Extension Method in C#
Last Updated :
13 Mar, 2024
In C#, the extension method concept allows you to add new methods in the existing class or in the structure without modifying the source code of the original type and you do not require any kind of special permission from the original type and there is no need to re-compile the original type. It is introduced in C# 3.0.
Let us discuss this concept with the help of an example. Suppose you have a class or a structure that contains three methods and you want to add two new methods in this class or structure, you do not have the source code of the class/structure or do not have permissions from the class/structure, or the class is a sealed class, but you still want to add new methods in it, then you can use the concept extension method to add the new method in the existing class/structure.
Now you create a new class that is static and contains the two methods that you want to add to the existing class, now bind this class with the existing class. After binding you will see the existing class can access the two newly added methods. As shown in the below program.
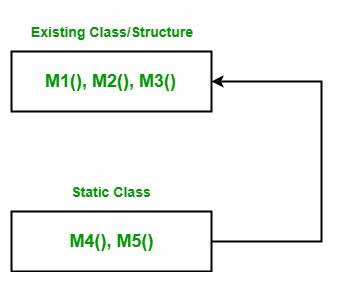
Example:
First we create a class named as Geek in Program1.csfile. It contains three methods that is M1(), M2(), and M3().
C#
// C# program to illustrate the concept
// of the extension methods
using System;
namespace ExtensionMethod {
// Here Geek class contains three methods
// Now we want to add two more new methods in it
// Without re-compiling this class
class Geek {
// Method 1
public void M1()
{
Console.WriteLine("Method Name: M1");
}
// Method 2
public void M2()
{
Console.WriteLine("Method Name: M2");
}
// Method 3
public void M3()
{
Console.WriteLine("Method Name: M3");
}
}
}
Now we create a static class named as NewMethodClass in Program2.cs file. It contains two methods that are M4() and M5(). Now we want to add these methods in Geek class, so we use the binding parameter to bind these methods with Geek class. After that, we create another named as GFG in which Geek class access all the five methods.
C#
// C# program to illustrate the concept
// of the extension methods
using System;
namespace ExtensionMethod {
// This class contains M4 and M5 method
// Which we want to add in Geek class.
// NewMethodClass is a static class
static class NewMethodClass {
// Method 4
public static void M4(this Geek g)
{
Console.WriteLine("Method Name: M4");
}
// Method 5
public static void M5(this Geek g, string str)
{
Console.WriteLine(str);
}
}
// Now we create a new class in which
// Geek class access all the five methods
public class GFG {
// Main Method
public static void Main(string[] args)
{
Geek g = new Geek();
g.M1();
g.M2();
g.M3();
g.M4();
g.M5("Method Name: M5");
}
}
}
Output:
Method Name: M1
Method Name: M2
Method Name: M3
Method Name: M4
Method Name: M5
Important Points:
- Here, Binding parameters are those parameters which are used to bind the new method with the existing class or structure. It does not take any value when you are calling the extension method because they are used only for binding not for any other use. In the parameter list of the extension method binding parameter is always present at the first place if you write binding parameter to second, or third, or any other place rather than first place then the compiler will give an error. The binding parameter is created using this keyword followed by the name of the class in which you want to add a new method and the parameter name. For example:
this Geek g
Here, this keyword is used for binding, Geek is the class name in which you want to bind, and g is the parameter name. - Extension methods are always defined as a static method, but when they are bound with any class or structure they will convert into non-static methods.
- When an extension method is defined with the same name and the signature of the existing method, then the compiler will print the existing method, not the extension method. Or in other words, the extension method does not support method overriding.
- You can also add new methods in the sealed class also using an extension method concept.
- It cannot apply to fields, properties, or events.
- It must be defined in top-level static class.
- Multiple binding parameters are not allowed means an extension method only contains a single binding parameter. But you can define one or more normal parameter in the extension method.
Advantages:
- The main advantage of the extension method is to add new methods in the existing class without using inheritance.
- You can add new methods in the existing class without modifying the source code of the existing class.
- It can also work with sealed class.
Share your thoughts in the comments
Please Login to comment...