How to Categorize a Year as a Leap or Non-Leap Using VueJS?
Last Updated :
22 Dec, 2023
In this article, we will demonstrate the way to categorize a year as a Leap or Non-Leap using VueJS. A leap year has 366 days, whereas a non-leap year has only 365 days. We can use logic to check whether a year is a Leap or not. If a year is divisible by 400 or 4 (but not by 100) it is a leap year, otherwise, it’s not.
Steps for Installation & Configuration:
Step 1: Run the following command in your command line to create a workspace for your Vue project.
npm create vue@latest
Step 2: Now, install the Vuesax package to use its styled components using the below command.
// For npm users
npm install vuesax@next
// For yarn users
yarn add vuesax@next
Step 3: After creating your Vue project move into the folder to perform different operations by visiting the folder using the below command.
cd <your-project-name>
Step to run the application:
Type the following commands in the terminal to run the application.
npm install
npm run dev
Project Structure
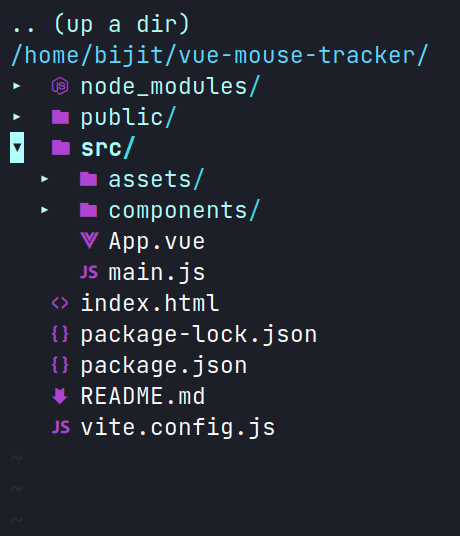
Project Structure
Example: The below example demonstrates categorization of a year as a Leap or Non-Leap using VueJS? change the App.vue code with the below code.
HTML
< template >
< div class = "grid" >
< form @ submit.prevent = "checkLeapYear" >
< vs-row >
< vs-col :sm = "size" >
< vs-input type = "number"
v-model = "year"
input-style = "border"
placeholder = "Enter the year" />
</ vs-col >
< vs-col :sm = "size" >
< vs-button color = "#2f8d46" type = "gradient" >
Check
</ vs-button >
</ vs-col >
</ vs-row >
</ form >
< div v-for = "(item, index) in leapYearArray" :key = "index" >
< p >Year: {{ item.year }}</ p >
< p >
Leap Year: {{ item.isLeapYear ? 'Yes' : 'No' }}
</ p >
< hr >< br >
</ div >
</ div >
</ template >
< script >
export default {
data() {
return {
year: null,
size: 6,
leapYearArray: []
}
},
methods: {
checkLeapYear() {
if (this.year === null) {
return;
}
const yearValue = parseInt(this.year);
let isLeapYear = false;
if ((yearValue % 4 == 0) && (yearValue % 100 != 0)) {
isLeapYear = true;
} else if (yearValue % 400 == 0) {
isLeapYear = true;
}
this.leapYearArray.push({ year: yearValue,
isLeapYear: isLeapYear });
this.year = null;
}
}
}
</ script >
|
Output:
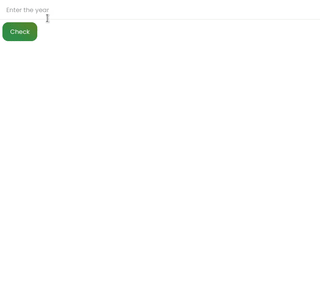
Output
Share your thoughts in the comments
Please Login to comment...