How to define Optional Parameter in Scala?
Last Updated :
01 Apr, 2024
Optional parameters in Scala provide us with a procedure or a way to define some default values for function parameters. The need to define default values comes when a value is not provided for the parameter during function invocation. These are also useful when we want to provide some default or specific values for function parameters. We can control the behavior to an extent using Optional Parameters.
Here is a simple example showing optional parameters.
Example1: Simple Example with a single Optional parameter
Scala
object OptionalParametersExample1{
def item(sweetitem: String = "Chocolate"): Unit = {//Optional parameter
println("Sweet $sweetitem!")//print value either default or custom parameter
}
def main(args: Array[String]) {
//Calling item() with default parameter
item()//Output: Sweet Chocolate!
//Calling item() with custom parameter
item("Ice Cream")//Output: Sweet Ice Cream!
}
}
Definition:
- def <MethodName> (<VariableName/Parameter>: <Datatype> = “<DefaultValue>”): <Return Type> = {// code}
- def item (sweetitem: String = “Chocolate”): Unit = {//code}
Explanation:
- Created an object named “OptionalParametersExample1” in which we defined method item and another main method.
- Defined ‘item’ method with single parameter ‘sweetitem’ of String type. It has a default value ‘Chocolate’. This function prints a statement like – ‘Sweet Chocolate’.
- ‘Unit’ is similar to void in other languages which means it doesn’t return any value.
- The another main method serves as an entry point of the program. The program execution starts from here.
- The item method is called twice, first time when the method was invoked was without providing any arguments i.e. ‘item()’ and second time the method was invoked by passing an argument i.e. ‘item(“Ice Cream”)’.
Explanation of the Output:
- The execution starts from the main method.
- In main method, the ‘item()’ is invoked which reverts to the functionality of ‘item’ method. Since, no argument is provided, the method uses the default value set to the parameter. So the output when ‘item()’ is invoked comes out to be “Sweet Chocolate!”.
- When the ‘item’ method is invoked with passing argument, the default value is ignored and the argument which is passed becomes the value of the parameter and that will be used in place of the default one. So the output of second line i.e. item(“Chocolate”) is – “Sweet Ice Cream!”.
Output:
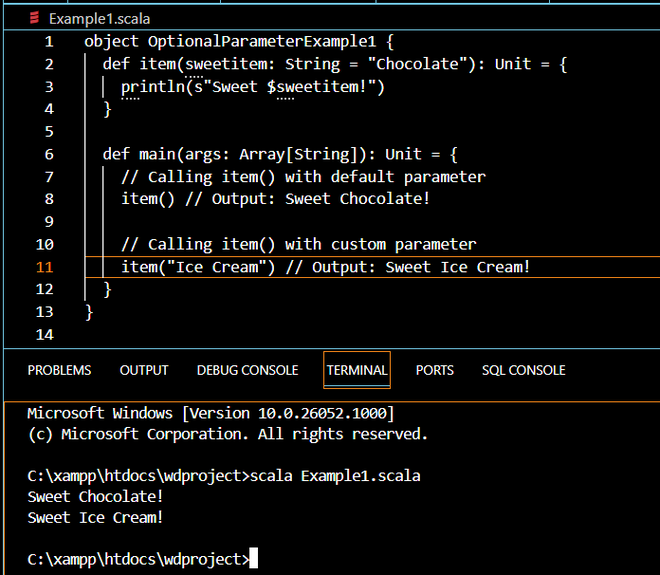
Example1
Lets see more examples for the same.
Example2: Simple Example with multiple Optional Parameter.
Scala
object OptionalParameterExample2{
def newname(noun: String = "Panda", adjective: String = "Fluffy"): Unit{
println("$adjective $noun")
}
def main(args: Array [String]): Unit {
//Calling item() with no arguments
newname()//Output: Fluffy Panda!
//Calling newname() by passing 2 arguments
newname("Animal","Cute")//Output: Cute Animal!
//Calling newname() with 1 argument
newname("Bear")//Output: Fluffy Bear!
}
}
Definition:
- def <MethodName> (<Parameter1>: <Datatype > == <DefaultValue1>, <Parameter2>: <Datatype> = <DefaultValue2>){//code}
- def newname(noun: String = “Panda”, adjective: String = “Fluffy”){//code}
Explanation:
- In above example-Example-2, we defined a method named ‘newname’ which takes 2 parameters and both the parameters has default values.
- In main method, we invoked the newname method thrice by passing arguments in different way or number.
Explanation of the Output:
- First, newname method is invoked with none arguments passed so it used default value set i.e. “Panda” and “Fluffy”. So this sets the output to be – “Fluffy Panda!”.
- Now, the second time the method is invoked by passing both the arguments i.e. “Animal” and “Cute”. This sets the output to be – “Cute Animal!”.
- And lastly, the method is invoked by passing single argument which is dedicated to “noun”. As we haven’t passed the second argument, the default argument is used for the adjective parameter and this sets the output to – “Fluffy Bear!”.
Output:
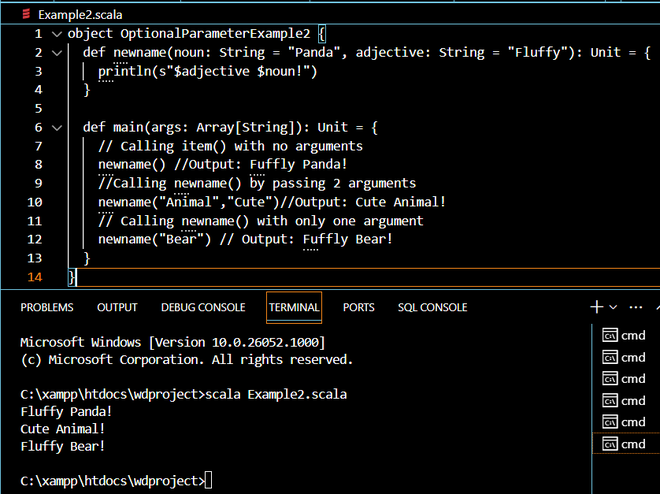
Example2
Example3: Simple Example of Optional Parameters with Different Datatypes.
Scala
object OptionalParametersExample3{
def marks(name: String = "Mihika", mark: Int = 95): Unit {
println("$name got $mark marks.")
}
def main(args: Array[String]){
marks()//Output: Mihika got 95 marks.
marks(mark = 70)//Output: Mihika got 70 marks.
marks("Nirali", 85)//Output: Nirali got 85 marks.
marks("Sourav")//Output: Sourav got 95 marks.
marks("Shravan", 100)//Output: Shravan got 100 marks.
}
}
Definition:
- def marks(name: String = “Mihika”, marks: Int = 95){//code}
Explanation:
- “marks” method is defined having 2 parameters of different datatypes i.e. one is string and another is number/Integer.
- This method takes two parameters and presents output in form of student and their marks
- The execution start from the main method.
Explanation of Output:
- First “marks()” is invoked and as you can see the method i called with no arguments, default values set to the parameters will be used. so the output will be – “Mihika got 95 marks.”.
- Next, the method is called by passing value to the second parameter and no argument or value is passed to the first one. In this case, the first argument will be set to the default value and the second argument or value considered will be the passed one. So the output is – “Mihika got 70 marks.”.
- Next, the method is called by passing both arguments and in such case, no default values are used and priority goes to the arguments passed. The output is set to be – “Nirali got 85 marks.”.
- Next, we can see that only one argument is passed without mentioning to which parameter it is passed to it by default sets that particular argument passed to the first parameter. This means the argument passed is equal to “name” and no second argument is passed so default value set will be used. The output comes out to be – “Sourav got 95 marks.”.
- At the last, the method is invoked by passed both the arguments which means the output turn out to be – “Shravan got 100 marks.”.
Output:
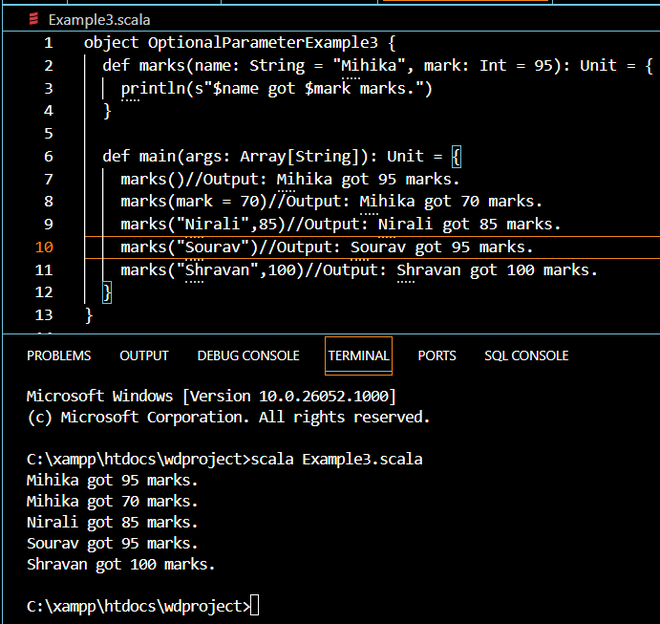
Example3
Share your thoughts in the comments
Please Login to comment...