How to convert an onload promise into Async/Await ?
Last Updated :
20 Nov, 2023
In this article, we will learn how to unload promise into Async/Await with multiple approaches.
An onload promise is a promise that is resolved when any onload event is fired onto a DOM ( Document Object Model ) element. The onload event is fired when the element has finished loading all of its resources, such as images and scripts. It’s like a commitment that happens when the element is all set and ready to go.
Promises are a way to handle asynchronous operations, representing a value that might not be available yet. It provides readable and manageable code by handling callbacks in a well-structured manner.
Async/Await is a modern method that simplifies working with promises. It provides a cleaner syntax by using async functions to deal with asynchronous methods, making code easier to understand and maintain. It also allows the use of await to handle promises in a more linear and synchronous style, enhancing code readability.
Example: In this example, we will see how we can fetch data by using onload promise.
Javascript
function fetchUserData(url) {
return new Promise((resolve, reject) => {
fetch(url)
.then(response => {
if (!response.ok) {
reject
( new Error( 'Failed in fetching the user data: '
+ url));
}
return response.json();
})
.then(userData => console.log(userData))
. catch (error => reject(error));
});
}
|
Output:
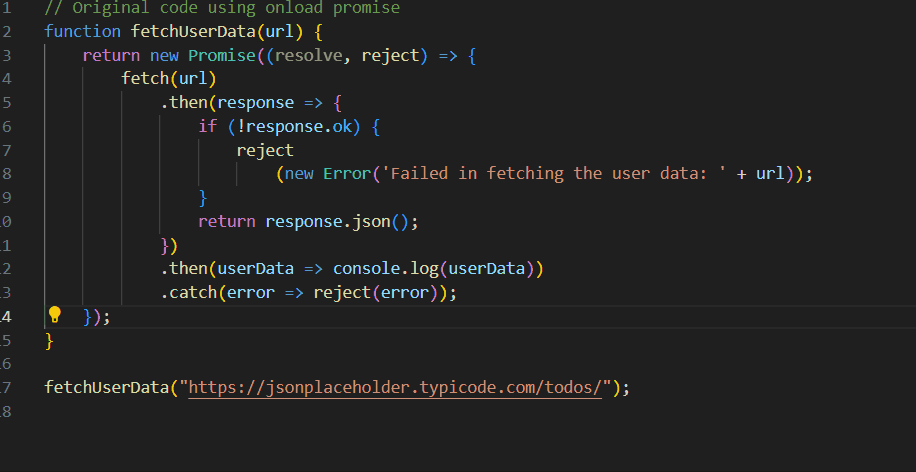
Output
Example 2: In this example, we will see how we can convert onload promise into Async/Await.
Javascript
async function fetchUserDataAsync(url) {
try {
const response = await fetch(url);
if (!response.ok) {
throw new Error
( 'Failed in fetching the user data: ' + url);
}
const userData = await response.json();
return userData;
} catch (error) {
throw new Error(error);
}
}
async function getUserInfo() {
try {
const userData = await
userData.forEach(item => {
console.log(`ID: ${item.id},
Title: ${item.title}`);
});
} catch (error) {
console.error( 'Error:' , error.message);
}
}
getUserInfo();
|
Explanation:
- The function fetchUserData employs the fetch API to retrieve user data from a specific URL, handling potential errors in a promise chain.
- Using async/await, the function fetchUserDataAsync streamlines the same process by awaiting the user data retrieval and subsequent error handling for improved readability.
- The getUserInfo function utilizes fetchUserDataAsync to retrieve user information, display the fetched data, or report an error in a user-friendly manner.
- Both fetchUserDataAsync and getUserInfo offer a cleaner way to handle asynchronous tasks, enhancing the code’s structure and readability for fetching user data.
- Output is shown by taking a dummy API and as a response data is fetched and shown on the console.
Output:
Share your thoughts in the comments
Please Login to comment...