Files Class writeString() Method in Java with Examples
Last Updated :
05 Feb, 2021
The writeString() method of File Class in Java is used to write contents to the specified file.
Syntax:
Files.writeString(path, string, options)
Parameters:
- path – File path with data type as Path
- string – a specified string which will enter in the file with return type String.
- options – Different options to enter the string in the file. Like append the string to the file, overwrite everything in the file with the current string, etc
Return Value: This method does not return any value.
Below are two overloaded forms of the writeString() method.
public static Path writeString​(Path path, CharSequence csq, OpenOption… options) throws IOException
public static Path writeString​(Path path, CharSequence csq, Charset cs, OpenOption… options) throws IOException
- UTF-8 charset is used to write the content to file in the first method.
- The second method does the same using the specified charset.
- How the file is opened is specified in Options.
Below is the implementation of the problem statement:
Java
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.StandardOpenOption;
public class GFG {
public static void main(String[] args)
{
Path filePath
= Paths.get( "/home/mayur/" , "temp" , "gfg.txt" );
try {
Files.writeString(filePath, "Hello from GFG !!" ,
StandardOpenOption.APPEND);
String content = Files.readString(filePath);
System.out.println(content);
}
catch (IOException e) {
e.printStackTrace();
}
}
}
|
Output:
Hello from GFG ! !
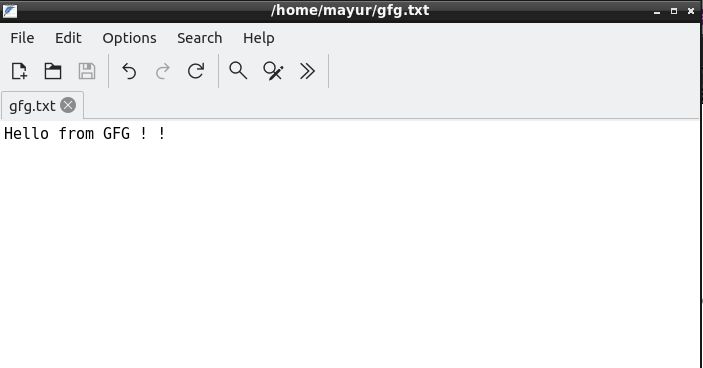
output File
Share your thoughts in the comments
Please Login to comment...