Creating and Executing a .jar File in Linux Terminal
Last Updated :
06 Jul, 2021
JAR – Java Archive. It is like a zip file but for java classes. It combines all the .class files in Java into a single .jar file. It is used to download all java classes on HTTP in a single operation. These can be created using the “jar” CLI tool. It also has an optional META-INF which can include files like –
- MANIFEST.MF – manifest file is used to define the extension and package-related data.
- INDEX.LIST – It contains location information for packages defined in an application or extension.
- x.SF – This is the signature file where ‘x’ is the base file name.
- x.DSA – This file stores the digital signature of the corresponding signature file.
- services/ – This directory stores all the service provider configuration files.
The most common and majorly used file is MANIFEST.MF
Requirements
Java (JDK + JRE) must be installed. Check by using command –
Java --version
jar --version
Create Jar files
Let us consider 4 class files – Class1, Class2, Class3, Class4
Java
class Class2 {
public static void cls2Method(){
System.out.println( " Hello from Class2 " );
}
}
class Class3 {
public static void cls3Method(){
System.out.println( " Hello from Class3 " );
}
}
class Class4 {
public static void cls4Method(){
System.out.println( " Hello from Class4 " );
}
}
public class Class1 {
public static void main(String[] args){
System.out.println( " Hello from Class1 " );
Class2.cls2Method();
Class3.cls3Method();
Class4.cls4Method();
}
}
|
Output
Hello from Class1
Hello from Class2
Hello from Class3
Hello from Class4
Let’s move them into one jar file called “allClasses.jar”.
Run the command:
jar –create –file allClasses.jar Class1.class Class2.class Class3.class
To get a clear output use –verbose
jar –create –verbose –file allClasses.jar Class1.class Class2.class Class3.class
Output:

This will create an allClasses.jar file in the folder. Let’s understand the above command thoroughly.
- –create: It is an option to create a jar file. We can perform more operations like extract, update, etc.
- –verbose: It gives a crisp and clear output and shows what’s going on behind the scenes.
- –file filename: filename is the name for the jar file. Extension(.jar) is optional.
- In the end, we specify the whole list of files to put in the jar file.
The shorthand for this command will be –
jar -cvf allClasses.jar *
Note: * represents all the files in the current folder. Use * with caution.
To update,
jar -uf allClasses.jar Class4.class
-u is for the –update.
This will update allClasses.jar files with the new Class4.class.
From the verbose output, it is clear that compression is taking place, to bypass compression or to archive files without compression use the option –no-compress.
jar –create –verbose –no-compress –file allClasses.jar Class1.class Class2.class Class3.class
or
jar -cvf0 allClasses.jar *
Output:
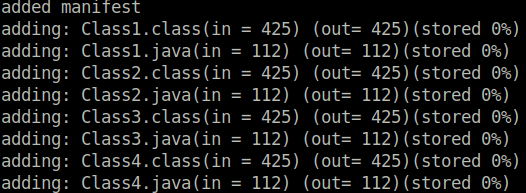
Execute Jar Files
Execution is fairly simple of jar files. Just use the command
java -jar allClasses.jar
If this gives an error – no main manifest attribute, in allClasses.jar
Open ./META-INF/MANIFEST.MF file and add a line in it.
Main-Class: classname
In our case, the class name will be “Class1” as it’s our main class.
Now, the file will look like this:-
Manifest-Version: 1.0
Created-By: Ubuntu
Main-Class: Class1
Again run the command
java -jar allClasses.jar
Output:
Hello from Class1
Hello from Class2
Hello from Class3
Hello from Class4
Caution: Don’t leave any space between lines in the MANIFEST.MF file else it will show the unexpected error.
If you still get the error and not able to find the error, use the below workaround –
java -cp allClasses.jar Class1
where Class1 is the name of the main class.
Note:
To Extract use command –
jar --extract --file allClasses.jar
or
jar -xf allClasses.jar
Share your thoughts in the comments
Please Login to comment...