How to Add Items in ComboBox in C#?
Last Updated :
27 Jun, 2019
In Windows forms, ComboBox provides two different features in a single control, it means ComboBox works as both TextBox and ListBox. In ComboBox, only one item is displayed at a time and the rest of the items are present in the drop-down menu. You are allowed to a list or collection of elements in your ComboBox by using Items Property. You can set this property using two different methods:
1. Design-Time: It is the easiest method to add the items in the ComboBox control using the following steps:
2. Run-Time: It is a little bit trickier than the above method. In this method, you can add the list of elements in the ComboBox programmatically with the help of given syntax:
public System.Windows.Forms.ComboBox.ObjectCollection Items { get; }
Here, the ComboBox.ObjectCollection indicates the elements present in the ComboBox. Following steps are used to add the elements in the ComboBox:
- Step 1: Create a combobox using the ComboBox() constructor is provided by the ComboBox class.
// Creating ComboBox using ComboBox class
ComboBox mybox = new ComboBox();
- Step 2: After creating ComboBox, add the elements in the ComboBox.
// Adding elements in the combobox
mybox.Items.Add(230);
mybox.Items.Add(231);
mybox.Items.Add(232);
mybox.Items.Add(233);
mybox.Items.Add(234);;
- Step 3: And last add this combobox control to form using Add() method.
// Add this ComboBox to form
this.Controls.Add(mybox);
Example:
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Data;
using System.Drawing;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Windows.Forms;
namespace WindowsFormsApp11 {
public partial class Form1 : Form {
public Form1()
{
InitializeComponent();
}
private void Form1_Load( object sender, EventArgs e)
{
Label l = new Label();
l.Location = new Point(222, 80);
l.Size = new Size(99, 18);
l.Text = "Select Your id" ;
this .Controls.Add(l);
ComboBox mybox = new ComboBox();
mybox.Location = new Point(327, 77);
mybox.Size = new Size(216, 26);
mybox.Name = "My_Cobo_Box" ;
mybox.Items.Add(230);
mybox.Items.Add(231);
mybox.Items.Add(232);
mybox.Items.Add(233);
mybox.Items.Add(234);
this .Controls.Add(mybox);
}
}
}
|
Output:
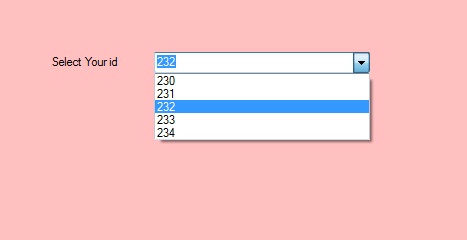
Share your thoughts in the comments
Please Login to comment...