How to create animated progress bar using react-bootstrap ?
Last Updated :
30 Nov, 2023
A progress bar is used to display the progress of a process on a computer. A progress bar displays how much of the process is completed and how much is left. You can add a progress bar on a web page using
- ProgressBar from ‘react-bootstrap/ProgressBar
- Predefined bootstrap classes.:
Here in this article, we will learn to build a progress bar in React using Bootstrap classes
Prerequisites:
Steps to Create the React Application And Installing Module:
Step 1: Create a react application using the following command
npx create-react-app foldername
Step 2: Once it is done change your directory to the newly created application using the following command
cd foldername
Step 3: Install Bootstrap dependency
npm install bootstrap
Project Structure:
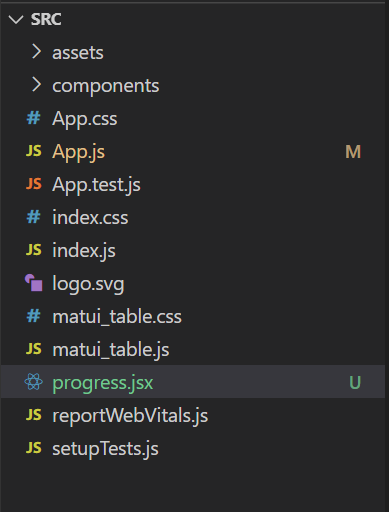
The updated dependencies in package.json file will look like:
"dependencies": {
"bootstrap": "^5.3.2",
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4",
}
Example: Now write down the following code in the App.js file
Javascript
import Progress from "./Progress" ;
function App() {
return (
<div className= "App" >
<Progress />
</div>
);
}
export default App;
|
Javascript
import React from "react" ;
import ProgressBar from 'react-bootstrap/ProgressBar'
function Progress() {
return (
<div>
<h1 style={{ color: 'green' }}>
<center>GeeksforGeeks</center>
</h1>
<div class= "container" >
<div className= "progress-bar bg-primary
progress-bar-striped
progress-bar-animated"
style={{ width: '50%' }}>50%</div>
<br></br>
<div className= "progress-bar bg-success
progress-bar-striped
progress-bar-animated"
style={{ width: '90%' }}>90%</div>
<br></br>
<div className= "progress-bar bg-warning
progress-bar-striped
progress-bar-animated"
style={{ width: '30%' }}>30%</div>
<br></br>
<div className= "progress-bar bg-danger
progress-bar-striped
progress-bar-animated"
style={{ width: '10%' }}>10%</div>
<br></br>
<div className= "progress-bar bg-info
progress-bar-striped
progress-bar-animated"
style={{ width: '70%' }}>70%</div>
</div>
</div>
)
}
export default Progress;
|
Step to Run the application: Enter the following command to run the application.
npm start
Output:
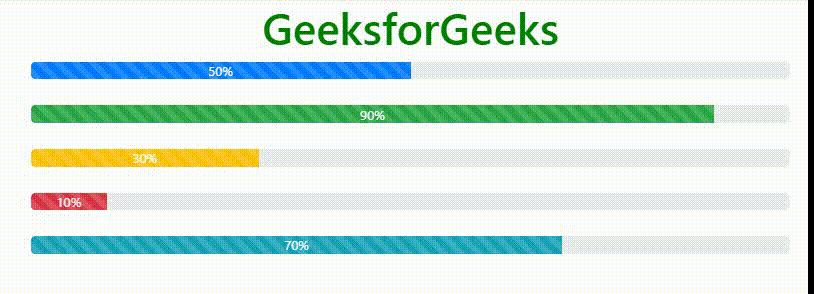
Share your thoughts in the comments
Please Login to comment...