How to Access Array of Objects in JavaScript ?
Last Updated :
30 Nov, 2023
In this article, we are going to learn how we Access an Array of Objects in JavaScript. An array of objects in JavasScript is a collection of elements that individually hold a number of properties and values.
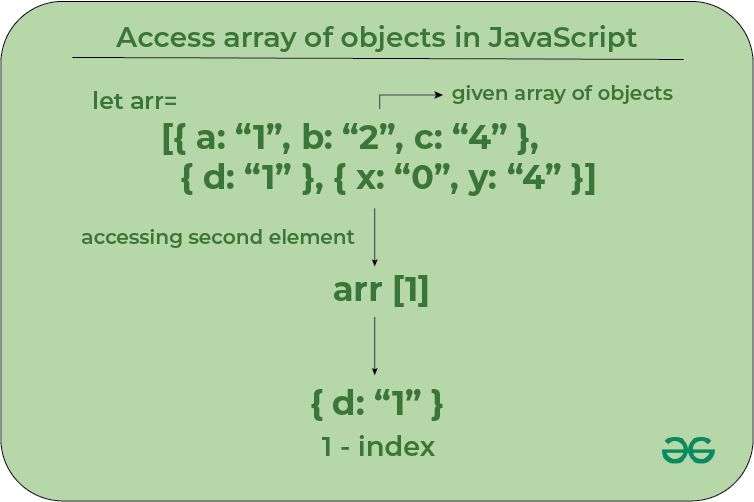
How to Access an Array of Objects in JavaScript?
The approaches to access the array of objects in JavaScript are:
Using the Brackets notation
Using bracket notation and the object’s desired index, you can access an object in an array. In type, the whole object is accessed and only one of the properties of a specific object can’t be accessed.
Syntax:
arrayName[arrayIndex]
Example: The code below demonstrates how we can use the brackets notation to access the elements of the array of objects.
Javascript
let objArr = [
{
name: 'john' ,
age: 12,
gender: 'male'
},
{
name: 'jane' ,
age: 15,
gender: 'female'
},
{
name: 'julie' ,
age: 20,
gender: 'trans'
}
];
console.log( "First Object in the Array using the [] notation:" )
console.log(objArr[0]);
|
Output
First Object in the Array using the [] notation:
{ name: 'john', age: 12, gender: 'male' }
Using the DOT notation
This method can’t be used directly to access the array of objects but we have to use it together with the bracket notation. With this method, we can access any specific property of a particular object in the array of objects but not the whole object.
Syntax:
arrayName[arrayIndex].propertyName
Example: The code below demonstrates how we can use the DOT notation along with the brackets notation to access the elements of the array of objects:
Javascript
let objArr = [
{
name: 'john' ,
age: 12,
gender: 'male'
},
{
name: 'jane' ,
age: 15,
gender: 'female'
},
{
name: 'julie' ,
age: 20,
gender: 'trans'
}
];
console.log( "Accessing the value using the [] and DOT notations:" )
console.log(objArr[1].gender);
|
Output
Accessing the value using the [] and DOT notations:
female
The for..in loop is a type of loop that is used in JavaScript and this can be used pretty well in accessing elements of the array. By this method, you can access both the whole elements and the properties inside the objects.
Syntax:
for (var key in arrayName) {
console.log(arrayName[key].name);
//Or we can access the specific properties using
console.log(arrayName[arrayIndex].propertyName);
}
Example: The code below demonstrates how we can use the for..in the loop to access the elements of the array of objects:
Javascript
let objArr = [
{
name: 'john' ,
age: 12,
gender: 'male'
},
{
name: 'jane' ,
age: 15,
gender: 'female'
},
{
name: 'julie' ,
age: 20,
gender: 'trans'
}
];
console.log( "Accessing the values using the for..in loop:" )
for (let key in objArr) {
console.log(objArr[key]);
}
|
Output
Accessing the values using the for..in loop:
{ name: 'john', age: 12, gender: 'male' }
{ name: 'jane', age: 15, gender: 'female' }
{ name: 'julie', age: 20, gender: 'trans' }
The forEach loop allows you to loop through an array of objects and access each one individually. By this method too, you can access both the whole elements and the properties inside the objects.
Syntax:
arrayName.forEach(function(item) {
console.log(item);
});
Example: The code below demonstrates how we can use the forEach loop to access the elements of the array of objects.
Javascript
let objArr = [
{
name: 'john' ,
age: 12,
gender: 'male'
},
{
name: 'jane' ,
age: 15,
gender: 'female'
},
{
name: 'julie' ,
age: 20,
gender: 'trans'
}
];
console.log( "Accessing the arrayusing the forEach loop:" );
objArr.forEach( function (item) {
console.log(item);
});
|
Output
Accessing the arrayusing the forEach loop:
{ name: 'john', age: 12, gender: 'male' }
{ name: 'jane', age: 15, gender: 'female' }
{ name: 'julie', age: 20, gender: 'trans' }
The map() function in JavaScript allows you to access an array of objects and change it into another array. The map() function calls a supplied function on each element of the original array, producing the results in a new array.
Syntax:
arrayName.map((item) => {
console.log(item);
});
Example: The code below demonstrates how we can use the forEach loop to access the elements of the array of objects.
Javascript
let objArr = [
{
name: 'john' ,
age: 12,
gender: 'male'
},
{
name: 'jane' ,
age: 15,
gender: 'female'
},
{
name: 'julie' ,
age: 20,
gender: 'trans'
}
];
console.log( "Accessing the Array using the forEach loop:" )
objArr.map((item) => {
console.log(item);
});
|
Output
Accessing the Array using the forEach loop:
{ name: 'john', age: 12, gender: 'male' }
{ name: 'jane', age: 15, gender: 'female' }
{ name: 'julie', age: 20, gender: 'trans' }
You may access an array of items and create a new array in JavaScript by using the map() function. The map() function calls a given function on each element of the original array, creating a new array with the results.
Syntax:
arrayName.filter(function(item) {
console.log(item);
});
Example: The code below demonstrates how we can use the filter function to access the elements of the array of objects. It also shows how we can get a specific object from the array of objects using the clause in the filter function:
Javascript
let objArr = [
{
name: 'john' ,
age: 12,
gender: 'male'
},
{
name: 'jane' ,
age: 15,
gender: 'female'
},
{
name: 'julie' ,
age: 20,
gender: 'trans'
}
];
console.log( "Accessing the Array using the forEach loop:" )
objArr.filter( function (item) {
console.log(item);
});
console.log( "Using the Filer method to acces value" )
const search = objArr.filter( function (item) {
return item.name === 'jane' ;
});
console.log(search);
|
Output
Accessing the Array using the forEach loop:
{ name: 'john', age: 12, gender: 'male' }
{ name: 'jane', age: 15, gender: 'female' }
{ name: 'julie', age: 20, gender: 'trans' }
Using the Filer method to ...
Share your thoughts in the comments
Please Login to comment...