D3.js node.eachBefore() Function
Last Updated :
31 Aug, 2020
The node.eachBefore() function is used to invoke a particular function for each node but in a pre-order-traversal order. It visits each node in pre-traversal order and performs an operation on that particular node and each of its descendants.
Syntax:
node.eachBefore(function);
Parameters: This function accepts a single parameter as mentioned above and described below:
- function: This is the function that is to be invoked for each node.
Return Value: This function does not return anything.
Example 1:
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" />
< meta name = "viewport" path1tent =
"width=device-width, initial-scale = 1.0" />
</ script >
</ head >
< body >
< script >
// Constructing a tree
var tree = {
// Root node
name: "rootNode",
children: [
{
// Child of root node
name: "child1",
value: 2
},
{
// Child of root node
name: "child2",
value: 3,
children: [
{
// Child of child2
name: "grandchild1",
value: 1,
children: [
{
// Child of grandchild1
name: "grand_granchild1_1",
value: 4
},
{
// Child of grandchild1
name: "grand_granchild1_2",
value: 5
}
]
},
{
name: "grandchild2",
children: [
{
// Child of grandchild2
name: "grand_granchild2_1"
},
{
// Child of grandchild2
name: "grand_granchild2_2"
}
]
}
]
}
]
};
var obj = d3.hierarchy(tree);
const BFS = [];
obj.eachBefore(d => BFS.push
(
" ".repeat(d.depth)
+ `${d.depth}: ${d.data.name}`
));
BFS.forEach((e) => {
console.log("level:", e);
})
</ script >
</ body >
</ html >
|
Output:
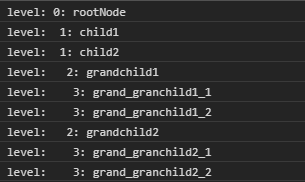
Example 2:
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" />
< meta name = "viewport" path1tent=" width = device -width,
initial-scale = 1 .0" />
</ script >
</ head >
< body >
< script >
var obj = d3.hierarchy({
// Root node
name: "rootNode",
children: [
{
// Child of root node
name: "child1",
value: 2
},
{
// Child of root node
name: "child2",
value: 3,
children: [
{
// Child of child2
name: "grandchild1",
value: 1,
children: [{
// Child of grandchild1
name: "grand_granchild1_1",
value: 4
},
{
// Child of grandchild1
name: "grand_granchild1_2",
value: 5
}]
}
]
}
]
});
const BFS = [];
obj.eachBefore(d => BFS.push
(
" ".repeat(d.depth)
+ `${d.depth}: ${d.data.name}`
));
console.log(BFS);
</ script >
</ body >
</ html >
|
Output:

Share your thoughts in the comments
Please Login to comment...