Design a Length Converter in Tailwind CSS
Last Updated :
01 May, 2024
The Length Converter is a web application that allows users to convert lengths between different units of measurement namely – meters, centimeters, kilometers, inches, feet, yards and miles.
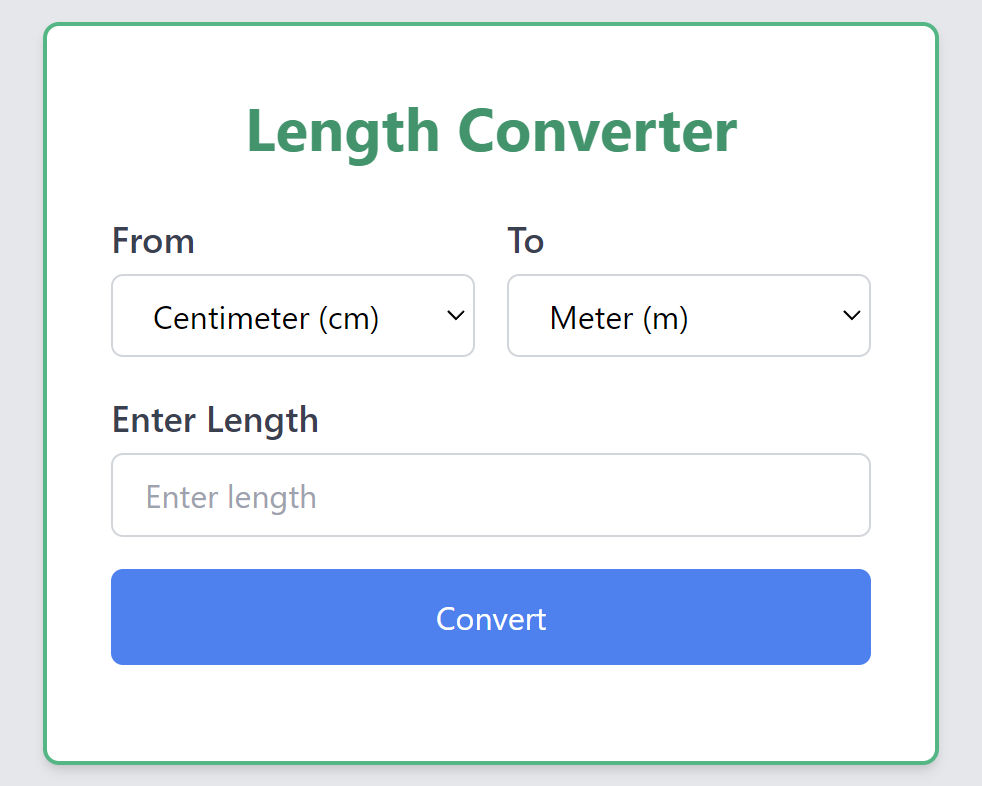
Length Converter
Prerequisites:
Approach
- Create an HTML document with Tailwind CSS for styling.
- Create form elements for selecting ‘from’ and ‘to’ units, and an input for length.
- Create a JavaScript file and link it to the HTML document using script tag.
- Create a JavaScript function to calculate length conversions based on selected units and input.
- Ensure the input length is a valid number & display an alert message if it is not.
Example: This example shows the implementation of above-explained approach.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<title>Length Converter</title>
<link href=
"https://cdn.jsdelivr.net/npm/tailwindcss@2.2.19/dist/tailwind.min.css"
rel="stylesheet">
</head>
<body class="bg-gray-200 min-h-screen flex
items-center justify-center">
<div class="bg-white border-2 border-green-500
p-8 rounded-lg shadow-md w-full max-w-md">
<h1 class="text-3xl font-bold text-center
text-green-600 mb-6">
Length Converter
</h1>
<div class="grid grid-cols-2 gap-4">
<div>
<label for="fromUnit" class="block text-lg
font-semibold text-gray-700">
From
</label>
<select id="fromUnit" class="mt-1 block w-full px-4
py-2 border border-gray-300
rounded-md focus:outline-none
focus:ring-2 focus:ring-blue-500
focus:border-transparent">
<option value="m">Meter (m)</option>
<option value="cm" selected>Centimeter (cm)</option>
<option value="km">Kilometer (km)</option>
<option value="in">Inch (in)</option>
<option value="ft">Feet (ft)</option>
<option value="yd">Yard (yd)</option>
<option value="mi">Mile (mi)</option>
</select>
</div>
<div>
<label for="toUnit" class="block text-lg
font-semibold text-gray-700">
To
</label>
<select id="toUnit" class="mt-1 block w-full px-4
py-2 border border-gray-300
rounded-md focus:outline-none
focus:ring-2 focus:ring-blue-500
focus:border-transparent">
<option value="m" selected>Meter (m)</option>
<option value="cm">Centimeter (cm)</option>
<option value="km">Kilometer (km)</option>
<option value="in">Inch (in)</option>
<option value="ft">Feet (ft)</option>
<option value="yd">Yard (yd)</option>
<option value="mi">Mile (mi)</option>
</select>
</div>
</div>
<div class="mt-4">
<label for="inputLength" class="block text-lg
font-semibold text-gray-700">
Enter Length
</label>
<input type="number" id="inputLength"
placeholder="Enter length"
class="mt-1 block w-full px-4
py-2 border border-gray-300
rounded-md focus:outline-none
focus:ring-2 focus:ring-blue-500
focus:border-transparent">
</div>
<div class="mt-4">
<button onclick="convertLength()"
class="w-full px-6 py-3 bg-blue-500
text-white rounded-md
hover:bg-blue-600 focus:outline-none
focus:ring-2 focus:ring-blue-500
focus:ring-opacity-50">
Convert
</button>
</div>
<div id="result" class="mt-4 text-xl font-semibold
text-gray-800 text-center">
</div>
</div>
<script src="script.js"></script>
</body>
</html>
Javascript
function convertLength() {
const fromUnit =
document.getElementById('fromUnit').value;
const toUnit =
document.getElementById('toUnit').value;
const inputLength =
parseFloat(document.getElementById('inputLength').value);
let result;
if (fromUnit === 'cm' && toUnit === 'km') {
result = inputLength / 100000;
} else {
const conversions = {
m: 1, // Meter
cm: 0.01, // Centimeter
km: 1000, // Kilometer
in: 0.0254, // Inch
ft: 0.3048, // Feet
yd: 0.9144, // Yard
mi: 1609.34 // Mile
};
result = inputLength * conversions[fromUnit] / conversions[toUnit];
}
if (result < 0.01) {
document.getElementById('result').textContent =
`${inputLength} ${fromUnit} = < 0.01 ${toUnit}`;
} else {
document.getElementById('result').textContent =
`${inputLength} ${fromUnit} = ${result.toFixed(2)} ${toUnit}`;
}
}
Output:
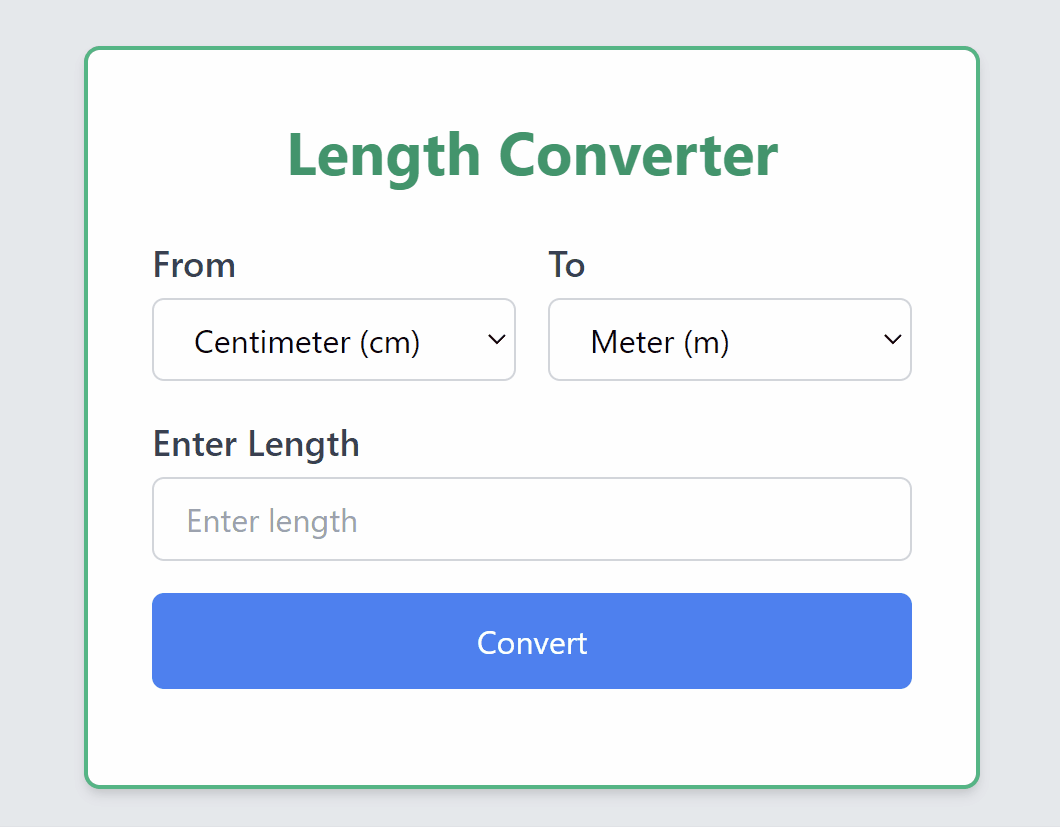
Length Converter
Share your thoughts in the comments
Please Login to comment...