Code for Fork System Call in OS
Last Updated :
13 Oct, 2023
Fork is a system call in the Operating System used to create a copy of the process, the copied process created is known as the child process. The main process or the process from which the child process is created is known as the parent process. The parent and child processes are in separate address spaces in fork(). A Child process will have its Process ID different from the parent’s ID.
Primary Terminologies related to Fork System Call in OS
Process: The task or job performed by the computer. Example: running a program.
- System call: A system call is a way to shift between user mode and kernel node to access the Functionalities of Operating Systems.
- Child process: The child process is the new process that is created by the parent process. It is essentially a copy of the parent process, and A system inherits many of its attributes, such as code, data, and open files.
- Parent process: The parent process is the original process that initiates the creation of a new process. It serves as the starting point for the new process
- Process ID (PID): Process ID is a special identification that OS assigns to every process. It returns an integer value.
We use fork() one time in a parent (P) then a child process is created (C1) and there is also a parent process (P) present. Similarly, if we write fork again, here C1 becomes the parent and creates a new child process C2 and there is a parent process (C1) available. P also creates a child process (C3) and P is also present (P).
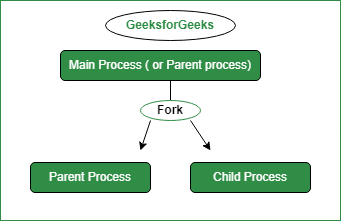
Example 1: Fork system call in OS
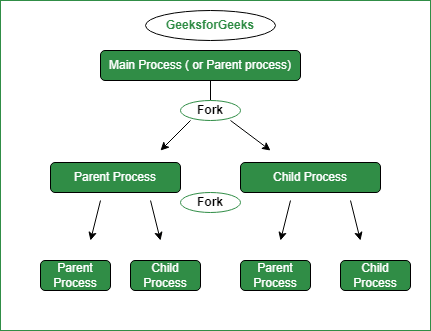
Example 2: Fork system call in OS
By observing, we can conclude:
- Total no. of child process generated will be: 2^n – 1.
- Total no of processes executed: 2^n.
Here n is the number of times forks called.
Remember that child processes return 0, while parent processes return a positive value (probably +1) at the fork call.
Examples of Fork System call in OS
1. Simple Fork:
C
#include <stdio.h>
#include <unistd.h>
int main() {
pid_t child_pid;
child_pid = fork();
if (child_pid == 0) {
printf ( "Child process\n" );
} else if (child_pid > 0) {
printf ( "Parent process\n" );
} else {
perror ( "Fork failed" );
}
return 0;
}
|
Output:
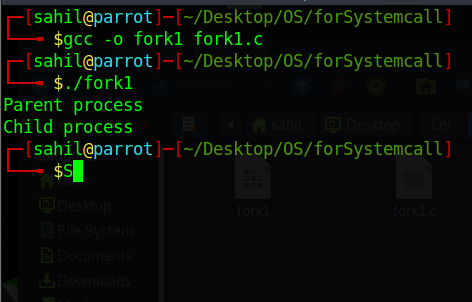
Fork example in linux OS
2. Parent and Child Processes Printing with PIDs:
C
#include <stdio.h>
#include <unistd.h>
int main() {
pid_t child_pid;
child_pid = fork();
if (child_pid == 0) {
printf ( "Child process: PID=%d\n" , getpid());
} else if (child_pid > 0) {
printf ( "Parent process: PID=%d, Child PID=%d\n" , getpid(), child_pid);
} else {
perror ( "Fork failed" );
}
return 0;
}
|
Output:
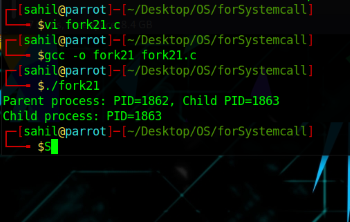
Fork example in linux OS
3. Forking Multiple Child Processes:
C
#include <stdio.h>
#include <unistd.h>
int main() {
int i;
for (i = 0; i < 3; i++) {
pid_t child_pid = fork();
if (child_pid == 0) {
printf ( "Child process %d: PID=%d\n" , i + 1, getpid());
break ;
} else if (child_pid < 0) {
perror ( "Fork failed" );
}
}
if (getpid() != 1) {
printf ( "Parent process: PID=%d\n" , getpid());
}
return 0;
}
|
Output:
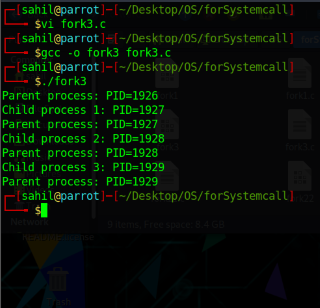
Fork example in linux OS
Conclusion
fork() system call is the fundamental tool that allows processes to duplicate themselves. It makes a copy of process in a separate memory space, it provides both child and parents a different process ID (PIDs) for identification. The uses of fork are multitasking, parallel processing and complex process structure.
Frequently Asked Questions (FAQ’s)
Question 1. How does ‘fork()’ work?
Answer:
When a process calls fork, it creates duplicate of the process. Both processes continue executing from the same point but have their separate memory and resource.
Question 2. What’s the difference between parent and child processes created by ‘fork()’ ?
Answer:
They have different Process IDs (PIDs), and changes made in one process don’t affect the other process.
Question 3. What are some common uses of ‘fork()’?
Answer:
Common uses of fork() are : Creating parallel processing, implementing multitasking and building complex process hierarchies.
Question 4. Can child processes also call ‘fork()’?
Answer:
Yes, child processes can call fork() which will create additional child processes.
Question 5. What are the values returned by ‘fork()’ and what they indicate?
Answer:
Fork can return 3 values 0, positive (maybe +1) or -1. In case of child process it will return 0 and for parent, it will return positive value. Otherwise if fork returns -1, it indicates failure typically due to resource limitations.
Question 6. The child process can run independently from the parent?
Answer:
Yes, the child process can run independently from the parent intentionally or unintentionally. Those processes that are runs even after their parent process has terminated or finished are known as Orphan processes.
Share your thoughts in the comments
Please Login to comment...