Find indices of elements equal to zero in a NumPy array
Last Updated :
08 Mar, 2023
Sometimes we need to find out the indices of all null elements in the array. Numpy provides many functions to compute indices of all null elements.
Method 1: Finding indices of null elements using numpy.where()
This function returns the indices of elements in an input array where the given condition is satisfied.
Syntax :
numpy.where(condition[, x, y])
When True, yield x, otherwise yield y
Python3
import numpy as np
n_array = np.array([ 1 , 0 , 2 , 0 , 3 , 0 , 0 , 5 ,
6 , 7 , 5 , 0 , 8 ])
print ( "Original array:" )
print (n_array)
print ("\nIndices of elements equal to zero of the \
given 1 - D array:")
res = np.where(n_array = = 0 )[ 0 ]
print (res)
|
Output:
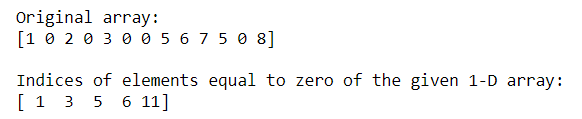
Time complexity: O(n) – where n is the size of the array
Auxiliary space: O(k) – where k is the number of null elements in the array, as we are storing their indices in a separate array.
Method 2: Finding indices of null elements using numpy.argwhere()
This function is used to find the indices of array elements that are non-zero, grouped by element.
Syntax :
numpy.argwhere(arr)
Python3
import numpy as np
n_array = np.array([[ 0 , 2 , 3 ],
[ 4 , 1 , 0 ],
[ 0 , 0 , 2 ]])
print ( "Original array:" )
print (n_array)
print ( "\nIndices of null elements:" )
res = np.argwhere(n_array = = 0 )
print (res)
|
Output:
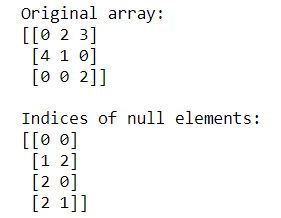
The time complexity of the code is O(m * n) where m and n are the dimensions of the 3-D Numpy array .
The auxiliary space complexity of the code is O(k) where k is the number of null elements in the 3-D Numpy array .
Method 3: Finding the indices of null elements using numpy.nonzero()
This function is used to Compute the indices of the elements that are non-zero. It returns a tuple of arrays, one for each dimension of arr, containing the indices of the non-zero elements in that dimension.
Syntax:
numpy.nonzero(arr)
Python3
import numpy as np
n_array = np.array([ 1 , 10 , 2 , 0 , 3 , 9 , 0 ,
5 , 0 , 7 , 5 , 0 , 0 ])
print ( "Original array:" )
print (n_array)
print ( "\nIndices of null elements:" )
res = np.nonzero(n_array = = 0 )
print (res)
|
Output:
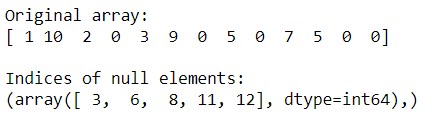
The time complexity is O(n), where n is the number of elements in the input array.
The auxiliary space complexity is O(k), where k is the number of null elements in the input array.
Method 4: Using numpy.extract() method
Use the numpy.extract() method. This method returns an array of values that satisfy a certain condition. In this case, we can use it to extract the indices of elements that are equal to zero.
Python3
import numpy as np
n_array = np.array([ 1 , 0 , 2 , 0 , 3 , 0 , 0 , 5 ,
6 , 7 , 5 , 0 , 8 ])
print ( "Original array:" )
print (n_array)
print ("\nIndices of elements equal to zero of the \
given 1 - D array:")
res = np.extract(n_array = = 0 , np.arange( len (n_array)))
print (res)
|
Output:
Original array:
[1 0 2 0 3 0 0 5 6 7 5 0 8]
Indices of elements equal to zero of the given 1-D array:
[ 1 3 5 6 11]
Time complexity: O(n), where n is the length of the input array.
Auxiliary space: O(m), where m is the number of elements in the input array that are equal to zero.
Share your thoughts in the comments
Please Login to comment...