Function overloading in programming allows multiple functions to have the same name but with different parameters. It lets a function perform different tasks based on the input parameters. This increases the flexibility and readability of the code. Languages like C++, Java, and C# have function overloading. A programmer can provide different implementations for one function name. The number and types of parameters differentiate them. This enhances code readability and usability. Conceptually similar tasks are handled by function variants.
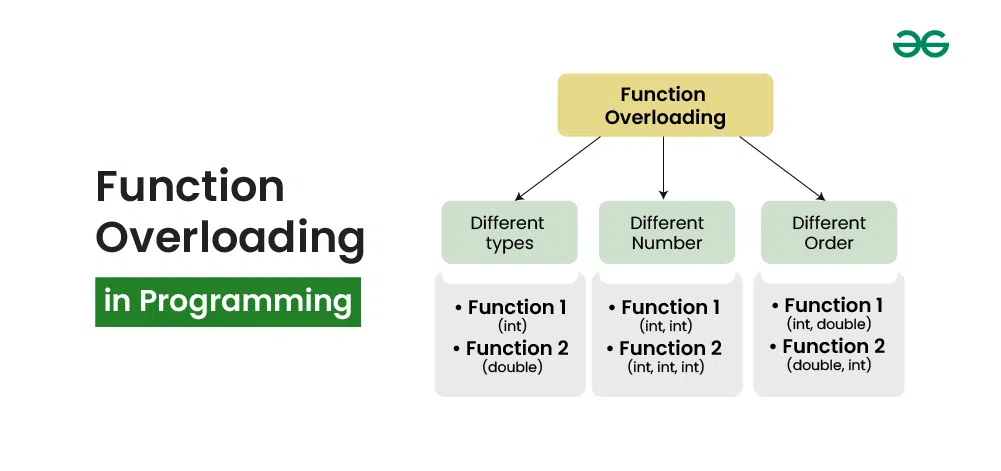
Function Overloading in Programming
What is Function Overloading?
Function overloading is a feature of object-oriented programming where two or more functions can have the same name but different parameters. This allows one function to perform different tasks depending on the context of the call. The functions must differ either by the numbers or types of their parameters. It’s a form of static polymorphism, and the specific function to call is resolved at compile time. This increases the readability and flexibility of the program.
How Function Overloading Works?
The number of parameters:
Functions can be overloaded based on differing numbers of parameters.
void display(int i);
void display(int i, int j);
Type of parameters:
Functions can also be overloaded with the same number of parameters, as long as the types are different.
void display(int i);
void display(double d);
Order of parameters:
If the number and types of parameters are the same, their order can also be a factor in overloading.
void display(int i, double d);
void display(double d, int i);
Implementation:
C++
#include<iostream>
using namespace std;
void print(int i) {
cout << "Printing int: " << i << endl;
}
void print(double f) {
cout << "Printing float: " << f << endl;
}
void print(int i, double f) {
cout << "Printing int and float: " << i << ',' << f << endl;
}
void print(double f, int i) {
cout << "Printing float and int: " << f << ',' << i << endl;
}
int main() {
print(10);
print(10.10);
print(10, 10.10);
print(10.10, 10);
return 0;
}
Java
public class Main {
static void print(int i) {
System.out.println("Printing int: " + i);
}
static void print(double f) {
System.out.println("Printing double: " + f);
}
static void print(int i, double f) {
System.out.println("Printing int and double: " + i + ", " + f);
}
static void print(double f, int i) {
System.out.println("Printing double and int: " + f + ", " + i);
}
public static void main(String[] args) {
print(10);
print(10.10);
print(10, 10.10);
print(10.10, 10);
}
}
OutputPrinting int: 10
Printing float: 10.1
Printing int and float: 10,10.1
Constructors and Destructors Overloading:
Object-oriented programming allows objects to have multiple ways of creation. This is known as overloading constructors. It lets classes initialize in diverse ways. Destructors, however, cannot be overloaded – one destructor handles object cleanup when its life ends.
Constructor Overloading:
Constructor overloading is a concept in programming where a class can have multiple constructors, each with a different parameter list. This allows objects of the class to be created in various ways, depending on the arguments provided during object creation.
With constructor overloading, a single class can initialize objects differently depending on the situation or inputs provided. Complex classes benefit most, where objects may require many initialization approaches.
Advantages of Constructors Overloading :
- Flexible Initialization: Varied constructors enable flexible object creation, tailored to specific needs.
- Better Code Quality: Logical overloads can enhance readability and maintainability for easier understanding.
- Use of Default and Copy Constructors: Default constructors (no parameters) and copy constructors (one parameter of the same class type) are special forms of constructor overloading.
C++
#include <iostream>
using namespace std;
class Box {
public:
Box() {
// default constructor
cout << "Default Constructor called!" << endl;
length = 5; // default value
}
Box(double l) {
// parameterized constructor
cout << "Parameterized Constructor called!" << endl;
length = l;
}
Box(const Box &obj) {
// copy constructor
cout << "Copy Constructor called!" << endl;
length = obj.length;
}
double getLength() {
return length;
}
private:
double length;
};
int main() {
Box Box1; // invokes default constructor
Box Box2(10.0); // invokes parameterized constructor
Box Box3(Box2); // invokes copy constructor
cout << "Length of Box1 : " << Box1.getLength() << endl;
cout << "Length of Box2 : " << Box2.getLength() << endl;
cout << "Length of Box3 : " << Box3.getLength() << endl;
return 0;
}
Java
public class Box {
private double length;
public Box() {
// Default constructor
System.out.println("Default Constructor called!");
this.length = 5; // Default value
}
public Box(double l) {
// Parameterized constructor
System.out.println("Parameterized Constructor called!");
this.length = l;
}
public Box(Box obj) {
// Copy constructor
System.out.println("Copy Constructor called!");
this.length = obj.length;
}
public double getLength() {
return this.length;
}
public static void main(String[] args) {
Box box1 = new Box(); // Invokes default constructor
Box box2 = new Box(10.0); // Invokes parameterized constructor
Box box3 = new Box(box2); // Invokes copy constructor
System.out.println("Length of Box1 : " + box1.getLength());
System.out.println("Length of Box2 : " + box2.getLength());
System.out.println("Length of Box3 : " + box3.getLength());
}
}
OutputDefault Constructor called!
Parameterized Constructor called!
Copy Constructor called!
Length of Box1 : 5
Length of Box2 : 10
Length of Box3 : 10
Destructor Overloading:
Destructor Overloading is not possible in programming. A destructor is a special function that is automatically called when an object goes out of scope. It doesn’t take any arguments and doesn’t return anything, so it can’t be overloaded with different parameters.
Destructors in C++ have clearly defined rules. They cannot take different forms – only one destructor exists per class. Additionally, destructors are not given inputs, nor do they output results. A destructor’s name begins with a tilde (~), followed by the class’s title. Its purpose is to properly free up any resources assigned to an object before that object ceases existing.
Operator Overloading:
The operator overloading is a feature in many programming languages where the existing operators are defined and used such that on of the operands will be either of a user defined class, struct, or enumeration. This is the usual feature of languages in the family of C++, Python and C# where operators do more than just their predefined interpretation.
Operator overloading is a compile time polymorphism.
Example:
int a;
float b,sum;
sum=a+b;
Here, variables “a” and “b” are of type “int” and “float”, which are built in data types. Hence the addition operator ‘+’ can easily add the contents of “a” and “b”. This is because, the addition operator “+” is predefined to add variables of built in data type only.
Function Overloading in C:
Here are the implementation of the function overloading in c language:
C
#include <stdio.h>
void add_int(int a, int b) {
printf("sum = %d\n", a + b);
}
void add_double(double a, double b) {
printf("sum = %f\n", a + b);
}
int main() {
add_int(10, 2);
add_double(5.3, 6.2);
return 0;
}
Outputsum = 12
sum = 11.500000
Function Overloading in C++:
Here are the implementation of the function overloading in c++ language:
C++
#include <iostream>
using namespace std;
void add(int a, int b)
{
cout << "sum = " << (a + b);
}
void add(double a, double b)
{
cout << endl << "sum = " << (a + b);
}
// Driver code
int main()
{
add(10, 2);
add(5.3, 6.2);
return 0;
}
Outputsum = 12
sum = 11.5
Function Overloading in Java:
Here are the implementation of the function overloading in java language:
Java
public class Main {
static void add(int a, int b) {
System.out.println("sum = " + (a + b));
}
static void add(double a, double b) {
System.out.println("sum = " + (a + b));
}
public static void main(String[] args) {
add(10, 2);
add(5.3, 6.2);
}
}
Outputsum = 12
sum = 11.5
Function Overloading in Python:
Here are the implementation of the function overloading in python language:
Python3
def add(a, b):
print("sum =", a + b)
def main():
add(10, 2)
add(5.3, 6.2)
if __name__ == "__main__":
main()
Outputsum = 12
sum = 11.5
Advantages of Function Overloading:
- Enhanced Readability: The same operations are named the same, but with different parameters with the standard being the names of the functions that return results.
- Simplified Usage: Modularization provides abstraction that is useful for many functions around a single name, instead of memorizing the multiple names.
- Consistency: Preserves constant nosing conventions all over connected operations, consequently, whole system remains intuitive and predictable to read.
- Compile-Time Polymorphism: Selections made about to call what variants function at compile time speed up executional efficiency because there is no communication with runtime during decision making.
Disadvantages of Function Overloading:
- Increased Complexity: The code becomes more complicated, because the functions in different groups contributes clutter. Sometimes new developers may find it hard to grasp quickly, which one of the functions will be called, without having even looked at the function.
- Ambiguity in Function Selection: However, if not taken care of and overloading functions then the return ambiguity will arise where the compiler is not capable to select which function to call, or the compiler will select the function that is not matching the provided function arguments. This generally occurs when languages have default type conversions.
- Compiler Dependency: Variation of compiler behavior and rule for function overloading exists, it is common to see the code act ‘unpredictably’ when porting it from one compiler to the other.
- Debugging Difficulties: Putting up with problems linked to function overloading is rather hard, since the errors you get may fail to show which of the functions it was that caused it.
- Overhead of Type Conversions: When type conversions are required for every function call, it might be a source of performance overhead, especially in cases when it is such conversions which are implicit and are immediately overlooked by the developer.
Use cases of Function Overloading:
1. Mathematical Functions: By means of overloading one can develop a function that does not display any problems when executing the portion of the code that deals with different parameters (integers, floats or doubles).
int max(int x, int y);
double max(double x, double y);
2. Constructors and Initialization: Constructors can be overloaded to implement object initialization in different scenarios, eg to provide more initialization options or to sort out side effects of some initial values.
class Rectangle {
public:
Rectangle();
Rectangle(double length, double breadth);
Rectangle(double side); // square
};
3. Print and Display Functions: Extra mechanisms that are already in a print() or display() functions have assisted in processing of more than just one data format and type, which in turn improves usability and functionality.
void print(string s);
void print(int n);
void print(double d);
4. File Handling: This can enable file operations comprising of different file access strategies on a single interface point.
void openFile(string filename);
void openFile(string filename, bool readOnly);
Conclusion:
Function overloading is a powerful feature in programming that enhances code readability and maintainability. It allows the same operation to be performed on different types of data by defining multiple functions with the same name but different parameters. This makes the code more flexible and reusable
Share your thoughts in the comments
Please Login to comment...