How to Change the Y-Axis Values from Real Numbers to Integers in Chart.js ?
Last Updated :
09 Jan, 2024
In Chart.JS, when the dataset consists of real numbers then the Y-axis scaling also consists of real numbers. This becomes complex to view the data. So we change the Y-axis values from real numbers to integers in Chart.Js using two different approaches. We have added the approaches along with the practical implementation in terms of examples and outputs.
Below are the possible approaches.
Approach 1: Using suggestedMin and suggestedMax Properties
In this approach, we are using suggestedMin and suggestedMax properties within the ticks configuration of the Y-axis. Using this property, the Y-axis values are displayed as integers, as we have given the step size of 1, ranging from the minimum value of 0 to the max value of 8.
Syntax:
const options = {
scales: {
y: {
ticks: {
stepSize: 1,
suggestedMin: 'min-int-value',
suggestedMax: 'max-int-value'
}
}
}
};
Example: Below is the implementation of the above-discussed approach.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Example 1</ title >
< script src =
</ script >
</ head >
< body >
< h1 style = "color: green;" >
GeeksforGeeks
</ h1 >
< h3 >
Approach 1: Using suggestedMin
and suggestedMax Properties
</ h3 >
< canvas id = "chart1"
width = "400"
height = "200" >
</ canvas >
< script >
const data = {
labels:
["January", "February", "March", "April", "May"],
datasets: [{
label: "GeeksforGeeks Data",
data: [5.5, 7.2, 1.8, 4.5, 2.0],
backgroundColor: 'rgba(255, 255, 25, 0.2)',
borderColor: 'rgba(75, 192, 192, 1)',
borderWidth: 1
}]
};
var options = {
scales: {
y: {
ticks: {
stepSize: 1,
suggestedMin: 0,
suggestedMax: 8
}
}
}
};
const ctx = document.
getElementById('chart1').getContext('2d');
const chart1 = new Chart(ctx, {
type: 'bar',
data: data,
options: options
});
</ script >
</ body >
</ html >
|
Output:
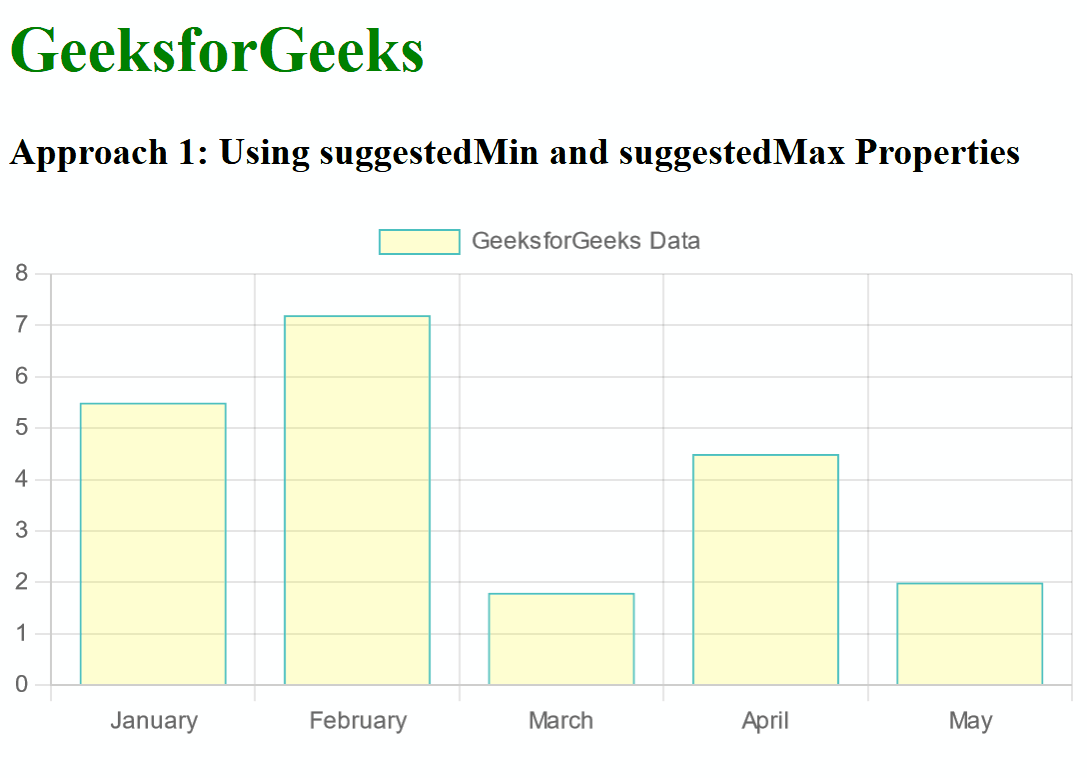
Approach 2: Using callback function for ticks
In this approach, we are using the callback function for the Y-axis ticks, which automatically performs the round operation using Math.round(value). This will convert the real number into a perfect integer value and display it on the Y-axis.
Syntax:
const options = {
scales: {
y: {
ticks: {
callback: function(value, index, values) {
// Login
}
}
}
}
};
Example: Below is the implementation of the above-discussed approach.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Example 2</ title >
< script src =
</ script >
</ head >
< body >
< h1 style = "color: green;" >
GeeksforGeeks
</ h1 >
< h3 >
Approach 2: Using callback
function for ticks
</ h3 >
< canvas id = "chart2"
width = "400"
height = "200" >
</ canvas >
< script >
var data = {
labels:
["January", "February", "March", "April", "May"],
datasets: [{
label: "GeeksforGeeks Data",
data: [10.5, 12.3, 0.8, 4.5, 2.4],
backgroundColor: 'red',
borderColor: 'red',
borderWidth: 1
}]
};
var options = {
scales: {
y: {
ticks: {
callback:
function (value, index, values) {
return Math.round(value);
}
}
}
}
};
var ctx = document.
getElementById('chart2').getContext('2d');
var chart2 = new Chart(ctx, {
type: 'line',
data: data,
options: options
});
</ script >
</ body >
</ html >
|
Output:
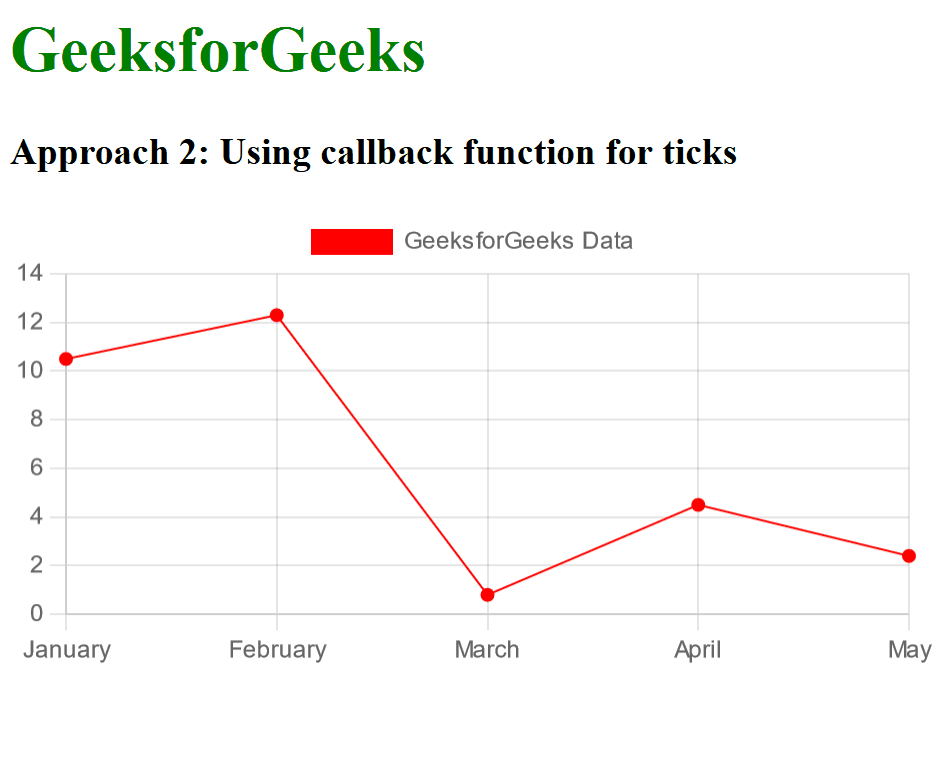
Share your thoughts in the comments
Please Login to comment...