the OS details
document.getElementById("OS").innerHTML = os;
}
</ script >
</ body >
</ html >
|
Output:
Before Click on the Button:
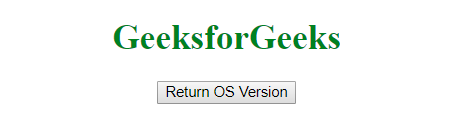
After Click on the Button: 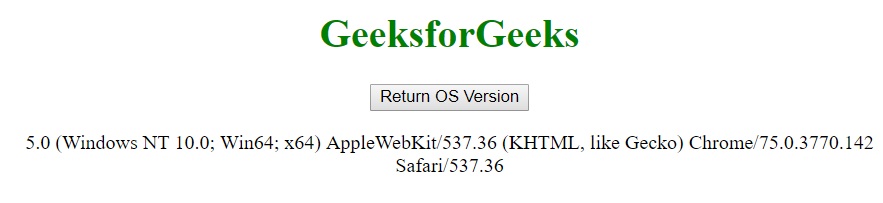
Share your thoughts in the comments
Please Login to comment...