Create a Wikipedia Search using HTML CSS and JavaScript
Last Updated :
05 Oct, 2023
In this article, we’re going to create an application, for searching Wikipedia. Using HTML, CSS, and JavaScript, users will be able to search for articles on Wikipedia and view the results in a user interface. When the user inputs the search text into the textbox, the search result for the same will be shown to the user, and the user can click on the result and he will be redirected to the Wikipedia page for the same.
Prerequisites
Final Output
.png)
Preview of Final Output
Approach
We’ll design a user interface that includes an input field for search queries and an area to display the search results. Once a user enters their query and clicks the “Search” button, our JavaScript code will send a request to the Wikipedia API to fetch articles. The retrieved results will then be shown on the web page.
- Start by creating an HTML file that includes an input field, and a container, for displaying the results and the required structure.
- Use CSS to style the interface and make it visually appealing.
- Implement JavaScript code to listen for when the “Enter” key is pressed.
- When the key press event occurs, construct a URL for accessing the Wikipedia API with the specified search query.
- Utilize AJAX to fetch JSON data from the API.
- Remove any search results. Dynamically generate HTML to display the new search results.
Project Structure
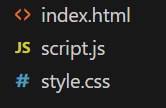
Project Structure
Example: This example describes the basic implementation of the Wikipedia Search using HTML, CSS, and JavaScript.
HTML
<!DOCTYPE html>
< html >
< head >
< link rel = "stylesheet"
type = "text/css"
href = "styles.css" />
< script src =
</ script >
< script src = "app.js"
type = "text/javascript" >
</ script >
</ head >
< body >
< h1 id = "top" >
GeeksforGeeks
</ h1 >
< h1 >
Wikipedia Viewer
</ h1 >
< div class = "container-new" >
< div id = "searchBox" >
< input type = "text"
id = "search"
autocomplete = "off"
placeholder = "Search here" />
</ div >
</ div >
</ body >
</ html >
|
CSS
body {
text-align : center ;
}
# top {
color : green ;
}
h 1 {
margin-top : 30px ;
}
p {
background-color : rgb ( 153 ,
225 ,
155 );
}
#randomPage {
color : white ;
}
input[type= "text" ] {
border-radius: 15px ;
text-align : center ;
height : 50px ;
width : 600px ;
-webkit-transition: width 0.4 s ease-in-out;
transition: width 0.4 s ease-in-out;
}
input[type= "text" ]:focus {
width : 100% ;
}
.searchTitle {
font-weight : bold ;
margin-bottom : 3px ;
}
.searchResult {
background-color : rgb ( 153 ,
225 ,
155 );
color : rgb ( 18 , 17 , 17 );
height : 60px ;
padding : 10px ;
margin : 2px ;
}
.searchResult:hover {
cursor : pointer ;
background-color : white ;
color : black ;
}
|
Javascript
$(document).ready( function () {
$(document).keypress( function (e) {
if (e.which == 13) {
let webLink =
"...api.php?action=query&list=search&srsearch=" +
document.getElementById(
"search"
).value +
"&utf8=&format=json" ;
$.ajax({
url: webLink,
dataType: "jsonp" ,
success: function (
data
) {
$( "div" ).remove(
".searchResult"
);
for (
i = 0;
i <
data.query
.search
.length;
i++
) {
let titleForResult =
data.query
.search[
i
].title;
let snippetForResult =
data.query
.search[
i
].snippet;
$(
"#searchBox"
).append(
titleForResult +
`" target= "_blank"
style= "text-decoration:none" >
<div class= "searchResult" >
<span class= "searchTitle" >` +
titleForResult +
"</span><span><br />" +
snippetForResult +
"</span></div></a>"
);
}
},
});
}
});
});
|
Output:
Share your thoughts in the comments
Please Login to comment...