How to bind event handlers in React?
Last Updated :
30 Jan, 2024
Binding event handlers in React is a fundamental concept that ensures interactive and responsive web applications. React, a popular JavaScript library for building user interfaces uses a declarative approach to handle events.
This article explores various methods to bind event handlers in React, highlighting their importance and usage in the context of JSX, React’s syntax extension.
Understanding Event Handling in React
In React, events are managed through event handlers, which are JavaScript functions that are called in response to user interactions like clicks, form submissions, etc. Properly binding these handlers to React elements is crucial for implementing interactivity.
We will discuss the following methods to bind event handlers in React
Prerequisites:
Steps to Create React Application :
Step 1: Create a react application by running the following command.
npx create-react-app my-app
Step 2: Navigate to the myapp directory
cd my-app
Project Structure:
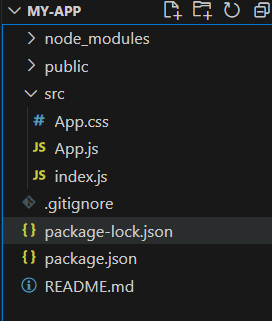
The updated dependencies in package.json file will look like.
"dependencies": {
"react": "^18.2.0",
"react-dom": "^18.2.0",
"react-scripts": "5.0.1",
"web-vitals": "^2.1.4",
}
Method 1: Using Arrow Functions in Render
Arrow functions can be used directly in the JSX to bind event handlers. This approach is simple and easy to understand.
Example:
Javascript
import React, { Component } from 'react' ;
export default class App extends Component {
handleClick() {
console.log( 'Button clicked' );
}
render() {
return <button onClick={
() => this .handleClick()}>
Click Me
</button>;
}
}
|
Output:

Explanation: When the button is clicked, “Button clicked” is logged to the console. However, using an arrow function in render method creates a new function each time the component renders, which might affect performance if the component renders often.
Method 2: Binding in the Constructor
This method involves binding the event handler in the component’s constructor. It’s a recommended approach for performance reasons.
Example:
Javascript
import React, { Component } from 'react' ;
export default class App extends Component {
constructor(props) {
super (props);
this .handleClick = this .handleClick.bind( this );
}
handleClick() {
console.log( 'Button clicked' );
}
render() {
return <button onClick={ this .handleClick}>
Click Me
</button>;
}
}
|
Output:

Method 3: Class Properties as Arrow Functions
Using class fields with arrow functions auto-binds them to the instance of the class. This is a newer and increasingly popular syntax.
Example:
Javascript
import React, { Component } from 'react' ;
export default class App extends Component {
handleClick = () => {
console.log( 'Button clicked' );
}
render() {
return <button onClick={ this .handleClick}>
Click Me
</button>;
}
}
|
Output:

Conclusion:
Binding event handlers in React is a key aspect of creating dynamic and interactive web applications. Each method of binding has its own benifits. Arrow functions in render offer simplicity, constructor binding can be used where performance is considered important, and class properties as arrow functions provide a balance between cleaner code and performance. Understanding these methods is essential for any developer working with React to ensure efficient and responsive applications.
Share your thoughts in the comments
Please Login to comment...