How to check if an element exists with Python Selenium?
Last Updated :
11 Oct, 2023
Selenium is one of the most powerful and widely used tools for web automating web applications. Whether you’re a software developer or a QA tester, Selenium is an important tool to have in your toolkit. Selenium is widely used for automating user interactions like clicking buttons, filling out the forms and many more but in order to automate user interactions we first need to check if an element exists on a web page.
How to Check if an Element Exists with Python Selenium
Selenium is widely used for automating user interactions on a web page for testing or getting information from a web page. But in order to automate user interactions on a web page we first need to check if a particular element exists on a web page or not as we cannot click a button until it exists on a web page. So, in this article, we’ll show you a step-by-step tutorial on how to check if an element exists on a web page with Python and Selenium.
Prerequisite
STEP-1. Setting up the Development Environment
1. Make sure that you have Python installed in your System
2. Install the Python Selenium Package- Go to your terminal and write ‘pip install Selenium‘
3. In the latest versions of Selenium we don’t need to download Web Drivers .
You can refer to a detailed guide on How to Install Selenium in Python
STEP-2 . Installing the Libraries.
We need to import Web Driver, By and NoSuchElementException from Selenium .
- Web Driver- A web driver acts as a bridge between your testing code ( Python , Java or any other language) and your web browser you want to automate. It provides a way to control the web browser programmatically.
- NoSuchElementException – No such element exception is a common exception that occurs when the Selenium web Driver is unable to locate a element on a web page using the specified locator.
- By- By is a class that provides a way to locate a web element on a web page using various locators.
Python
from selenium import webdriver
from selenium.common.exceptions import NoSuchElementException
from selenium.webdriver.common.by import By
|
Approach
“In step 1, we’ll initialize the Selenium Web Driver and navigate to the web page using the driver.get(“www.google.com”) method. Here, ‘driver’ refers to the initialized Web driver.
In the next step, we’ll try to locate the element whose presence we want to check using various Locating Strategies. Now that we have located the element, we’ll check its presence using driver.find_element() with a suitable locating strategy. Here, ‘driver’ still refers to the initialized web driver. find_element() returns the element of class Selenium Web driver; otherwise, it throws a NoSuchElementFound exception, so we’ll use Exception Handling to tackle that.”
Implementation
STEP-1. Initialize the Web Web Driver And Navigate to the Web page.
Now we have to initialize the web driver and navigate to the web page, in this article we’ll use Chrome Web browser . Navigate to the website that you want to check the presence of an element
Python
from selenium import webdriver
from selenium.common.exceptions import NoSuchElementException
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
driver.get(url)
|
OUTPUT– It will open the url in a new Chrome browser and will close in few seconds.
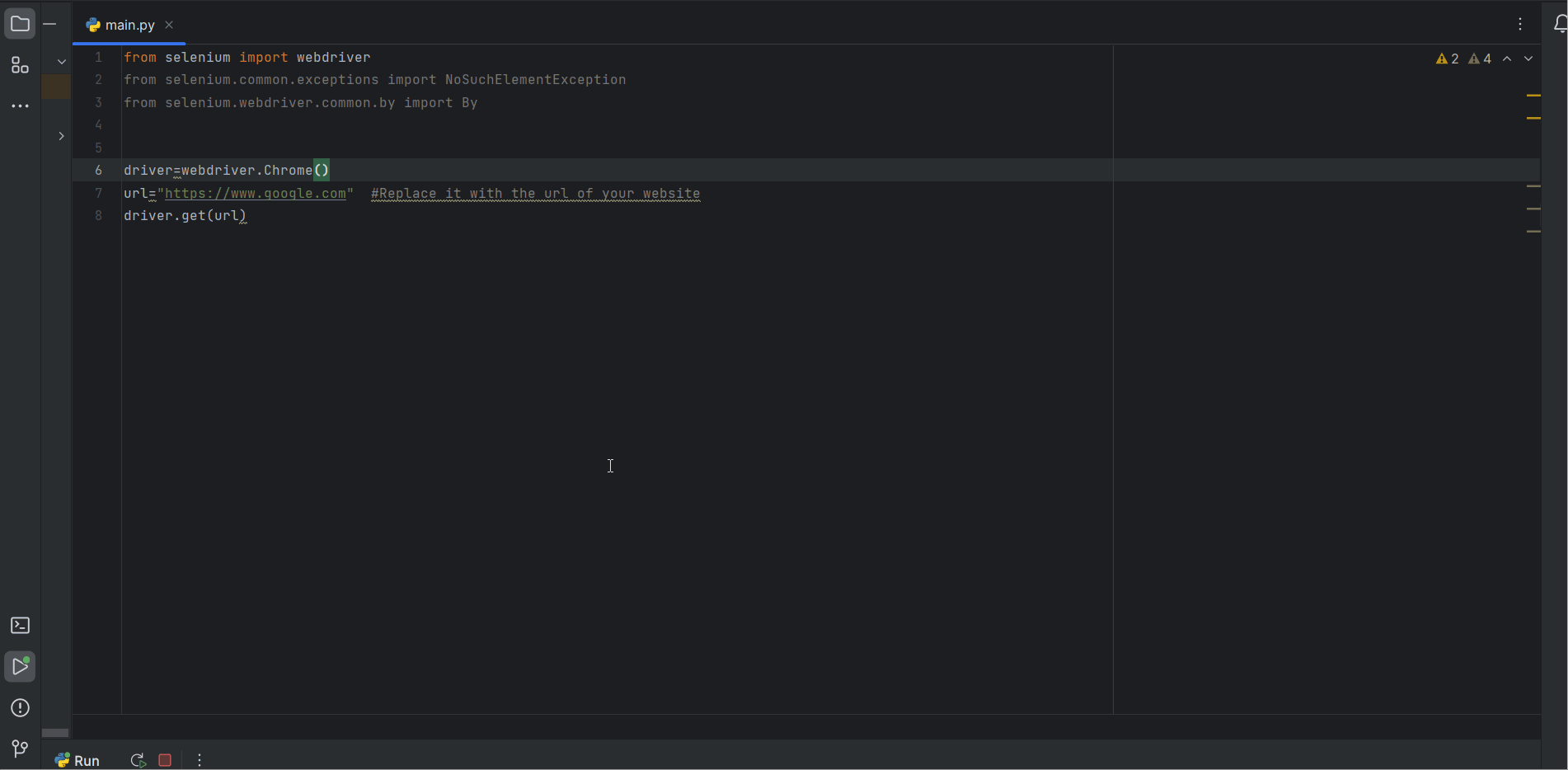
How to check if an element exists with Python Selenium?
Make sure to replace the url with the url of the website where you want to check for an element
STEP-2. Locate the element
For the script whether the element exists on a webpage or not , we first need to choose a appropriate locating strategy.
Locating Strategies- Locating Strategies refers to the method used to identify and locate an element on a webpage. Locating strategies are a crucial part for interact with an element on a webpage , they allows us to find the element we want on a web page.
Selenium provides various Locating Strategies, they are-
- ID- Id’s are the unique identifiers for an element in a HTML DOM. In this Strategy Id attribute is used to locate the element on a webpage.
- CLASS_NAME- Elements with same functionality share a common class, In this Strategy Class attribute is used to locate the element on a webpage.
- TAG_NAME- In this Strategy Tag Name of the element are used to locate an element on a webpage.
- NAME-Name attribute is used in HTML forms to handle form controls such as input fields , radio button etc. In this strategy name attribute is used to locate an element on a webpage.
- CSS SELECTOR- In this strategy we used CSS selectors to locate an element on a webpage.
- XPath- XPath stands for XML Path Language. XPath is a powerful and flexible way to locate an element on a web pages. XPath is used to target elements on a web page based on their position in a HTML document structure.
- LINK TEXT- This strategy is only used to locate anchor element(<a>) by their exact link texts.
- PARTIAL LINK TEXT- This strategy is only used to locate anchor elements (<a>) by a partial match of their link texts.
NOTE- Make sure you go through the source code in inspect element of your browser and choose the location strategy accordingly.
STEP-3. Checking if the Element Exists.
Now that we have chosen our locating strategy, we’ll use the find_element method to check if an element exists or not. If find_element finds the element, it will return the element as an instance of the Selenium WebDriver class. However, if it is unable to locate the element, it will throw a NoSuchElementException exception. Therefore, we’ll use a try and catch block to handle the possibility of the element not being found.
In this example, we are using Google. We will inspect the elements of Google and choose the Google Search Button to test. We have found a name attribute in the button’s tag and selected ‘Name’ as our locating strategy.
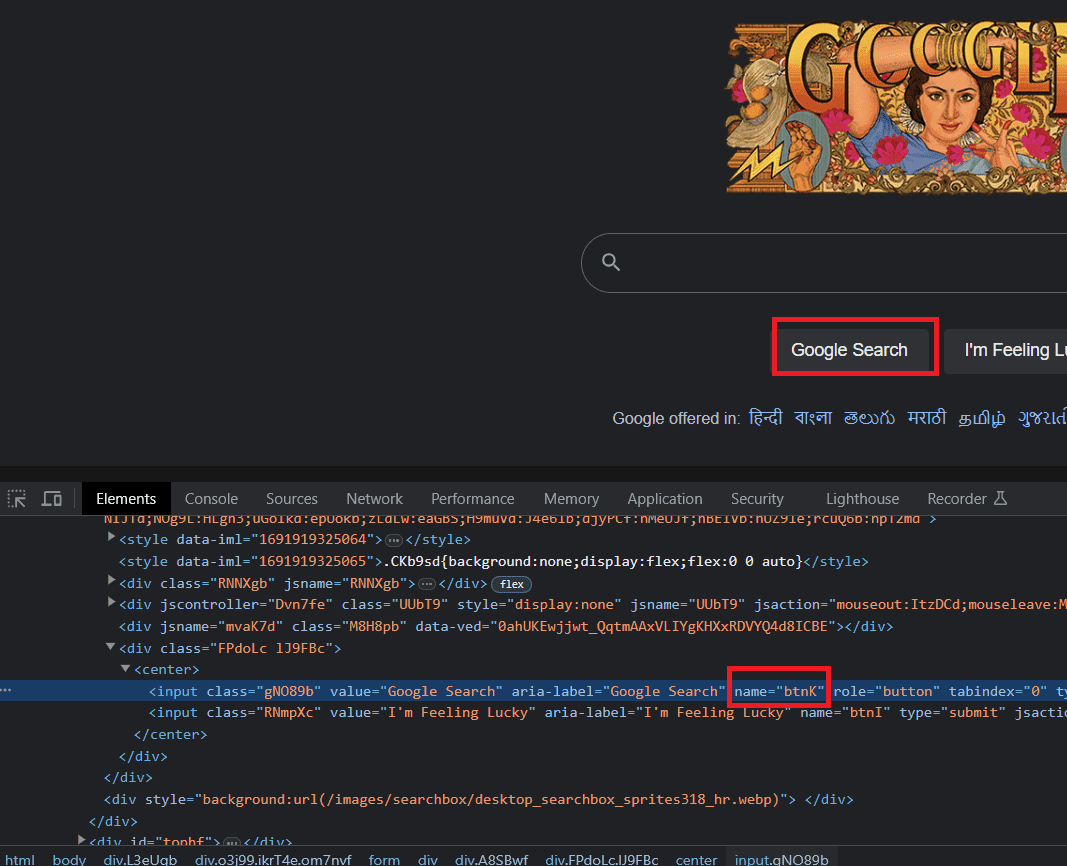
Here as we can see button element has a name attribute which has the value “btnK”.
Python
from selenium import webdriver
from selenium.common.exceptions import NoSuchElementException
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
driver.get(url)
try :
button = driver.find_element(By.NAME, "btnK" )
print ( "element exits" )
except NoSuchElementException:
print ( "element not found" )
|
OUTPUT- element exits
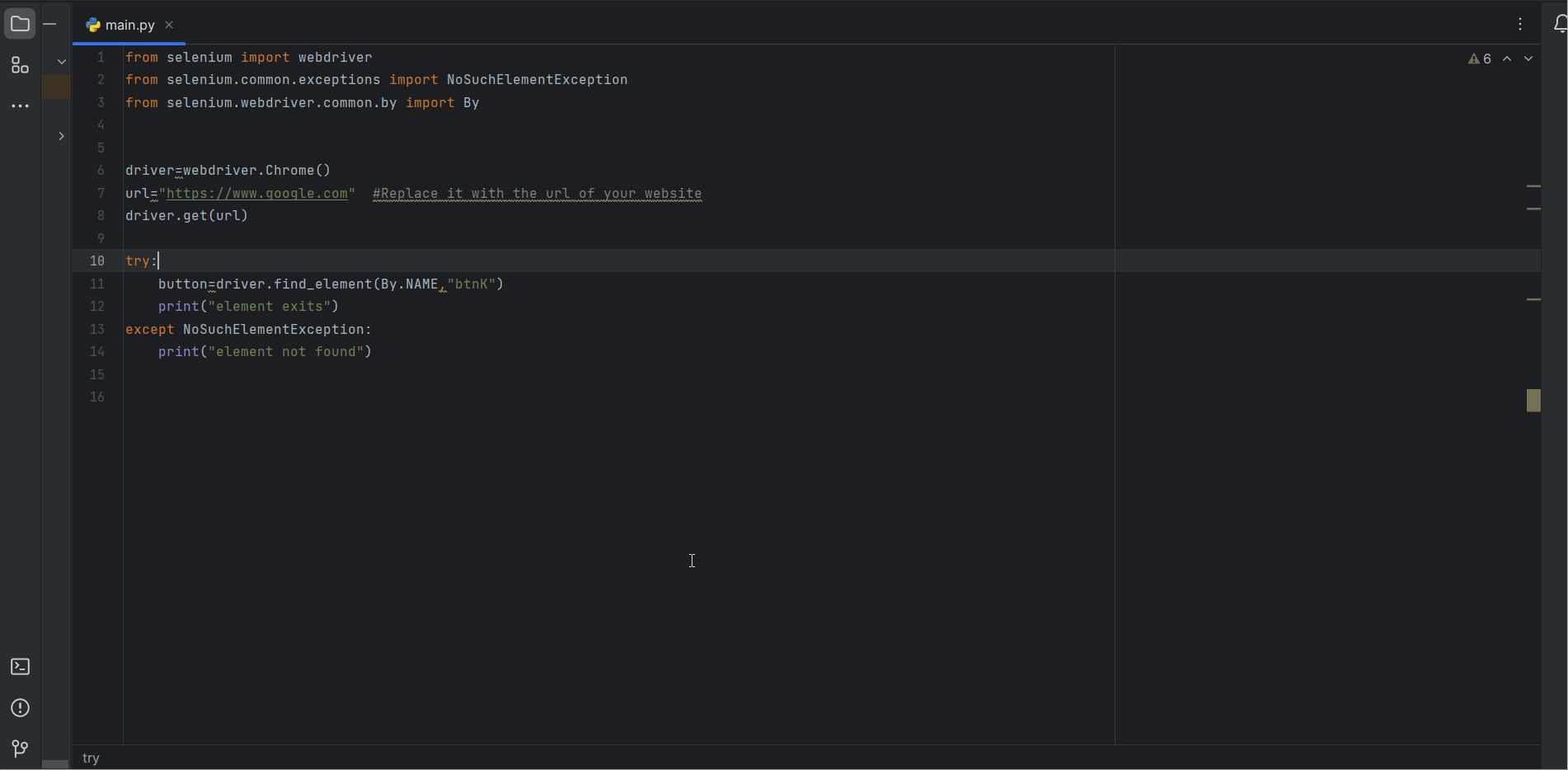
How to check if an element exists with Python Selenium?
But if we change btnK to btnk then our output will will not be the same.
Python
from selenium import webdriver
from selenium.common.exceptions import NoSuchElementException
from selenium.webdriver.common.by import By
driver = webdriver.Chrome()
driver.get(url)
try :
button = driver.find_element(By.NAME, "btnk" )
print ( "element exits" )
except NoSuchElementException:
print ( "element not found" )
|
OUTPUT- element not found
As driver was unable to found any element with name attribute of btnk .
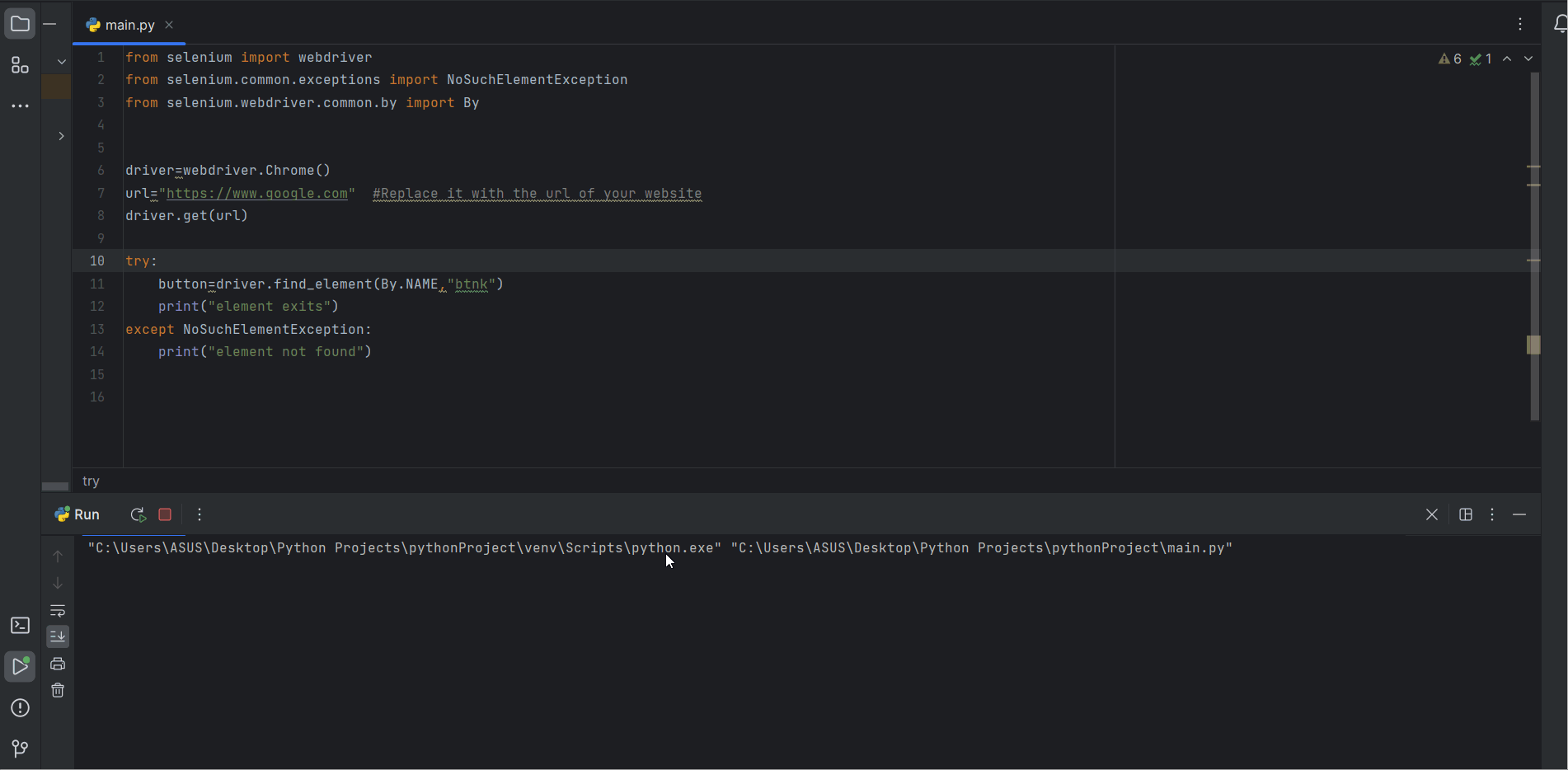
How to check if an element exists with Python Selenium?
Conclusion
Finding the presence of an element is a very crucial step specially if we are dealing with dynamic web application (A web application that changes the layout and content of the webpage according to the request made to the server). Selenium provides methods such as find_element() and find_elements() which we can use with try and except block(Exception Handling) in order to check if an element exist or not. Try and Except block is used because find_element() and find_elements() methods throws a NoSuchElementException when its usable to locate an element . Getting a good hold over these methods with surely help you in handling the dynamic nature of websites.
FAQ’s
What are Dynamic Web applications?
Dynamic web applications are the websites or web based application that generate and display content on the website
dynamically, in real time or based on user-interactions.
What is the difference between find_element and find_elements method in Selenium?
Find element is a method in Selenium used to check if an element exists on a web page or not by using the given locating
strategy and it return the first element that matches the condition. Whereas find_elements is used to return a list of all
elements that has matches the locating strategy condition.
What is a Web Driver in Selenium?
A web driver acts as a bridge between your testing code ( Python , Java or any other language) and your web
browser you want to automate. It provides a way to control the web browser programmatically.
Share your thoughts in the comments
Please Login to comment...