How to Delete a Key from a MongoDB Document using Mongoose?
Last Updated :
15 Apr, 2024
MongoDB is a popular NoSQL database that stores data in flexible, JSON-like documents. When working with MongoDB using Mongoose, a MongoDB object modeling tool designed to work in an asynchronous environment and developers encounter scenarios where they need to delete a key from a document.
In this article, We will learn about the process of deleting a key from a MongoDB document using Mongoose by understanding the various methods along with the examples and so on.
How to Delete a key from a MongoDB document using Mongoose?
When working with MongoDB and Mongoose, developers often need to perform tasks such as updating documents, deleting keys, and executing complex queries. Below methods help us to understand How to Delete a key from a MongoDB document using Mongoose are as follow:
- Using the updateOne() method
- Using the findOneAndUpdate() method
Steps to Create Database Connection and Mongoose Schema
Step 1: Install Necessary Dependencies
npm install mongoose
Step 2: Create the MongoDB connection
- Create a dbConnect.js file and paste the below code
const mongoose = require("mongoose");
mongoose
.connect("mongodb://localhost:27017/gfg")
.then(() => {
console.log("Connected to MongoDB database successfully!");
})
.catch((err) => {
console.error("Error connecting to MongoDB:", err);
});
Step 3: Create a Mongoose Schema
- Create a model.js file, which includes the Mongo schema [courseSchema] with fields like Title, Fees, Instructor & Date.
const mongoose = require("mongoose");
const courseSchema = new mongoose.Schema({
Title: {
type: String,
},
Fees: {
type: Number,
},
Instructor: {
type: String,
},
date: Date,
});
const Course = mongoose.model("Course", courseSchema);
module.exports = Course;
Step 4: Query Delete a key from a MongoDB document using Mongoose Using Below Approaches
1. Using updateOne() Method with $unset Operator
- The updateOne() method is used to update a single document that matches the specified filter criteria in a collection. If multiple documents match the filter, only the first document encountered is updated.
Syntax:
Model.updateOne(
<filter>,
<update>,
[options],
[callback]
)
Explanation:
- Model: This is the Mongoose model that we want to update.
- filter: This is the filter that selects the document to update. It can be an object that specifies the query criteria.
- update: This is the update operator that specifies the changes to make to the document. In the example, we used `$unset` operator to delete the `address` field.
- options: This is an optional object that contains options for the update operation. For example, we can specify the `upsert` option to create a new document if no document matches the filter.
- callback: This is an optional callback function that is called when the update operation is complete. It takes an error object and a result object as arguments
Let’s set up an Environment
To understand How to Delete a key from a MongoDB document using Mongoose we need a collection and some documents on which we will perform various queries. Here we will consider a collection called courses which contains information like course name, Instructore name, fees and date of the courses in various documents.
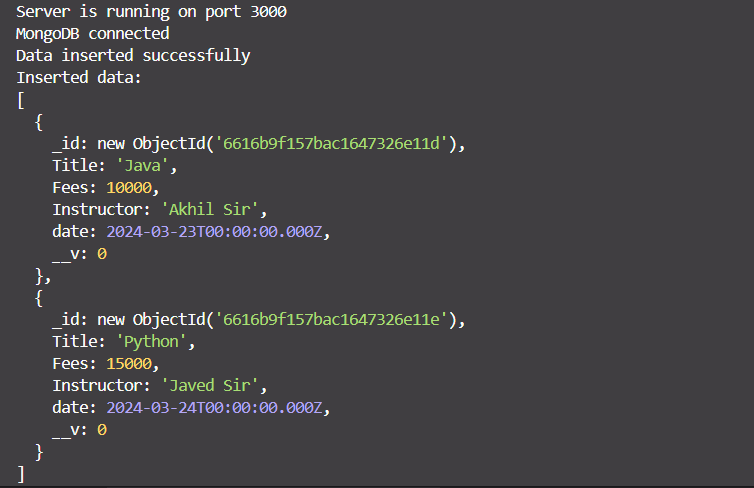
Example 1: Removing the Fees key from the document
Add the code into the queryData.js file and run using node queryData.js command
Query:
const mongoose = require("mongoose");
const Course = require("./model");
const db = require("./dbConnect");
async function deleteKeyFromDocument() {
try {
await Course.updateOne({}, { $unset: { Fees: 1 } });
console.log("Key deleted successfully from document");
const courses = await Course.find({});
console.log("Courses after deletion:");
console.log(courses);
} catch (error) {
console.error("Error deleting key:", error);
} finally {
mongoose.disconnect();
}
}
deleteKeyFromDocument();
Output:
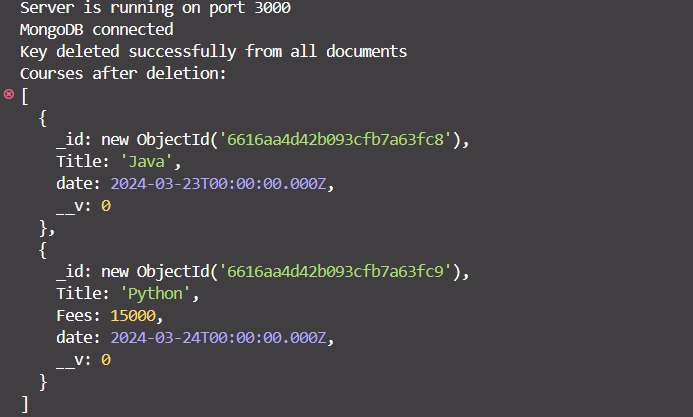
fees-1
Explanation:
- The async function uses the Course.updateOne() method to update the document in the collection by removing the Fees key from first document. with the help of the $unset operator.
- After the update operation, the function logs a success message to the console and then uses Course.find({}) to retrieve all the courses from the database. These courses are then logged to the console.
Example 2: Removing the Instrctutor key from the document
Query:
const mongoose = require("mongoose");
const Course = require("./model");
const db = require("./dbConnect");
async function deleteKeyFromDocument() {
try {
await Course.updateOne({}, { $unset: { Instructor: 1 } });
console.log("Key deleted successfully from document");
const courses = await Course.find({});
console.log("Courses after deletion:");
console.log(courses);
} catch (error) {
console.error("Error deleting key:", error);
} finally {
mongoose.disconnect();
}
}
deleteKeyFromDocument();
Output:
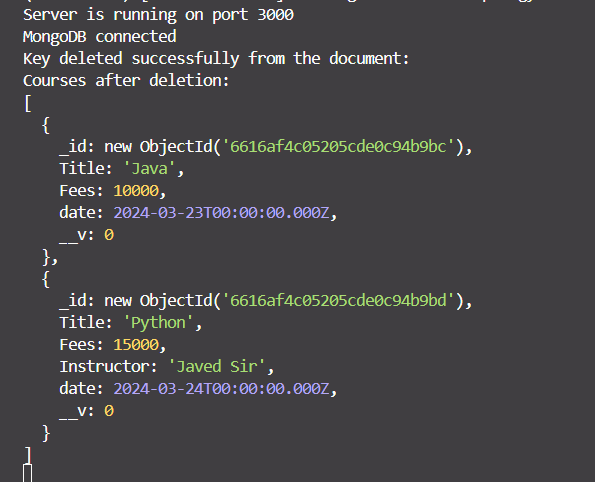
instructor
Explanation:
- The async function uses the Course.updateOne() method to update the document in the collection by removing the Instructor key from the first document. with the help of the $unset operator.
- After the update operation, the function logs a success message to the console and then uses Course.find({}) to retrieve all the courses from the database. These courses are then logged to the console.
2. Using findOneAndUpdate() Method with $unset Operator
In MongoDB, findOneAndUpdate() is a method that combines the functionality of findOne() and update() in a single atomic operation. This method used to find a single document that matches the specified filter criteria, update it, and return the updated document.
Syntax:
Model.findOneAndUpdate(filter, update, options, callback)
Explanation:
- filter: a query that matches the document to update.
- update: the update to apply to the document.
- options: an options object that contains additional settings for the update operation.
- callback: a callback function that is called when the update operation is complete.
Example 1: Removing the Fees key from the document
Query:
const mongoose = require("mongoose");
const Course = require("./model");
const db = require("./dbConnect");
async function deleteKeyFromDocument() {
try {
await Course.findOneAndUpdate(
{},
{ $unset: { Fees: 1 } },
{ new: true }
);
console.log("Key deleted successfully from the document:");
const courses = await Course.find({});
console.log("Courses after deletion:");
console.log(courses);
} catch (error) {
console.error("Error deleting key:", error);
} finally {
mongoose.disconnect();
}
}
deleteKeyFromDocument();
Output:
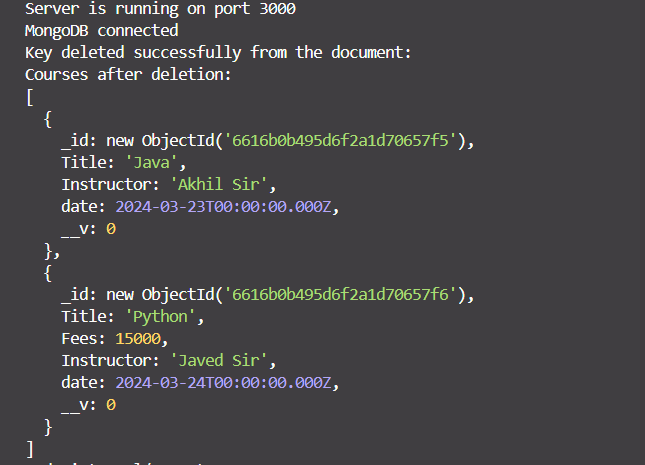
fees
Explanation:
- The async function uses the Course.findOneAndUpdate() method to update the document in the collection by removing the Fees key from first document. with the help of the $unset operator.
- After the update operation, the function logs a success message to the console and then uses Course.find({}) to retrieve all the courses from the database. These courses are then logged to the console.
Example 2: Removing the Instructor Key From the Document
Query:
const mongoose = require("mongoose");
const Course = require("./model");
const db = require("./dbConnect");
async function deleteKeyFromDocument() {
try {
await Course.findOneAndUpdate(
{},
{ $unset: { Instructor: 1 } },
{ new: true }
);
console.log("Key deleted successfully from the document:");
const courses = await Course.find({});
console.log("Courses after deletion:");
console.log(courses);
} catch (error) {
console.error("Error deleting key:", error);
} finally {
mongoose.disconnect();
}
}
deleteKeyFromDocument();
Output:
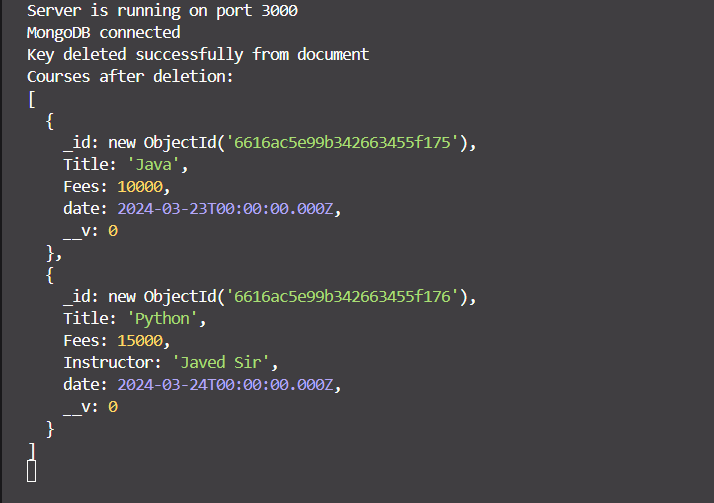
instructor
Explanation:
- The async function uses the Course.findOneAndUpdate() method to update the document in the collection by removing the Instructor key from the first document. with the help of the $unset operator.
- After the update operation, the function logs a success message to the console and then uses Course.find({}) to retrieve all the courses from the database. These courses are then logged to the console.
Conclusion
Overall, the Deleting a key from a MongoDB document using Mongoose is a straightforward process that involves using the findOneAndUpdate()
method with the $unset
operator. This approach allows you to modify MongoDB documents efficiently and is particularly useful when you need to remove unnecessary or sensitive data from your documents.
Share your thoughts in the comments
Please Login to comment...