How to Create Keyboard Shortcuts in JavaScript ?
Last Updated :
10 Oct, 2023
This article will demonstrate how to create keyboard shortcuts in JavaScript. We can manually set user-defined functions for different shortcut keys.
Keyboard shortcuts allow users to perform actions quickly by pressing a combination of keys, such as Ctrl + S for saving, Ctrl + C for copying, or custom shortcuts tailored to your web application’s needs. To implement these shortcuts, you need to define custom/user-defined functions that are triggered when the specified key combinations are pressed.
Syntax
Creating a basic keyboard shortcut in JavaScript typically involves the following syntax:
function handleShortcut(event) {
if (event.key === "YOUR_KEY") {
event.preventDefault();
// Your action to perform when the key is pressed
}
}
document.addEventListener("keydown", handleShortcut);
Here
- handleShortcut: This is the function that handles the keyboard shortcut.
- event.key: Represents the key that triggers the shortcut.
- event.preventDefault(): Prevents the default behavior associated with the key.
- document.addEventListener(“keydown”, handleShortcut): Listens for a “keydown” event on the document and calls the handleShortcut function when the specified key is pressed.
Approach for creating shortcuts in JavaScript
- Define Custom Functions: Create functions for each shortcut key action you want to implement. These functions contain the code to execute when the shortcut key is pressed.
- Listen for Keyboard Events: Use JavaScript’s event listeners to detect keyboard events, such as “keydown” or “keyup.” Assign these event listeners to specific elements, such as the document or a specific input field.
- Trigger Custom Functions: Within the event listener function, check if the pressed key combination matches the desired shortcut. If it matches, call the corresponding custom function to execute the desired action.
Example 1: Showing an Alert on “Ctrl + A”
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content = "width=device-width, initial-scale=1.0" >
< title >Custom Function Example
</ title >
< style >
h1 {
margin-left: 40%;
color: green;
}
p {
margin-left: 40%;
}
</ style >
</ head >
< body >
< h1 >GeeksforGeeks</ h1 >
< p >Press A for Showing an Alert </ p >
< script >
function showAlert() {
alert("A was pressed!");
}
document
.addEventListener("keydown",
function (event) {
if (event.key === "a") {
event.preventDefault();
showAlert();
}
});
</ script >
</ body >
</ html >
|
Output:
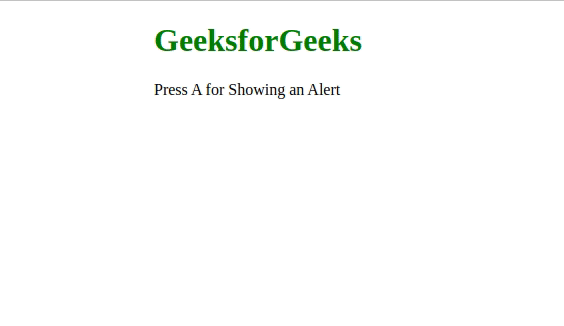
Example 2: Toggling Visibility on “Spacebar”
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content = "width=device-width, initial-scale=1.0" >
< title >Custom Function Example
</ title >
< style >
#element {
display: none;
}
h1 {
margin-left: 40%;
color: green;
}
p {
margin-left: 40%;
}
</ style >
</ head >
< body >
< p >Press Spacebar for showing
toggled element </ p >
< div id = "element" >
< h1 >GeeksforGeeks</ h1 >
</ div >
< script >
function toggleVisibility() {
const element = document
.getElementById("element");
element.style.display = element.style
.display === "none" ? "block" : "none";
}
document
.addEventListener("keydown",
function (event) {
if (event.key === " ") {
// Spacebar
event.preventDefault();
toggleVisibility();
}
});
</ script >
</ body >
</ html >
|
Output:
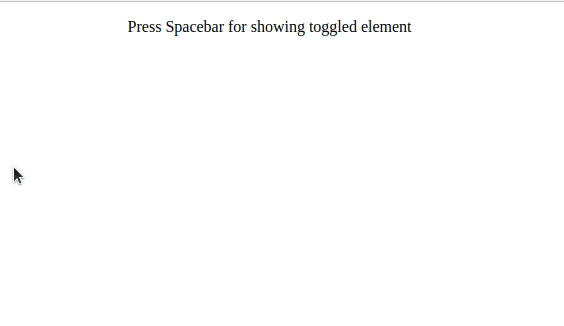
Example 3: Changing Background Color on “Y”
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content = "width=device-width, initial-scale=1.0" >
< title >Custom Function Example
</ title >
</ head >
< body >
< style >
h1 {
margin-left: 40%;
color: green;
}
p {
margin-left: 40%;
}
body {
background-color: white;
}
</ style >
< h1 >GeeksforGeeks</ h1 >
< p >Press Y for color change white to
yellow </ p >
< script >
function changeBackgroundColor() {
document.body.style.backgroundColor = "yellow";
}
document.addEventListener("keydown",
function (event) {
if (event.key === "y") {
event.preventDefault();
changeBackgroundColor();
}
});
</ script >
</ body >
</ html >
|
Output:
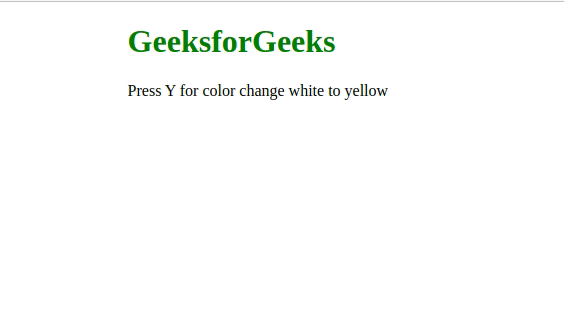
Example 4: Opening a Link in a New Tab on “O”
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content = "width=device-width, initial-scale=1.0" >
< title >Custom Function Example
</ title >
< style >
h1 {
margin-left: 40%;
color: green;
text-decoration: none;
outline: none;
}
p {
margin-left: 40%;
text-decoration: none;
outline: none;
color: black;
}
a {
text-decoration: none;
}
</ style >
</ head >
< body >
id = "myLink" >
< h1 >GeeksforGeeks</ h1 >
< p >Press O for Redirect on
newlink (website) </ p >
</ a >
< script >
function openLinkInNewTab() {
window.open(
document.getElementById(
"myLink").href, "_blank"
);
}
document
.addEventListener("keydown",
function (event) {
if (event.key === "o") {
event.preventDefault();
openLinkInNewTab();
}
});
</ script >
</ body >
</ html >
|
Output:
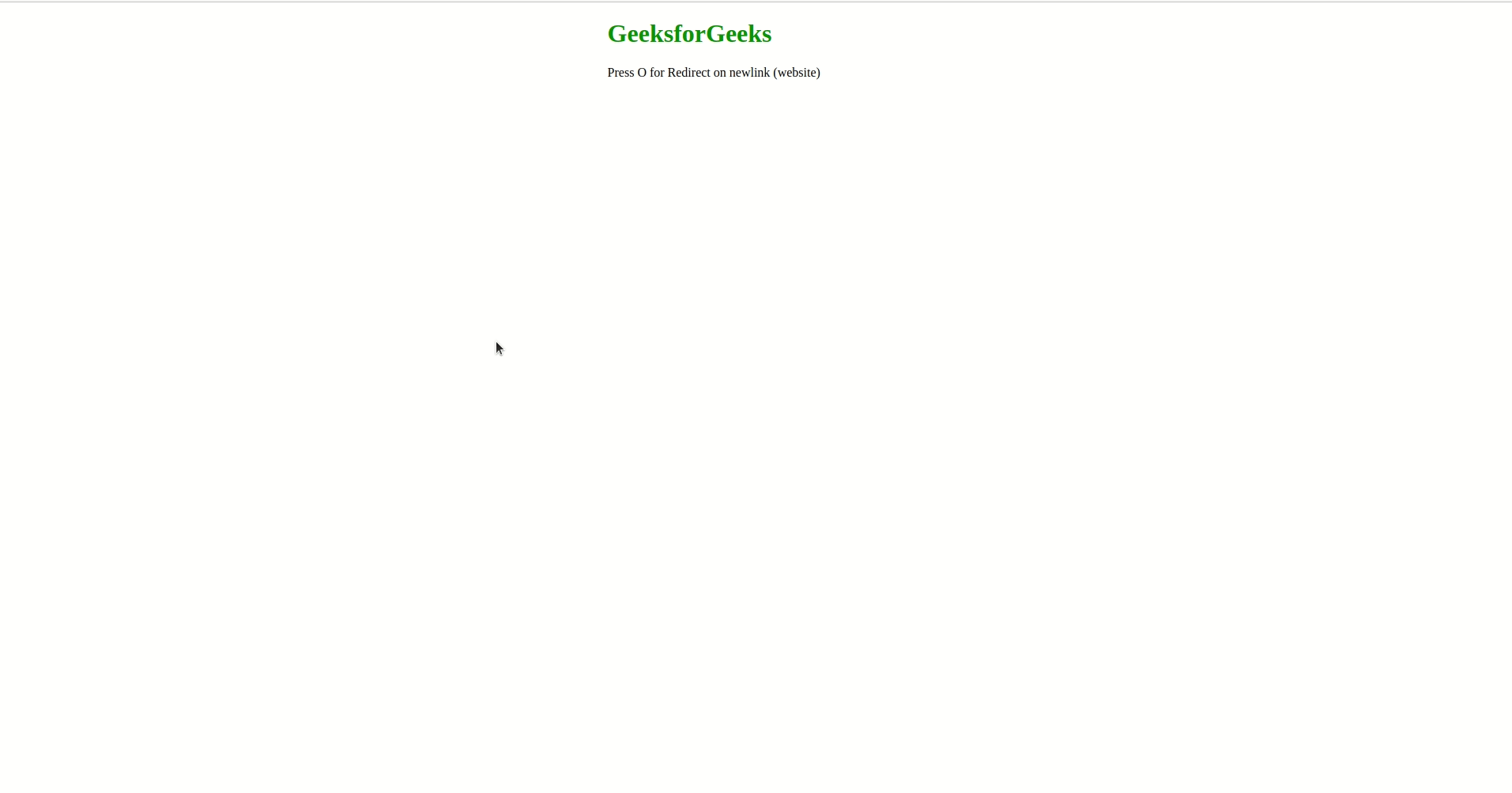
Conclusion
In conclusion, custom/user-defined functions for handling shortcut keys in web applications offer a powerful means to enhance user interactivity and productivity. These functions empower users to navigate and interact with the application more efficiently, reducing the learning curve and streamlining workflows. Additionally, the adaptability of custom shortcuts to the application’s specific requirements and the potential to boost user productivity make them a valuable feature for improving the overall user experience. By implementing these functions, web applications can provide a more user-friendly and efficient environment, ultimately leading to increased user satisfaction and success.
Share your thoughts in the comments
Please Login to comment...