File Transfer using TCP Socket in Python
Last Updated :
17 Apr, 2023
In this article, we implement a well-known Protocol in Computer Networks called File Transfer Protocol (FTP) using Python. We use the TCP Socket for this, i.e. a connection-oriented socket. FTP (File Transfer Protocol) is a network protocol for transmitting files between computers over Transmission Control Protocol/Internet Protocol (TCP/IP) connections. FTP is commonly used to transfer files behind the scenes for other applications, such as banking services.
Prerequisites: Socket-Programming in Python
File Structure
TCP-SERVER.py: Server-Side Implementation
We start by importing the socket library and making a simple socket. AF_INET refers to the address-family ipv4. The SOCK_STREAM means connection-oriented TCP protocol. After this, we bind the host address and port number to the server.
The user enters the maximum number of client connections required. On the basis of the input, we determine the size of an array storing clients called Connections. The server then keeps listening for client connections and if found adds them to the Connections array. It does this until the Connections array reaches its maximum limit. Then, for every connection, processing starts,
- Data is received and then decoded.
- If data is not null, the server creates a file with the name “Output<a unique number>.txt” and writes it into it.
- This is continued until all data is written into the file
- The above happens for all clients and then connections are closed. Hence, we are able to receive a new file at the server’s end with the exact same data.
Python3
import socket
if __name__ = = '__main__' :
host = '127.0.0.1'
port = 8080
totalclient = int ( input ( 'Enter number of clients: ' ))
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
sock.bind((host, port))
sock.listen(totalclient)
connections = []
print ( 'Initiating clients' )
for i in range (totalclient):
conn = sock.accept()
connections.append(conn)
print ( 'Connected with client' , i + 1 )
fileno = 0
idx = 0
for conn in connections:
idx + = 1
data = conn[ 0 ].recv( 1024 ).decode()
if not data:
continue
filename = 'output' + str (fileno) + '.txt'
fileno = fileno + 1
fo = open (filename, "w" )
while data:
if not data:
break
else :
fo.write(data)
data = conn[ 0 ].recv( 1024 ).decode()
print ()
print ( 'Receiving file from client' , idx)
print ()
print ( 'Received successfully! New filename is:' , filename)
fo.close()
for conn in connections:
conn[ 0 ].close()
|
TCP-CLIENT.py: Client-side Implementation
We first create a socket for a client and connect it to the server using the tuple containing the host address and port number. Then, the User enters the file name it wants to send to the server. After this, using the open() function of Python, the data of the file is read. Data is read line-by-line and then encoded into binary and sent to the server using socket.send(). When sending data is finished, the file is closed. It keeps on asking for a filename from the user until the client connection is terminated.
Python3
import socket
if __name__ = = '__main__' :
host = '127.0.0.1'
port = 8080
sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
sock.connect((host, port))
while True :
filename = input ( 'Input filename you want to send: ' )
try :
fi = open (filename, "r" )
data = fi.read()
if not data:
break
while data:
sock.send( str (data).encode())
data = fi.read()
fi.close()
except IOError:
print ('You entered an invalid filename!\
Please enter a valid name')
|
Output:
We first run the server using the command.
python tcp-server.py
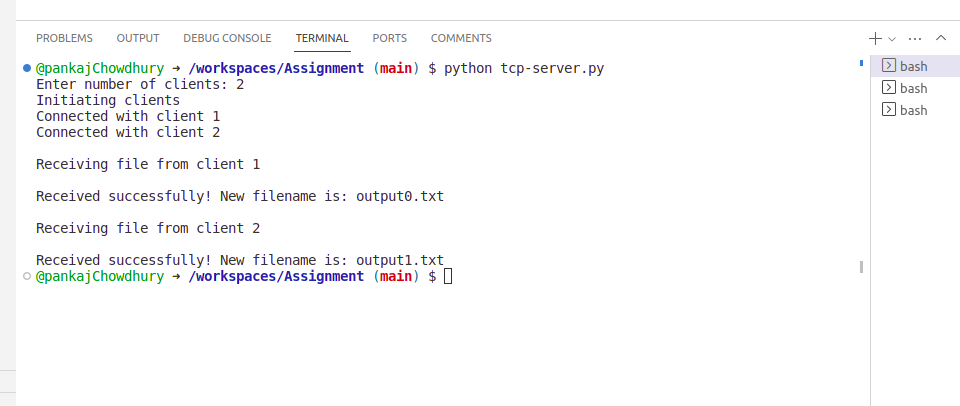
Server Side Output
After this, we specify the maximum number of client connections possible ( say 2).
- Clients’ connections are initialized, i.e., the Connections array is generated with size 2 to store clients.
- After this, we create a new terminal and run our client using the command.
python tcp-client.py
The maximum number of tabs where we can run the command in the terminal will be 2. As we are initializing our client with this. So we create two clients Client 1 and Client 2.
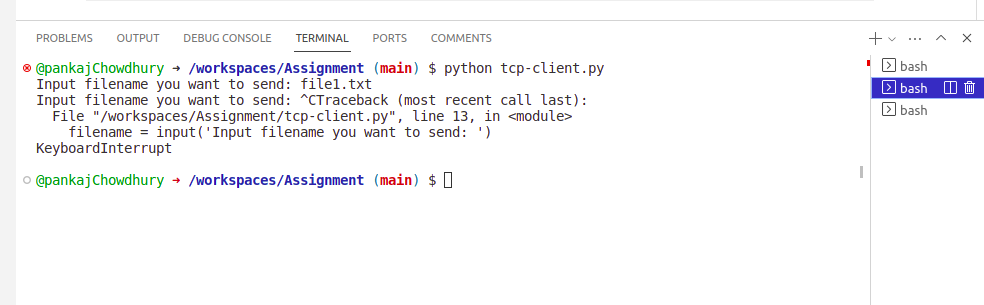
Client Side Output
Once the client is run, we see an output “Connected with client 1” on the server side, indicating the connection is completed successfully with the first client. Same for the second client also.
After this, on the client side, we give the file name we want to send. Here file1.txt is sent by both clients. The content of file1.txt is shown below:
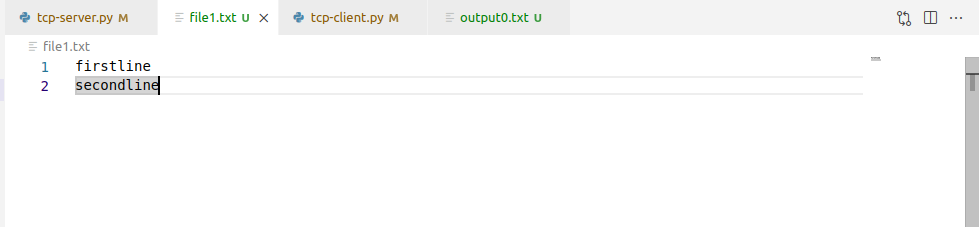
File sent to the server
Note that on the client side, we keep on showing the prompt: “Input filename you want to send:” so that a client can send multiple files. Once the connection with the client is disconnected, using “Control+C” in the terminal, a new file with the name Output<a number>.txt is generated on the server side with the same content, and the connection is closed. Here, the Client 1 file is stored as Output0.txt, and the Client 2 file is stored as Ouput1.txt.
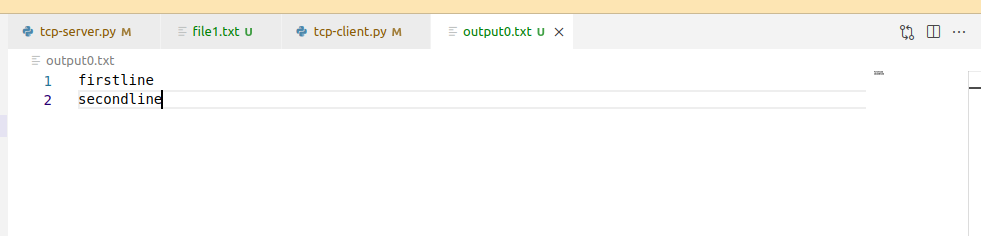
File Received from Client 1
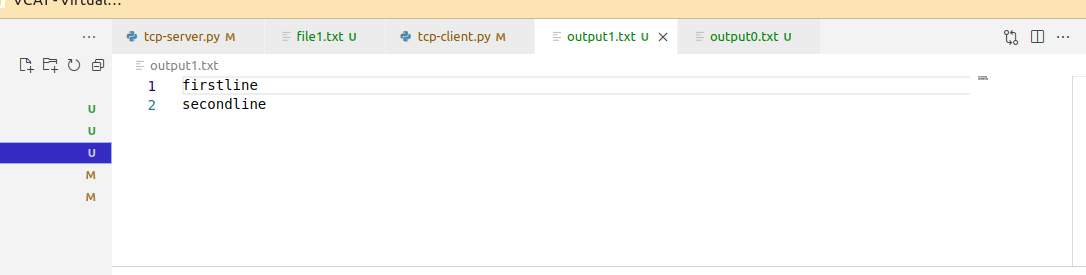
File Received from Client 2
Video for Reference
Share your thoughts in the comments
Please Login to comment...