Flask – Language Detector App
Last Updated :
28 Mar, 2023
In this article, we will see how we can detect the language of an entered text in Python using Flask. To detect language using Python we need to install an external library called “langdetect” and “pycountry“. Let’s see how to implement Flask Language Detector App, before implementation we will try to understand the basic modules that we will need in our Flask Project .
pip install langdetect
pip install pycountry
What is langdetect in Python?
A langdetect is a Python module for language detection. It is a port of the language-detection library for Java, which was developed by Nakatani Shuyo. The langdetect module provides a simple interface for detecting the language of a given text. It uses a set of language profiles to identify the language of the input text.
langdetect Example
This app uses the langdetect library to detect the language of a given sentence. The detect() function from this library takes a string as input and returns the detected language as a string. Here is an example of how to use the langdetect module to detect the language of a given text:
Python3
from langdetect import detect
text = "This is a sample text in English."
language = detect(text)
print (language)
|
Output:
en
What is Pycountry in Python?
The pycountry is a Python library for working with ISO country and language codes. It provides access to a wide range of data, including information about countries, languages, currencies, and time zones. The library includes a number of useful functions and data structures for working with country and language codes. For example, you can use the countries attribute to get a list of all countries, and the languages attribute to get a list of all languages.
Pycountry Example
Now you can see that your detect languages are displayed in codes (eg: ‘en’, ‘hi’, ‘es’, etc). Let’s convert these codes to language names.
Python3
from langdetect import detect
import pycountry
def get_language_name(language_code):
language = pycountry.languages.get(alpha_2 = language_code)
return language.name
text = "This is a sample text in English."
language = detect(text)
print ( 'The detected language is: {}' . format (get_language_name(language)))
|
Output:
The detected language is: English
Implementation of Flask Language Detector App
To use this app, you can send a GET request to the /detect-language route with a query parameter called sentence containing the sentence to be detected. For example, if you send a request to http://example.com/detect-language?sentence=Hello%20world, the app will return The detected language is: en (assuming the sentence is in English). You can also use this app in a form on a web page.
index.html
Here’s an example of how you could create a simple HTML form that submits a sentence to the Flask app and displays the detected language:
HTML
<!DOCTYPE html>
< html >
< head >
< title >Page Title</ title >
</ head >
< body >
< h2 >Welcome To GFG</ h2 >
< p >Detect your Language</ p >
< form action = "/detect-language" method = "GET" >
< label for = "sentence" >Enter a sentence:</ label >
< input type = "text" id = "sentence" name = "sentence" >
< input type = "submit" value = "Detect language" >
</ form >
</ body >
</ html >
|
app.py
Now you can see that your detect languages are displayed in codes (eg: ‘en’, ‘hi’, ‘es’, etc). Let’s convert these codes to language names. For doing so, we will be using `pycountry`. When the user submits the form, the app will receive a GET request with the sentence query parameter containing the entered sentence. The app will then detect the language of the sentence and return it as a response.
Python3
from flask import Flask, request, render_template
from langdetect import detect
import pycountry
def get_language_name(language_code):
language = pycountry.languages.get
(alpha_2 = language_code)
return language.name
app = Flask(__name__)
@app .route( '/' )
def home():
return render_template( "home.html" )
@app .route( '/detect-language' )
def detect_language():
sentence = request.args.get( 'sentence' )
language = detect(sentence)
return 'The detected language is: {}'
. format (get_language_name(language))
if __name__ = = '__main__' :
app.run(debug = True )
|
Output:
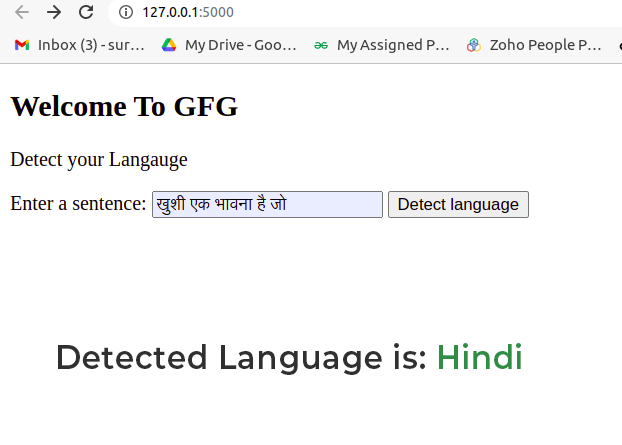
Input 1
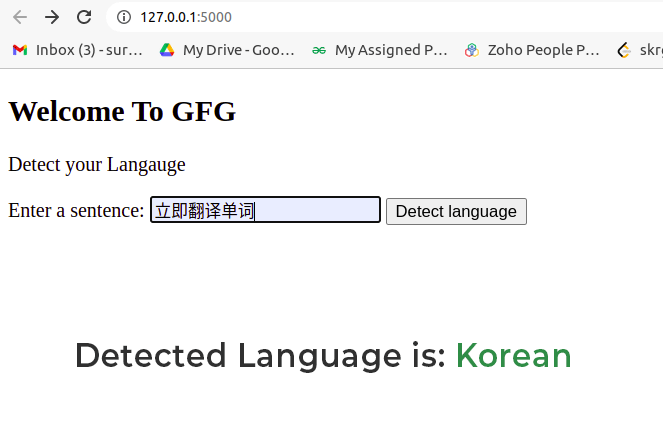
Input 2
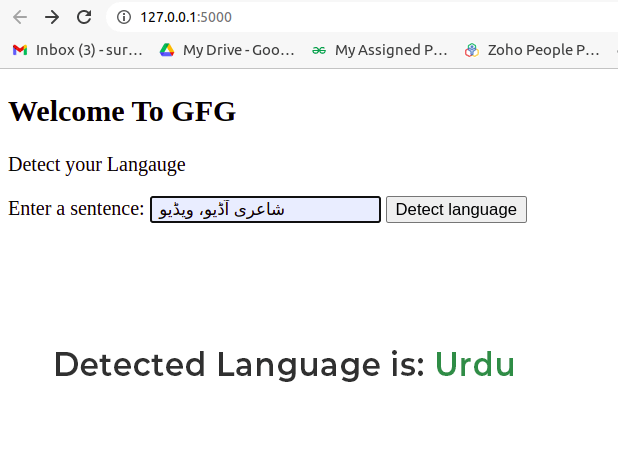
Input 3
Share your thoughts in the comments
Please Login to comment...