Deflater getAdler() function in Java with examples
Last Updated :
24 Jun, 2019
The getAdler() function of the Deflater class in java.util.zip returns the Adler-32 value of the uncompressed data. Adler-32 is a checksum algorithm which is widely used zlib compression library.
Function Signature:
public int getAdler()
Syntax:
d.getAdler();
Parameter: The function requires no parameter
Return Type: The function returns an Integer value which is the Adler-32 value of the uncompressed data.
Exception: The function does not throw any exception
Example 1:
import java.util.zip.*;
import java.io.UnsupportedEncodingException;
class GFG {
public static void main(String args[])
throws UnsupportedEncodingException
{
Deflater d = new Deflater();
String pattern = "GeeksforGeeks" , text = "" ;
for ( int i = 0 ; i < 4 ; i++)
text += pattern;
d.setInput(text.getBytes( "UTF-8" ));
d.finish();
byte output[] = new byte [ 1024 ];
int size = d.deflate(output);
System.out.println( "Compressed String :"
+ new String(output)
+ "\n Size " + size);
System.out.println( "Original String :" + text
+ "\n Size " + text.length());
System.out.println( "Adler-32 value :"
+ d.getAdler());
d.end();
}
}
|
Output:
Compressed String :x?sOM?.N?/r???q??
Size 21
Original String :GeeksforGeeksGeeksforGeeksGeeksforGeeksGeeksforGeeks
Size 52
Adler-32 value :511972501
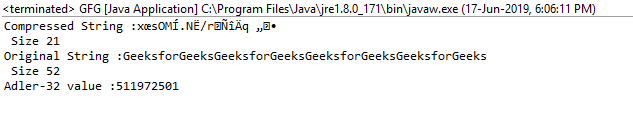
Reference: https://docs.oracle.com/javase/7/docs/api/java/util/zip/Deflater.html#getAdler()
Share your thoughts in the comments
Please Login to comment...