Creating a Personalized Greeting Card with HTML CSS and JavaScript
Last Updated :
26 Dec, 2023
In this article, we are going to learn how to create a Personalized Greeting Card with HTML, CSS, and JavaScript.
Output Preview:
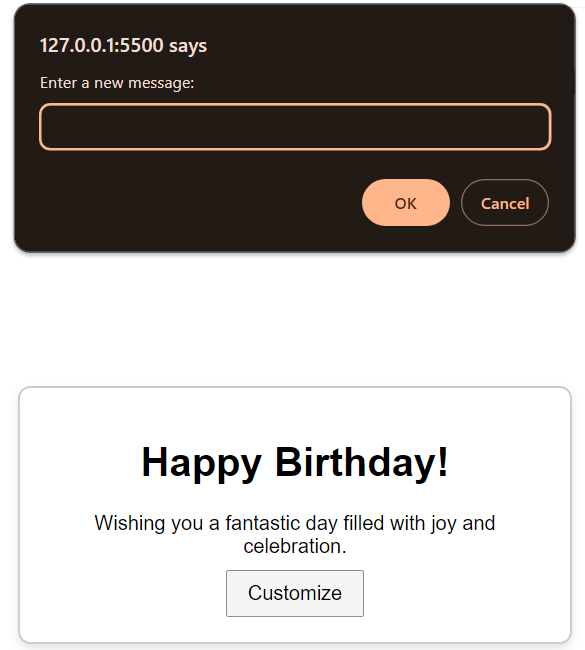
Prerequisites
Approach:
- Create a JavaScript function called
customizeCard
.
- Utilize the
prompt
function to gather user input for a new greeting and a new message.
- Validate user input by checking if both the new greeting and new message are not null.
- Use
document.getElementById
to select HTML elements with the IDs ‘greeting’ and ‘message’.
- Update the text content of these elements with the user-provided values, effectively customizing the card based on the entered information.
Javascript
function customizeCard() {
let newGreeting = prompt( "Enter a new greeting:" );
let newMessage = prompt( "Enter a new message:" );
if (newGreeting !== null && newMessage !== null ) {
document.getElementById( 'greeting' )
.textContent = newGreeting;
document.getElementById( 'message' )
.textContent = newMessage;
}
}
|
HTML
<!DOCTYPE html>
< html lang = "en" >
< head >
< meta charset = "UTF-8" >
< meta name = "viewport"
content = "width=device-width, initial-scale=1.0" >
< link rel = "stylesheet" href = "styles.css" >
< title >Personalized Greeting Card</ title >
</ head >
< body >
< div class = "card" >
< h1 id = "greeting" >Happy Birthday!</ h1 >
< div id = "message" >
Wishing you a fantastic day filled
with joy and celebration.
</ div >
< button onclick = "customizeCard()" >
Customize
</ button >
</ div >
< script src = "script.js" ></ script >
</ body >
</ html >
|
CSS
body {
font-family : 'Arial' , sans-serif ;
display : flex;
align-items: center ;
justify- content : center ;
height : 100 vh;
margin : 0 ;
}
.card {
text-align : center ;
padding : 20px ;
border : 2px solid #ccc ;
border-radius: 10px ;
max-width : 400px ;
background-color : #fff ;
box-shadow: 0 4px 8px rgba( 0 , 0 , 0 , 0.1 );
}
button {
margin-top : 10px ;
padding : 8px 16px ;
font-size : 16px ;
cursor : pointer ;
}
|
Output:
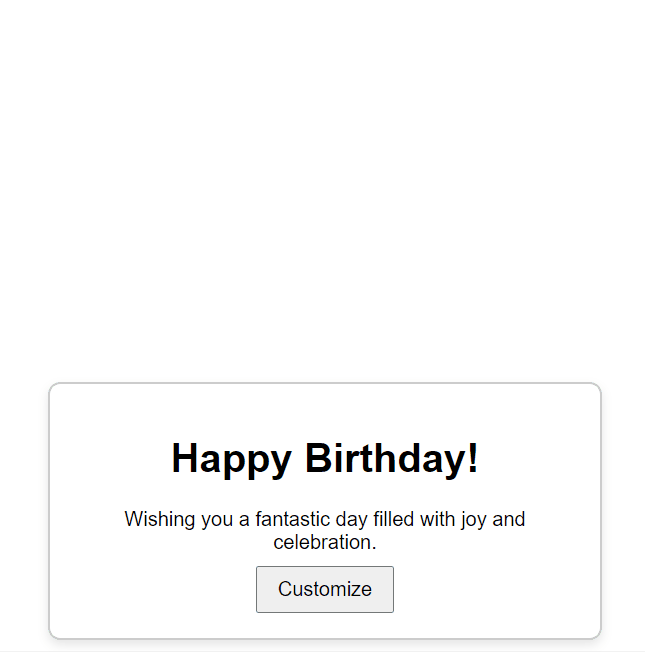
Share your thoughts in the comments
Please Login to comment...