Creating a PDF Merger using Javascript and Express Js
Last Updated :
13 Mar, 2024
Certain forms require us to upload multiple documents as a single PDF file. We merge those files using any online PDF merger in such cases. This article focuses on creating our own PDF merger using Javascript and Express JS.
Prerequisites:
Syntax:
import PDFMerger from 'pdf-merger-js';
const merger = new PDFMerger();
Steps to Create the Application:
Step 1: Create a folder called “PDFTools” using the following command:
mkdir PDFTools
cd PDFTools
Step 2: Assuming Node.js has been installed in the device, set an npm package inside the above directory created using the below command.
npm init
Step 3: Make the following installations:
npm i nodemon –save-dev multer pdf-merger-js
Step 4: Add “type”: “module” inside package.json, since all modules are ECMAScript modules.
Step 5: Inside package.json, under “scripts”, add “start”: “nodemon server.js”.
The updated dependencies in package.json:
"dependencies": {
"express": "^4.18.3",
"multer": "^1.4.5-lts.1",
"pdf-merger-js": "^5.1.1"
}
Folder Structure:
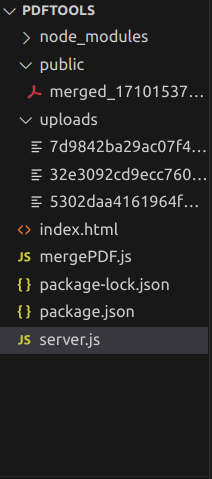
Folder Structure
The updated dependencies in package.json:
"dependencies": {
"express": "^4.18.3",
"multer": "^1.4.5-lts.1",
"pdf-merger-js": "^5.1.1"
}
Approach to Create a PDF Merger:
Explanation:
- For serving all static files which are inside the “public” folder:
app.use('/static', express.static('public'));
- For uploading the pdfs using the “name” attribute of the HTML input tag and allowing the upload of a maximum of 10 pdfs:
upload.array('pdfs', 10)
- For fetching the file objects:
req.files
- For sending the path of the files where they have been uploaded as arguments for the merge operation
for (let file of req.files) {
mergedFilePaths.push(path.join(__dirname, file.path));
}
merger.add(pdfFile);
- For saving the final merged PDF inside the public folder:
merger.save(`public/${mergedFileName}`);
Example: Below is the code exampple
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>PDF Merger</title>
</head>
<body>
<div>
<h2>PDFMerger - The Best Tool for Merging PDFs</h2>
<form method="post" action="/merge" enctype="multipart/form-data">
<input type="file" id="myFile" name="pdfs" multiple accept=".pdf">
<br><br>
<input type="submit" value="Merge">
</form>
</div>
</body>
</html>
Javascript
//mergePDF.js:
import PDFMerger from 'pdf-merger-js';
const merger = new PDFMerger();
const mergePDFs = async (pdfFiles) => {
for (const pdfFile of pdfFiles) {
await merger.add(pdfFile);
}
let d = new Date().getTime();
let mergedFileName = `merged_${d}.pdf`;
await merger.save(`public/${mergedFileName}`);
return mergedFileName;
}
export default mergePDFs;
Javascript
//server.js
import express from 'express';
import path from 'path';
import { fileURLToPath } from 'url';
import { dirname } from 'path';
import multer from 'multer';
import mergePDFs from './mergePDF.js';
const upload = multer({ dest: 'uploads/' });
const app = express();
const port = 3000;
const __filename = fileURLToPath(import.meta.url);
const __dirname = dirname(__filename);
app.use('/static', express.static('public'));
app.get('/', (req, res) => {
res.sendFile(path.join(__dirname, "index.html"));
})
app.post('/merge', upload.array('pdfs', 10), async (req, res, next) => {
if (!req.files || req.files.length < 2) {
return res.status(400).send('Please upload at least 2 PDF files');
}
let mergedFilePaths = [];
for (let file of req.files) {
mergedFilePaths.push(path.join(__dirname, file.path));
}
let mergedFileName = await mergePDFs(mergedFilePaths);
res.redirect(`http://localhost:${port}/static/${mergedFileName}`);
})
app.listen(port, () => {
console.log(`Example app listening on port http://localhost:${port}`);
})
Step to Run the App:
npm start
Output:
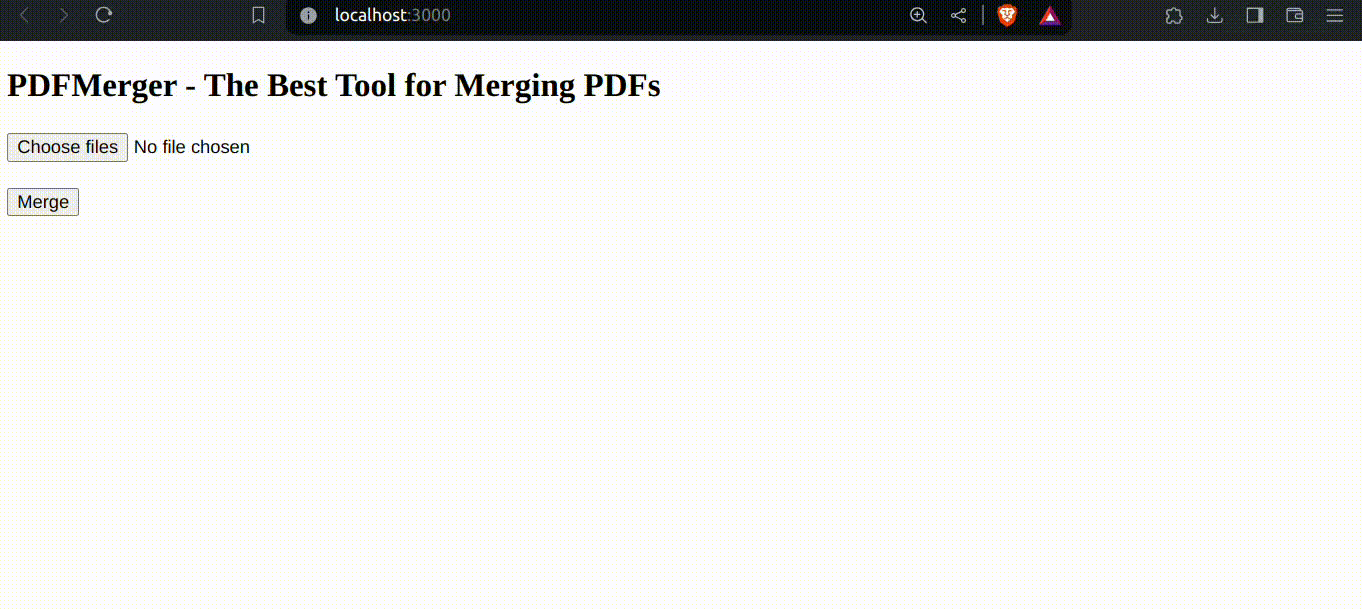
Execution of the application
Share your thoughts in the comments
Please Login to comment...