Escape Sequences in Java
Last Updated :
01 Nov, 2023
A character with a backslash (\) just before it is an escape sequence or escape character. We use escape characters to perform some specific task. The total number of escape sequences or escape characters in Java is 8. Each escape character is a valid character literal. The list of Java escape sequences:
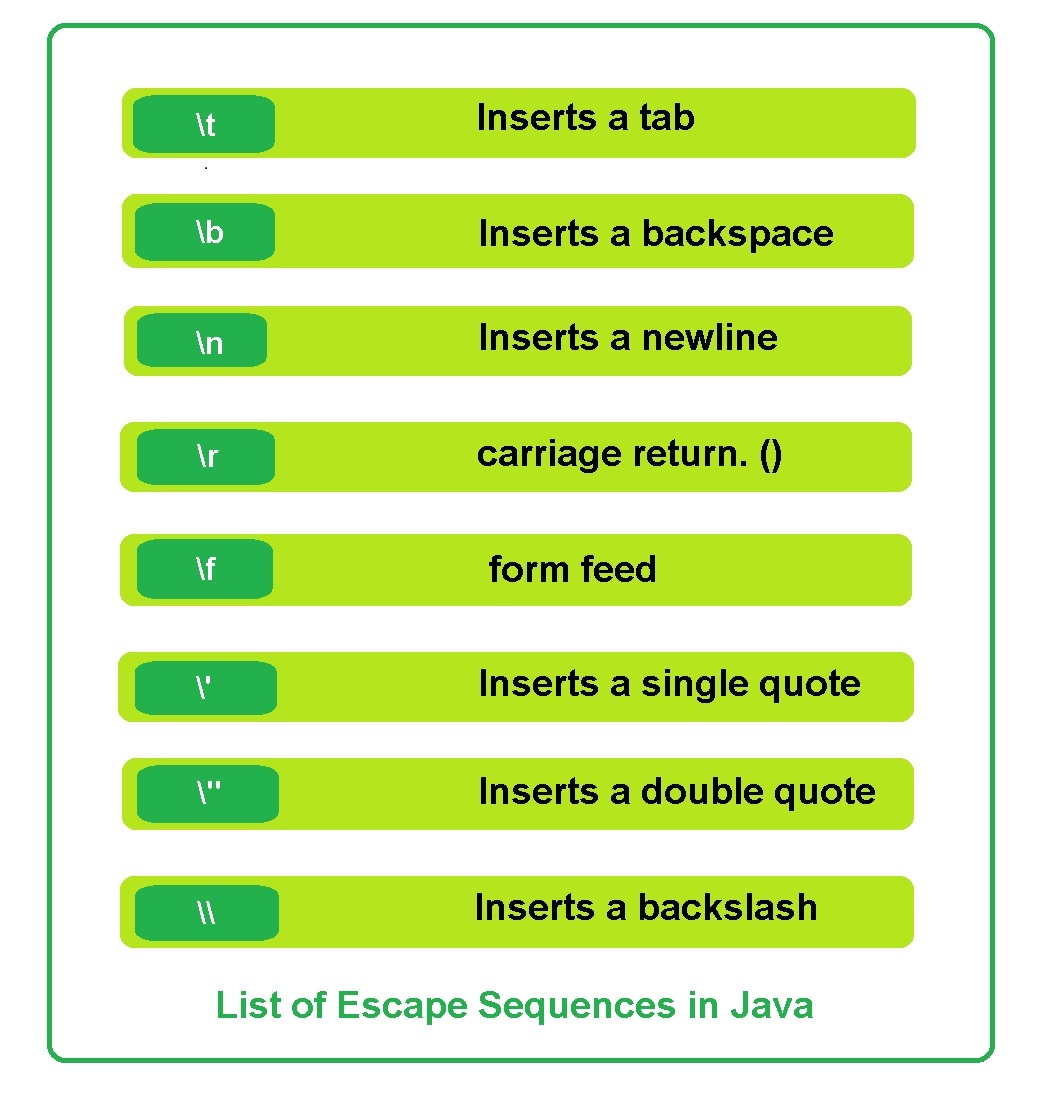
Why will we need Escape sequence?
Suppose we would like to run the subsequent java code:
Java
public class Test {
public static void main(String[] args)
{
System.out.println("Hi geek, welcome to " GeeksforGeeks ".");
}
}
|
This code gives a compile time error as :
prog.java:3: error: ')' expected
System.out.println("Hi geek, welcome to "GeeksforGeeks".");
^
prog.java:3: error: not a statement
System.out.println("Hi geek, welcome to "GeeksforGeeks".");
^
prog.java:3: error: ';' expected
System.out.println("Hi geek, welcome to "GeeksforGeeks".");
^
3 errors
This happened because the compiler expects nothing but only strings inside the quotation mark but when the compiler found a quotation mark, it expects another quotation mark in the near future (the closing one) and between them, the string of text should be created. During this case, the quotation marks of the word “GeeksforGeeks” gets nested(inside another quotation marks). Once the compiler reaches here, the compiler gets confused. According to the rule, the quotation mark suggests the compiler for creating a string but the compiler was busy with doing that thing previously and the code gives us a compile-time error. So we should provide proper instructions to the compiler about the quotation mark. i.e, when a quotation is used for creating a string(as a command) and when it is a character itself (the part of the output string). Similar sorts of confusion arise with other characters also(like- backslashes(), single and double quotation mark (‘, ”)) and these also provide a compile-time error in every case. For Solving these sorts of issues we have to use the java character escaping.
Control Sequence:
A control sequence is nothing however the backslash(\) glued with a character (the character which has to be escaped) is called a control sequence.
Example:
\\ is a control sequence used for displaying a backslash as output. so let’s use this concept in the previous java code to avoid the compile-time error:
Java
public class Test {
public static void main(String[] args)
{
System.out.println("Hi geek, welcome to \"GeeksforGeeks\".");
}
}
|
Output: Hi geek, welcome to "GeeksforGeeks".
Some Coding Examples of Java Escape Characters
- Java code for the escape sequence \t:
Java
public class Test {
public static void main(String[] args)
{
System.out.println("Good Morning\t Geeks! ");
}
}
|
Output:
Good Morning Geeks!
- Java code for the escape sequence \b :
Java
public class Test {
public static void main(String[] args)
{
System.out.println("Good Morning\bg Geeks! ");
}
}
|
Output:(The output depends upon compiler)
Good Morning Geeks!
- Java code for the escape sequence \n :
Java
public class Test {
public static void main(String[] args)
{
System.out.println("Good Morning Geeks! \n How are you all? ");
}
}
|
Output:
Good Morning Geeks!
How are you all?
- Java code for the escape sequence \r:
Java
public class Test {
public static void main(String[] args)
{
System.out.println("Good Morning Geeks! \r How are you all? ");
}
}
|
Output:(The output depends upon compiler)
Good Morning Geeks!
How are you all?
- Java code for the escape sequence \f:
Java
public class Test {
public static void main(String[] args)
{
System.out.println("Good Morning Geeks! \f How are you all? ");
}
}
|
Output:(The output depends upon compiler)
Good Morning Geeks!
How are you all?
- Java code for the escape sequence \’
Java
public class Gfg {
public static void main(String[] args)
{
System.out.println("Good Morning \'Geeks!\' How are you all? ");
}
}
|
Output:
Good Morning 'Geeks!' How are you all?
- Java code for the escape sequence \”
Java
public class Gfg {
public static void main(String[] args)
{
System.out.println("Good Morning \"Geeks!\" How are you all? ");
}
}
|
Output:
Good Morning "Geeks!" How are you all?
- Java code for the escape sequence \\
Java
public class Gfg {
public static void main(String[] args)
{
System.out.println("\\- this is a backslash. ");
}
}
|
Output:
\- this is a backslash.
Explanation:It contains two backslashes, this means after reading the first \ the compiler read the next \ as a new character.
Share your thoughts in the comments
Please Login to comment...