How to catch a NumPy warning like an exception in Python
Last Updated :
18 Oct, 2023
An N-dimensional array that is used in linear algebra is called NumPy. Whenever the user tries to perform some action that is impossible or tries to capture an item that doesn’t exist, NumPy usually throws an error, like it’s an exception. Similarly, in a Numpy array, when the user tries to perform some action that is impossible or tries to capture the item that doesn’t exist, such as dividing by zero, it throws an exception. In this article, we will study various ways to catch Numpy warnings like it’s an exception.
Catch a NumPy Warning like an Exception in Python
- Using errstate() function
- Using warnings library
Using errstate() Function
The Numpy context manager which is used for floating point error handling is called errstate() function. In this way, we will see how we can catch Numpy errors using errstate() function.
Syntax: with numpy.errstate(invalid=’raise’):
Example 1: In this example, we have specified a Numpy array, which we try to divide by zero and catch the error using errstate() function. It raises the error stating ‘FloatingPointError‘ which we caught and printed our message.
Python3
import numpy as np
arr = np.array([ 1 , 5 , 4 ])
with np.errstate(invalid = 'raise' ):
try :
arr / 0
except FloatingPointError:
print ( 'Error: Division by Zero' )
|
Output:

Using warnings library
The message shown to user wherever it is crucial, whether the program is running or has stopped is called warning. In this way, we will see how we can catch the numpy warning using warnings library.
Syntax: with warnings.catch_warnings():
Example 1: In this example, we have specified a numpy array, which we try to divide by zero and print the error message using warnings library. It raises the error stating ‘FloatingPointError‘ with detailed message of divide by zero encountered in divide.
Python3
import numpy as np
arr = np.array([ 1 , 5 , 4 ])
with warnings.catch_warnings():
try :
answer = arr / 0
except Warning as e:
print ( 'error found:' , e)
|
Output:
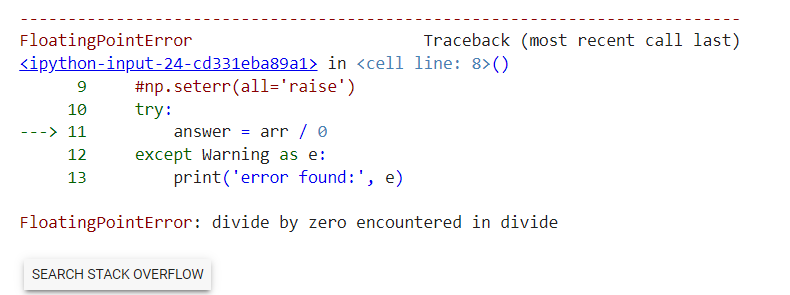
Using ‘numpy.seterr’ Function
The ‘numpy.seterr’ function to configure how NumPy handles warnings. NumPy warnings are typically emitted when there are issues related to numerical operations, data types, or other conditions that might lead to unexpected behavior. By configuring NumPy to treat warnings as exceptions, you can catch and handle them in your code. In the example above, np.seterr(all=’raise’) is used to configure NumPy to raise exceptions for all warnings. You can customize this behavior by specifying specific warning categories using the divide, over, under, etc., options of np.seterr based on your needs.
Python3
import numpy as np
np.seterr( all = 'raise' )
try :
result = np.array([ 0 , 1 ]) / 0
except Warning as e:
print (f "Caught a NumPy warning: {e}" )
except Exception as e:
print (f "Caught an exception: {e}" )
|
Output:
Conclusion
It is a matter of disappointment to see the errors or warnings while coding, but the various ways explained in this article will help you to catch the numpy warnings and resolve them or raise an exception, wherever required.
Share your thoughts in the comments
Please Login to comment...