How to Create Text Animation Effect using JavaScript ?
Last Updated :
15 Jan, 2024
Creating text animations in JavaScript can add an extra layer of interactivity and engagement to a website or application. By using JavaScript, we can create text that moves, fades, changes color, or transforms in other ways. Animating text can also help convey important information or messages in a visually appealing way. We are going to learn how to Create Text Animation Effects using JavaScript.
Approach
- HTML Setup:
- The HTML file contains a
<div>
with the id “animatedText” representing the text to be animated.
- CSS Styling:
- The initial style of the text is set using CSS, including font size, font weight, and an initial color.
- JavaScript Logic:
- The JavaScript script selects the element with the id “animatedText” using
document.getElementById
.
- The
changeColor
function is defined to change the text color by cycling through an array of colors.
- The
setInterval
function is used to call the changeColor
function every 1000 milliseconds (1 second), creating the animation effect.
- Color Array:
- An array named
colors
holds the colors to be used for the animation. You can customize this array with any colors you desire.
- Color Cycling:
- The
colorIndex
variable keeps track of the current color in the array, and it is incremented to cycle through the colors.
Example: This example describes the basic usage of JavaScript for creating Text Animation effects.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content = "width=device-width, initial-scale=1.0" >
< title >Text Animation Example</ title >
< style >
#animatedText {
font-size: 24px;
font-weight: bold;
color: blue;
/* Initial color */
}
</ style >
</ head >
< body >
< div id = "animatedText" >Hello, World!</ div >
< script >
const animatedText = document.getElementById('animatedText');
let colorIndex = 0;
function changeColor() {
const colors = ['red', 'green', 'blue', 'orange']; // Add more colors as needed
animatedText.style.color = colors[colorIndex];
colorIndex = (colorIndex + 1) % colors.length;
}
// Change text color every 1000 milliseconds (1 second)
setInterval(changeColor, 1000);
</ script >
</ body >
</ html >
|
Output:
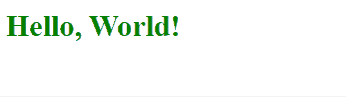
Share your thoughts in the comments
Please Login to comment...