Creating SVG Image using PyCairo
Last Updated :
12 Nov, 2020
In this article we will see how we can create SVG file using PyCairo in Python.
SVG : An SVG file is a graphics file that uses a two-dimensional graphic vector format that defines images using an XML-based text format. As a standard format for showing vector graphics on the web, SVG files are developed.
PyCairo : It is a Python module providing bindings for the cairo graphics library.This library is used for creating SVG i.e vector files in python. In order to install the pycairo module we will use the command given below
Installation:
pip install pycairo
Opening SVG file : The easiest and quickest way to open an SVG file to view it (read only) is with a modern web browser like Chrome, Firefox, Edge, or Internet Explorer—nearly all of them should provide some sort of rendering support for the SVG format.
Steps-by-step Approach:
1. Import the cairo module.
2. Create an SVG surface and add context to it.
3. For creating a small face add two rectangles for eyes.
4. Add a curve line for the smile.
5. Set the color and width of the context.
Below is the complete program based on the above approach:
Python3
import cairo
with cairo.SVGSurface( "geek.svg" , 700 , 700 ) as surface:
context = cairo.Context(surface)
context.rectangle( 100 , 100 , 100 , 100 )
context.rectangle( 500 , 100 , 100 , 100 )
x, y, x1, y1 = 0.1 , 0.5 , 0.4 , 0.9
x2, y2, x3, y3 = 0.4 , 0.1 , 0.9 , 0.6
context.scale( 700 , 700 )
context.set_line_width( 0.04 )
context.move_to(x, y)
context.curve_to(x1, y1, x2, y2, x3, y3)
context.set_source_rgba( 0.4 , 1 , 0.4 , 1 )
context.stroke()
print ( "File Saved" )
|
Output:
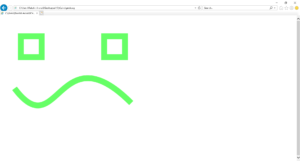
Share your thoughts in the comments
Please Login to comment...