How to convert a file to zip file and download it using Node.js ?
Last Updated :
06 Apr, 2023
The Zip files are a common way of storing compressed files and folders. In this article, I’ll demonstrate how to convert the file to zip format by using the adm-zip module (NPM PACKAGE).
Uses of ADM-ZIP
- compress the original file and change them to zip format.
- update/delete the existing files(.zip format).
Installation of ADM-ZIP:
Step 1: Install the module using the below command in the terminal.
npm install adm-zip
Step 2: Check the version of the installed module by using the below command
npm version adm-zip
Project Structure:
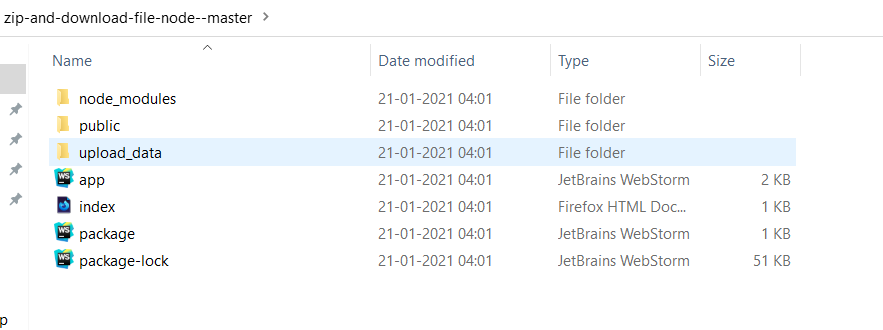
final project
We are going to change this upload_data folder to a zip file using the adm-zip module!
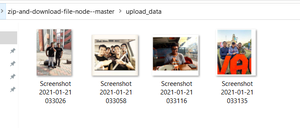
upload_data FOLDER
Code for conversion and downloading zip file:
Javascript
const express = require( 'express' )
const file_system = require( 'fs' )
const admz = require( 'adm-zip' )
const app = express()
var to_zip = file_system.readdirSync(__dirname + '/' + 'upload_data' )
app.get( '/' , function (req, res) {
res.sendFile(__dirname + '/' + 'index.html' )
const zp = new admz();
for (let k = 0; k < to_zip.length; k++) {
zp.addLocalFile(__dirname + '/' + 'upload_data' + '/' + to_zip[k])
}
const file_after_download = 'downloaded_file.zip' ;
const data = zp.toBuffer();
res.set( 'Content-Type' , 'application/octet-stream' );
res.set( 'Content-Disposition' , `attachment; filename=${file_after_download}`);
res.set( 'Content-Length' , data.length);
res.send(data);
})
app.listen(7777, function () {
console.log( 'port is active at 7777' );
})
|
Steps to run the program:
Open the terminal at the desired local and make sure that u have downloaded the adm-zip package using the following command.
npm install adm-zip
Run the app.js file using the following command.
node app.js
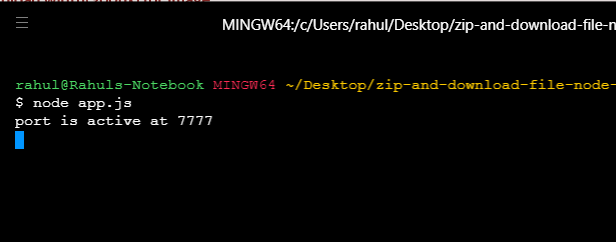
app is running
Open the browser and open localhost:7777 and then the upload_data folder gets converted to a zip file and gets downloaded!
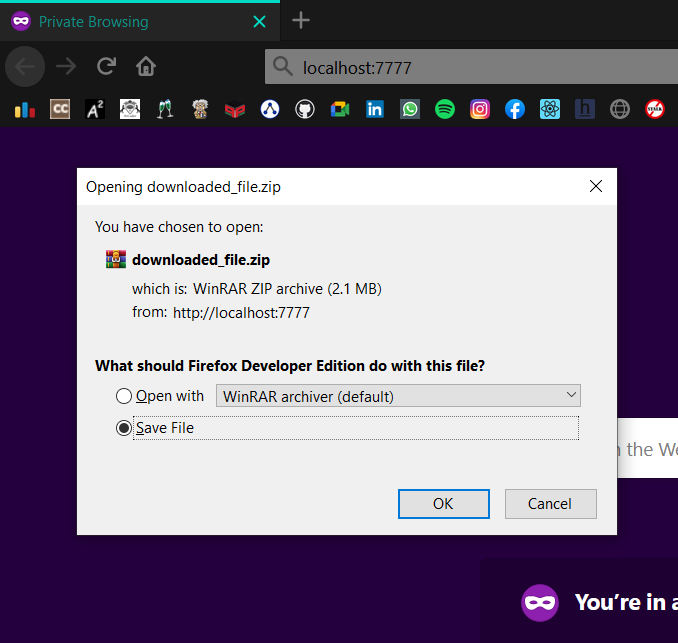
changed to zip file
Output: Representing the whole procedure for converting files to zip files with the help of the following gif, so in this way, you can change your folder to a zip file and then download it!
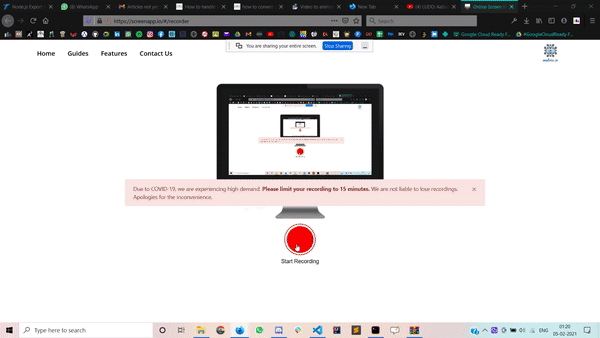
file to zip file
Share your thoughts in the comments
Please Login to comment...