How to Add Commas Between a List of Items Dynamically with CSS ?
Last Updated :
30 Mar, 2023
In this article, we are going to create a list that is separated with commas using CSS.
Suppose you have given the name of the students and the task is to show them on the frontend in a list view, and sometimes you have to remove some of the students who did not get the required marks. But one thing we have to keep in mind is we have to display no comma after the last element initially or after removing the items from the list.
Approach: We use JavaScript to make the list display dynamically and getting more control over the CSS. We use the general sibling selector to get rid of the comma at the end. Design the list using HTML ul using class name as students and then create li elements with class name student show.
Apply the CSS to the given list. Apply list-style : none to remove the default bullets from the list and then set the display: flex to make the list horizontal and remove the padding by setting it to zero.
Now apply the general sibling selector on student class to select all the .student elements after the first .student element and then by using the :: before pseudo-element assigning a space followed by a comma.
HTML
< ul class = "students" >
< li class = "student show" >John</ li >
< li class = "student show" >Peter</ li >
< li class = "student show" >Mark</ li >
< li class = "student show" >Bill</ li >
< li class = "student show" >Jack</ li >
</ ul >
|
CSS
.students{
display : flex;
list-style : none ;
padding : 0 ;
}
.student ~ .student::before{
content : ", " ;
}
|
Output:
John, Peter, Mark, Bill, Jack
Add some JavaScript code to remove items from the list. Add some buttons that trigger the on-demand JavaScript codes.
Add logic to add/remove items from the list. We have created two functions, the first one removes the items at a specific position and the second one adds elements at the specific position.
HTML design:
HTML
< ul class = "students" >
< li class = "student show" >John</ li >
< li class = "student show" >Peter</ li >
< li class = "student show" >Mark</ li >
< li class = "student show" >Bill</ li >
< li class = "student show" >Jack</ li >
</ ul >
< button onclick = "removeItem('first')" >Remove first</ button >
< button onclick = "removeItem('last')" >Remove last</ button >
< button onclick = "removeItem('random')" >Remove Random</ button >
< br >< br >
< button onclick = "addItem('first')" >Add first</ button >
< button onclick = "addItem('last')" >Add last</ button >
< button onclick = "addItem('random')" >Add Random</ button >
|
Javascript
let student = document.querySelectorAll( ".student" )
function removeItem(position) {
if (position == "first" ) {
student[0].remove()
}
if (position == "last" ) {
student[student.length - 1].remove()
}
if (position == "random" ) {
student[(Math.floor(
Math.random() * student.length))].remove()
}
}
let list = document.querySelector( ".students" )
function addItem(position) {
let item = document.createElement( "li" )
item.innerText = "Added Item"
item.className = "student"
if (position == "first" ) {
list.insertBefore(item, list.childNodes[0])
}
if (position == "last" ) {
list.insertBefore(item,
list.childNodes[list.children.length - 1])
}
if (position == "random" ) {
list.insertBefore
(item, list.childNodes[(Math.floor(
Math.random() * list.children.length))])
}
}
|
Output: Click here to see live code output
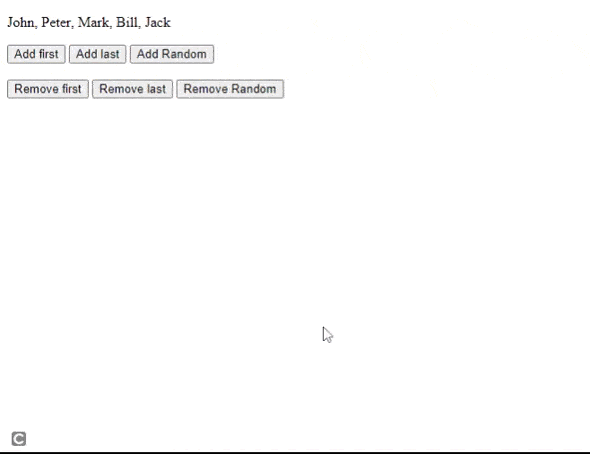
Share your thoughts in the comments
Please Login to comment...