How to Create a Desktop App Using JavaScript ?
Last Updated :
28 Dec, 2023
Building a desktop application with JavaScript is possible using various frameworks and technologies. One popular approach is to use Electron, which is an open-source framework developed by GitHub. Electron allows you to build cross-platform desktop applications using web technologies such as HTML, CSS, and JavaScript. In this article, we are going to learn how to build a desktop app with JavaScript.
Prerequisites:
Steps to build a desktop app using JavaScript and Electron:
Step 1: Install Node.js and npm
Make sure you have Node.js and npm (Node Package Manager) installed on your machine. You can download them from the official website: Node.js
Step 2: Create a new project:
Create a new directory for your project and navigate into it in the terminal.
mkdir my-electron-app
cd my-electron-app
Step 3: Initialize a new Node.js project
Run the following command to initialize a new package.json
file.
npm init -y
Step 4: Install Electron
Install Electron as a development dependency using npm.
npm install electron --save-dev
Step 5: Create main and renderer processes:
In your project directory, create two files, main.js
and index.html
. main.js
will be the main process, and index.html
will be the renderer process.
Example: This example shows the creation of a desktop app.
Javascript
const { app, BrowserWindow } = require( 'electron' );
let mainWindow;
function createWindow() {
mainWindow = new BrowserWindow({
width: 400,
height: 300,
webPreferences: {
nodeIntegration: true ,
},
});
mainWindow.loadFile( 'index.html' );
mainWindow.on( 'closed' , function () {
mainWindow = null ;
});
}
app.whenReady().then(createWindow);
app.on( 'window-all-closed' , function () {
if (process.platform !== 'darwin' ) app.quit();
});
app.on( 'activate' , function () {
if (mainWindow === null ) createWindow();
});
|
HTML
<!DOCTYPE html>
< html >
< head >
< title >Desktop App</ title >
</ head >
< body >
< h1 >Hello, Geeks!</ h1 >
</ body >
</ html >
|
Step 6: Update package.json
:
Modify the scripts
section in your package.json
file to include a start script.
"scripts": {
"start": "electron main.js"
},
Step 7: Run your Electron app:
Execute the following command to run your Electron app.
npm start
This will launch your desktop application with Electron. You can further customize and enhance your app by exploring the Electron API and integrating additional packages as needed.
Output:
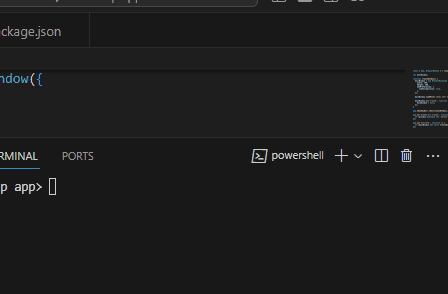
Share your thoughts in the comments
Please Login to comment...