How to Allow Only Special Characters and Spaces in Text Field Using jQuery ?
Last Updated :
10 Jan, 2024
We are going to create an input box that only contains the special characters and the space as the input. It will show an error if any other character is entered. We will use regular expressions that consist of only special symbols and spaces with different events in jQuery.
In this approach, we will use the regular expression with the input event that checks the correctness of the entered input at the same time and write the respective text for the same.
Syntax:
$('input_selector').on('input', function(){});
Example: The below code example will help you understand the use of the regex with input event to check for the correct input.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< title >
How to allow only special characters and
spaces in text field using jQuery?
</ title >
< style >
.container{
text-align: center;
}
</ style >
</ head >
< body >
< div class = "container" >
< h1 style = "color: green" >
GeeksforGeeks
</ h1 >
< h2 >
The below input will take only the < br />
special characters and spaces as input.
</ h2 >
< input type = "text" id = "inp" >
< p class = "result" ></ p >
</ div >
< script src =
integrity =
"sha512-v2CJ7UaYy4JwqLDIrZUI/4hqeoQieOmAZNXBeQyjo21dadnwR+8ZaIJVT8EE2iyI61OV8e6M8PP2/4hpQINQ/g=="
crossorigin = "anonymous" referrerpolicy = "no-referrer" >
</ script >
< script >
$(document).ready(() => {
$('#inp').on('input', function (e) {
const enteredVal = $(this).val();
let result = $('.result');
let regex = /^[\s!@#$%^&*()_+{}\[\]:;<>,.?~\\/-]+$/g;
if (regex.test(enteredVal)) {
result.html(
`< b style = "color: green" >
The entered value is correct!!
</ b >`)
}
else {
result.html(
`< b style = "color: red" >
The entered value is incorrect!!
</ b >`)
}
});
});
</ script >
</ body >
</ html >
|
Output:
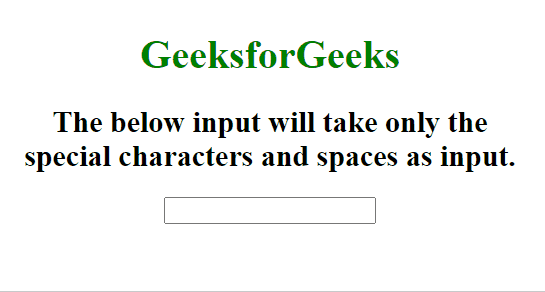
By comparing the ASCII codes of the pressed keys
In this method, we will check the ASCII value of the currently pressed key and compare it with the ASCII values of the special symbol and the space. It will compare the entered key once the key is pressed.
Syntax:
$('input_selector').on('keypress', function(e){});
Example: The below code example will help you understand and implement this approach practically.
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport" content =
"width=device-width, initial-scale=1.0" >
< title >
How to allow only special characters
and spaces in text field using jQuery?
</ title >
< style >
.container{
text-align: center;
}
</ style >
</ head >
< body >
< div class = "container" >
< h1 style = "color: green" >
GeeksforGeeks
</ h1 >
< h2 >
The below input will take only the < br />
special characters and spaces as input.
</ h2 >
< input type = "text" id = "inp" >
< p class = "result" ></ p >
</ div >
< script src =
integrity =
"sha512-v2CJ7UaYy4JwqLDIrZUI/4hqeoQieOmAZNXBeQyjo21dadnwR+8ZaIJVT8EE2iyI61OV8e6M8PP2/4hpQINQ/g=="
crossorigin = "anonymous"
referrerpolicy = "no-referrer" >
</ script >
< script >
$(document).ready(() => {
$(document).on('keypress', '#inp', function (e) {
let result = $('.result');
const pressedKey = e.key;
let pressedKeyCode = e.charCode;
if ((pressedKeyCode >= 32 && pressedKeyCode <= 47) ||
(pressedKeyCode >= 58 && pressedKeyCode <= 64) ||
(pressedKeyCode >= 91 && pressedKeyCode <= 96) ||
(pressedKeyCode >= 123 && pressedKeyCode <= 126))
{
e.preventDefault();
result.html(`
< b style = "color: green" >
The pressed key
< em >
'${pressedKey}'
</ em >
is a Valid input!!
</ b >`)
}
else {
e.preventDefault();
result.html(`
< b style = "color: red" >
The pressed key
< em >
'${pressedKey}'
</ em >
is not a Valid input!!
</ b >`)
}
});
});
</ script >
</ body >
</ html >
|
Output:
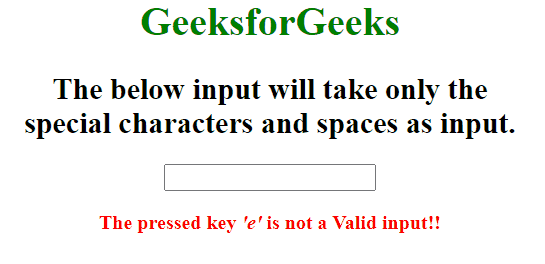
Share your thoughts in the comments
Please Login to comment...