Literals In C++
Last Updated :
02 Jan, 2024
In C++ programming language, we use literals to represent fixed values. C++ supports various types of literals including integer literals, floating-point literals, character literals, and string literals. In this article, we will discuss all the necessary information on C++ literals and their usage.
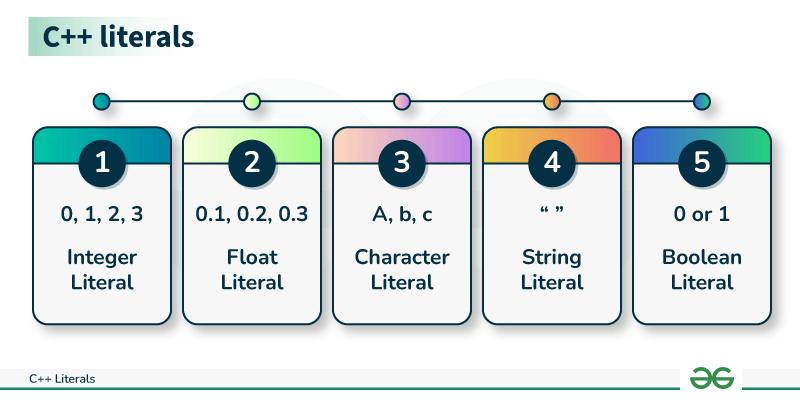
What are Literals in C++?
Literals are fundamental elements used to represent constant values used in C++ programming language. These constants can include numbers, characters, strings, and more. Understanding and using the literals is essential in C++ for data assignment, calculations, and data representation. They are generally present as the right operand in the assignment operation.
Types of Literals in C++
There are five types of literals in C++ which are:
- Integer Literals
- Floating Point Literals
- Character Literals
- String Literals
- Boolean Literals
1. Integer Literals
Integer literals in C++ are constants that represent whole numbers without the fractional or decimal part. They can be positive or negative and can have different bases such as decimal, octal, or hexadecimal.
Example
int integerLiteral = 42;
Implementation
C++
#include <iostream>
using namespace std;
int main()
{
int age = 35;
cout << "Age: " << age << endl;
return 0;
}
|
In C++, integer literals can be categorized into various types based on their bases.
1. Decimal Literal (No Prefix)
The decimal literal represents an integer in base 10 without any prefix.
Example
int decimal = 42;
2. Octal Literal (Prefix: 0)
The octal literal represents an integer in base 8 and is preceded by the 0 prefix.
Example
int octal = 052; // 42 in decimal
3. Hexadecimal Literal (Prefix: 0x or 0X)
The hexadecimal literal represents an integer in the base 16 and is preceded by either 0x or 0X prefix.
Example
int hexadecimal = 0x2A; // 42 in decimal
4. Binary Literal (Suffix: b or B)
The binary literal represents an integer in the base 2 and can have a suffix of b or B.
Example
int binary = 0b101010; // 42 in decimal
5. Long Integer Literal (Suffix: l or L)
The long integer literal can be suffixed with the l or L to indicate that it is of type long int.
Example
long int longInteger = 42L;
6. Unsigned Integer Literal (Suffix: u or U)
The unsigned integer literal can be suffixed with the u or U to indicate that it is of type unsigned int.
Example
unsigned int unsignedInt = 42U;
7. Long Long Integer Literal (Suffix: ll or LL)
The long long integer literal can be suffixed with the ll or LL to indicate that it is of type long long int.
Example
long long int longLongInteger = 42LL;
2. Floating-Point Literals
The Floating-point literals in C++ are constants that represent numbers with the fractional or decimal part. These can be either single-precision or double-precision values.
Like integer literals, the floating point literals can also be defined as double or float by using suffix (no suffix) and f respectively. By default, all the fractional numbers are considered to be of type double by C++ compiler.
Example
double floatLiteral = 3.14;
Implementation
C++
#include <iostream>
using namespace std;
int main()
{
float e = 2.7f;
double pi = 3.14;
long double g = 9.8L;
cout << "The Value of pi: " << pi << endl;
cout << "The value of e: " << e << endl;
cout << "The value of g: " << g << endl;
return 0;
}
|
Output
The Value of pi: 3.14
The value of e: 2.7
The value of g: 9.8
3. Character Literals
The Character literals in C++ are constants that represent individual characters or escape sequences. They are enclosed in single quotes and you can use them to represent the single character.
Example
char charLiteral = 'A';
Implementation
C++
#include <iostream>
using namespace std;
int main()
{
char grade = 'A' ;
cout << "Grade: " << grade << endl;
return 0;
}
|
4. String Literals
The String literals in C++ are constants that represent sequences of the characters. They are enclosed in double quotes and you can use them to represent text or character sequences.
Example
const char* stringLiteral = "Hello, World!";
Implementation
C++
#include <iostream>
using namespace std;
int main()
{
const char * message = "Hello, GeeksforGeeks!" ;
cout << "Message: " << message << endl;
return 0;
}
|
Output
Message: Hello, GeeksforGeeks!
Note: The string literals are the only literals that are not rvalues.
5. Boolean Literals
The Boolean literals represent the truth values and have only two possible values: true and false. These values are used in the Boolean expressions and logic operations.
Example
C++
#include <iostream>
using namespace std;
int main()
{
bool isTrue = true ;
bool isFalse = false ;
if (isTrue) {
cout << "isTrue is true" << endl;
}
else {
cout << "isTrue is false" << endl;
}
if (isFalse) {
cout << "isFalse is true" << endl;
}
else {
cout << "isFalse is false" << endl;
}
return 0;
}
|
Output
isTrue is true
isFalse is false
Share your thoughts in the comments
Please Login to comment...