File.Create(String, Int32, FileOptions) Method in C# with Examples
Last Updated :
20 Jul, 2021
File.Create(String, Int32, FileOptions) is an inbuilt File class method that is used to overwrite an existing file, specifying a buffer size and options that describe how to create or overwrite the file else create a new file if the specified file is not existing.
Syntax:
public static System.IO.FileStream Create (string path, int bufferSize, System.IO.FileOptions options);
Parameter: This function accepts three parameters which are illustrated below:
- path: This is the specified file path.
- bufferSize: This is the specified buffer size.
- options: This is one of the FileOptions values that describes how to create or overwrite the file.
Exceptions:
- UnauthorizedAccessException: The caller does not have the required permission. OR the path specified a file that is read-only. OR the path specified a file that is hidden.
- ArgumentException: The path is a zero-length string, contains only white space, or one or more invalid characters as defined by InvalidPathChars.
- ArgumentNullException: The path is null.
- PathTooLongException: The specified path, file name, or both exceed the system-defined maximum length.
- DirectoryNotFoundException: The given path is invalid.
- IOException: An I/O error occurred while creating the file.
- NotSupportedException: The path is in an invalid format.
Return Value: Returns a new file with the specified buffer size.
Below are the programs to illustrate the File.Create(String, Int32, FileOptions) method.
Program 1: Initially, no file is created. But below code itself create a new file file.txt with the specified contents.
C#
using System;
using System.IO;
using System.Text;
class GFG {
public static void Main()
{
string myfile = @"file.txt" ;
using (FileStream fs = File.Create(myfile, 1024, FileOptions.RandomAccess))
{
Byte[] info = new UTF8Encoding( true ).GetBytes( "GeeksforGeeks" );
fs.Write(info, 0, info.Length);
}
using (StreamReader sr = File.OpenText(myfile))
{
string s = "" ;
while ((s = sr.ReadLine()) != null ) {
Console.WriteLine(s);
}
}
}
}
|
Executing:
mcs -out:main.exe main.cs
mono main.exe
GeeksforGeeks
After running the above code, above output is shown and a new file file.txt is created with some specified contents shown below:
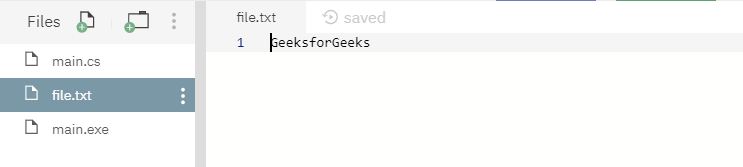
Program 2: The below shown file file.txt is created before running the below code.
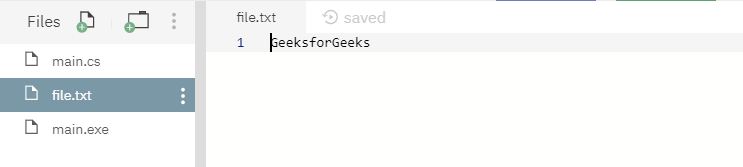
C#
using System;
using System.IO;
using System.Text;
class GFG {
public static void Main()
{
string myfile = @"file.txt" ;
using (FileStream fs = File.Create(myfile, 1024, FileOptions.RandomAccess))
{
Byte[] info = new UTF8Encoding( true ).GetBytes( "GFG is a CS Portal." );
fs.Write(info, 0, info.Length);
}
using (StreamReader sr = File.OpenText(myfile))
{
string s = "" ;
while ((s = sr.ReadLine()) != null ) {
Console.WriteLine(s);
}
}
}
}
|
Executing:
mcs -out:main.exe main.cs
mono main.exe
GFG is a CS Portal.
After running the above code, the above output is shown, and existing file contents get overwritten.
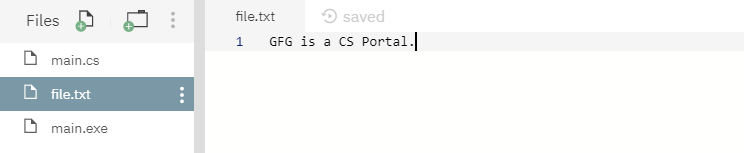
Share your thoughts in the comments
Please Login to comment...