Converting A Blob Into A Bytearray In Python Flask
Last Updated :
26 Mar, 2024
In web development, dealing with binary data, such as images or files, is a common requirement. Blobs (Binary Large Objects) are a representation of binary data, and at times, you may need to convert a blob into a more manageable data structure, like a Python bytearray. This article will guide you through the process of converting a blob into a bytearray in the context of a Python Flask application.
sample.xml
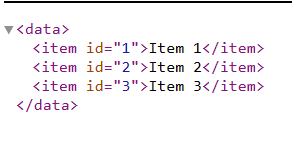
Converting Blob to Bytearray in Flask
Below are the step-by-step procedure to convert blob to bytearray in Python Flask:
Create a Virtual Environment
We will create the virtual environment and activate it by using the following commands:
python -m venv venv
venv\Scripts\activate
Now, We will install the following libraries before starting:
pip install flask
File Structure
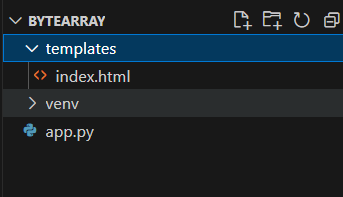
Set Up a Flask Application
Create a file named app.py in the project directory and initialize a Flask application.
Python3
from flask import Flask, render_template, request
import io
import base64
app = Flask(__name__)
@app.route('/')
def index():
return render_template('index.html')
@app.route('/upload', methods=['POST'])
def upload():
blob_file = request.files['blob_file']
if blob_file:
blob_data = blob_file.read()
byte_array = bytearray(blob_data) # Convert Blob to Bytearray
encoded_data = base64.b64encode(bytes(byte_array)).decode(
'utf-8') # Encode bytearray to base64
return (encoded_data)
return "No file uploaded."
if __name__ == '__main__':
app.run(debug=True)
Create HTML Template
Inside the templates directory, create a file named index.html.
HTML
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Upload Blob</title>
</head>
<body>
<form action="/upload" method="post" enctype="multipart/form-data">
<input type="file" name="blob_file" accept=".txt,.pdf,.jpg,.png,.gif">
<button type="submit">Upload</button>
</form>
</body>
</html>
Output
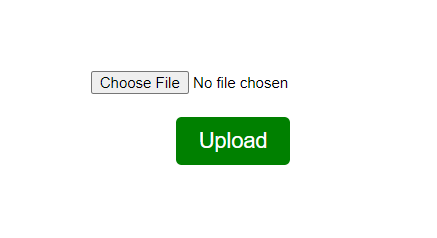
Run the Program
Save all the changes and run the Flask application:
python app.py
Visit http://127.0.0.1:5000/ in your web browser. You should see a file upload form. Choose a file and click the “Upload” button. The application will convert the uploaded Blob into a Bytearray.
Output:
-660.png)
Conclusion
Converting a blob into a bytearray in a Python Flask application is a straightforward process. By fetching the blob data from your data source, converting it using Python’s bytearray constructor, and integrating it into your Flask application, you can effectively manage binary data within your web application. Adjust the provided code snippets based on your specific use case and database setup.
Share your thoughts in the comments
Please Login to comment...