How to Add Two Values in Bootstrap Range Slider ?
Last Updated :
16 Apr, 2024
The range slider is used to allow users to select values within a defined range. Sliders offer a user-friendly interface to interact, users can select values by sliding a handle along a track. We will focus on the steps that how to add two values in the Bootstrap 5 range slider.
Approach
- First, create a html structure and add the CDN links. Set the background color of the page to light using the “bg-light” class in the <body> tag.
- In the ‘body’ section, create a main container using the ‘container‘ class, which serves as the main holding point for all elements.
- And inside the container, create an <div> element with classes “text-center”, “bg-success”, “p-5”, and “rounded” to style the content area.
- After that include two <input> elements of type range with classes “form-range”, “range-primary”, and “range-info” for styling the range sliders. You can set their min, max, and value attributes as you need.
- Write JavaScript code to update the displayed values whenever the slider values change.
Example: This example shows the implementation of adding two values in the Bootstrap 5 range slider.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<title>Dynamic Tabs Example</title>
<link rel="stylesheet" href=
"https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha1/dist/css/bootstrap.min.css">
</head>
<body class="bg-light">
<div class="container d-flex
justify-content-center
align-items-center vh-100">
<div class="text-center bg-success
p-5 rounded">
<h1 class="text-light">
Adding two values in Bootstrap 5 Range Slider
</h1>
<div class="mb-3">
<input type="range"
class="form-range range-primary"
id="rangeSlider1" min="0"
max="1000" value="0">
<input type="range"
class="form-range mt-3 range-info"
id="rangeSlider2"
min="0" max="1000" value="0">
</div>
<div class="text-center">
<span id="sliderValue1" class="text-light">
0
</span>
<span class="text-light">
+
</span>
<span id="sliderValue2" class="text-light">
0
</span>
<span class="text-light">
=
</span>
<span id="sum" class="text-light">
0
</span>
</div>
</div>
</div>
<script src=
"https://cdn.jsdelivr.net/npm/bootstrap@5.3.0-alpha1/dist/js/bootstrap.bundle.min.js">
</script>
<script>
let range_slide1 = document.getElementById('rangeSlider1');
let range_slide2 = document.getElementById('rangeSlider2');
let sliderValue1 = document.getElementById("sliderValue1");
let sliderValue2 = document.getElementById("sliderValue2");
let sumDisplay = document.getElementById("sum");
function updateSum() {
let val1 = parseInt(range_slide1.value);
let val2 = parseInt(range_slide2.value);
let sum = val1 + val2;
sliderValue1.textContent = val1;
sliderValue2.textContent = val2;
sumDisplay.textContent = sum;
};
range_slide1.addEventListener('input', function () {
updateSum();
});
range_slide2.addEventListener('input', function () {
updateSum();
});
window.addEventListener('load', updateSum);
</script>
</body>
</html>
Output:
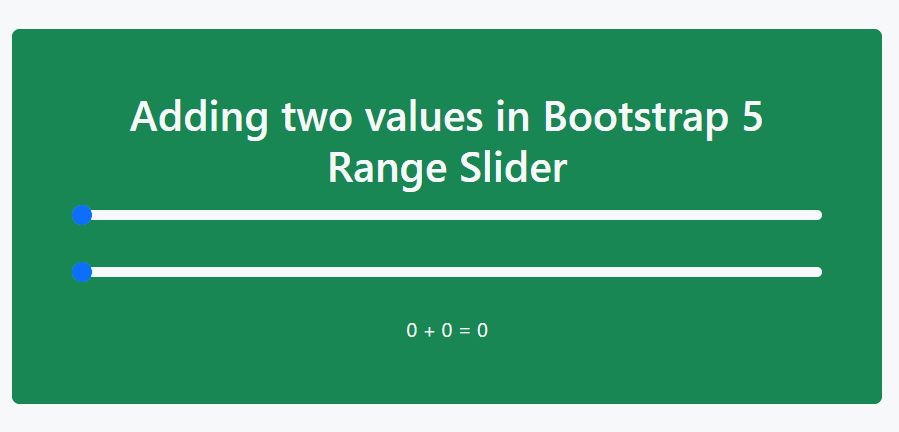
Output
Share your thoughts in the comments
Please Login to comment...