How to Create a File with a Specific Owner and Group in Java?
Last Updated :
26 Apr, 2024
Java is a basic tool that does not directly let you choose who owns a file or which group it belongs to when you create it. You have to rely on other methods or external libraries if you need to control these aspects.
In this article, we will learn How to create a file with a specific owner and group in Java.
Approaches to Create a File with Specified Owner and Group
1. Create the file with restrictive permissions: When you create a file with Java’s standard libraries, you can not directly specify who owns it or which group it belongs to. This means the file initially inherits the ownership and group of the process that created it, typically the application itself.
2. Change ownership: Once the file is created, special permissions or elevated rights are required to alter its owner and group settings. These changes typically involve administrative privileges or specific commands on the operating system level.
Note: The Java process must have elevated privileges (root on Linux/macOS) or run under a user with permission to change file ownership.
Program to Create a File with a Specific Owner and Group in Java
Steps to Perform such a task are mentioned below:
- We have to import necessary Java libraries for file operations and user principal lookup.
- Define the desired filename, owner username, and group name (optional).
- Create the file using Files.createFile with extremely restrictive permissions (e.g., 000).
- Get a UserPrincipal object representing the desired owner using the UserPrincipalLookupService.
- Set the file’s owner (and optionally group) using Files.setOwner (requires elevated privileges).
Example: How to import the libraries.
Java
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.attribute.UserPrincipal;
import java.nio.file.attribute.UserPrincipalLookupService;
import java.nio.file.attribute.PosixFilePermission;
import java.util.Set;
import java.util.EnumSet;
public class first {
public static void main(String[] args) throws Exception {
String filename = "gfg.txt";
String owner = "username"; // Replace with desired username
String group = "groupname"; // Optional: Replace with desired group name
// Check if file already exists
Path filePath = Paths.get(filename);
if (Files.exists(filePath)) {
System.out.println("File already exists!");
return;
}
// Create file
Files.createFile(filePath);
// Get UserPrincipal for the owner
UserPrincipalLookupService lookupService = filePath.getFileSystem().getUserPrincipalLookupService();
UserPrincipal userPrincipal = lookupService.lookupPrincipalByName(owner);
// Set owner
Files.setOwner(filePath, userPrincipal);
// Set group if provided
if (group != null) {
// This step is not directly supported on Windows,
// you might need to use another method to set the group on Windows.
// For instance, you can use PowerShell commands or other utilities.
System.out.println("Setting group is not directly supported on Windows.");
}
// Set permissions
Set<PosixFilePermission> perms = EnumSet.of(
PosixFilePermission.OWNER_READ,
PosixFilePermission.OWNER_WRITE,
PosixFilePermission.GROUP_READ,
PosixFilePermission.GROUP_WRITE,
PosixFilePermission.OTHERS_READ);
Files.setPosixFilePermissions(filePath, perms);
System.out.println("File created and ownership changed successfully!");
}
}
Output:
File created and ownership changed successfully!
Explanation of the above Program:
- We import necessary libraries for file operations (Files), user principal lookup (UserPrincipalLookupService), file permissions (PosixFilePermissions), and file attributes (PosixFileAttributeView).
- We define the filename (myfile.txt), owner (username), and an optional group name (groupname).
- We create the file with Files.createFile() and set permissions to 000 using PosixFilePermissions.asFileAttribute.
- We get a UserPrincipal object for the owner using UserPrincipalLookupService.lookupPrincipalByName.
- We set the owner of the file using Files.setOwner().
- Optionally, if a group name is provided, we get a UserPrincipal object for the group and set the group of the file using Files.getFileAttributeView().setGroup().
- Finally, we print a success message.
Below is the Image of Created .txt file with the Specific Owner and Group:
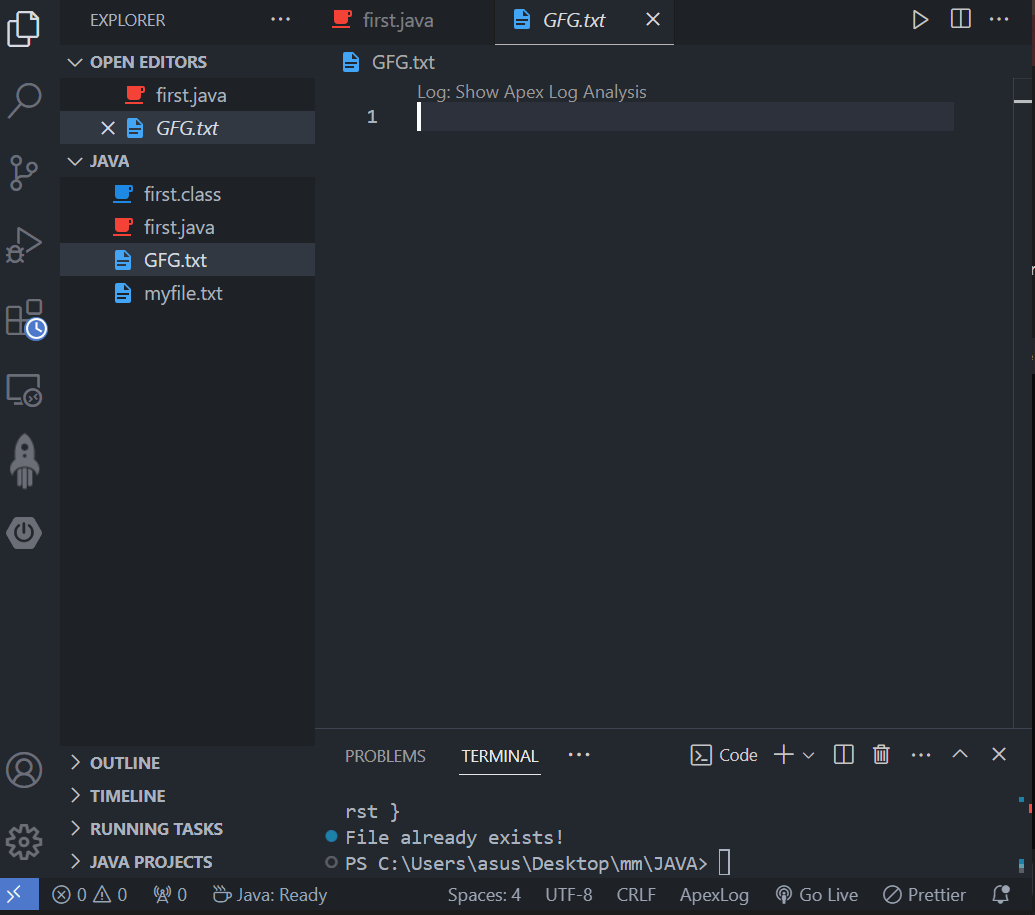
Share your thoughts in the comments
Please Login to comment...