Closeable Interface in Java
Last Updated :
04 Jan, 2021
A Closeable is a source or destination of the data that needs to be closed. The close() method is invoked when we need to release resources that are being held by objects such as open files. It is one of the important interfaces to stream classes. Closeable Interface was introduced in JDK 5 and is defined in java.io. From JDK 7+, we are supposed to use AutoCloseable interface. The closeable interface is an older interface that was introduced to preserve backward compatibility.
The Hierarchy of Closeable interface
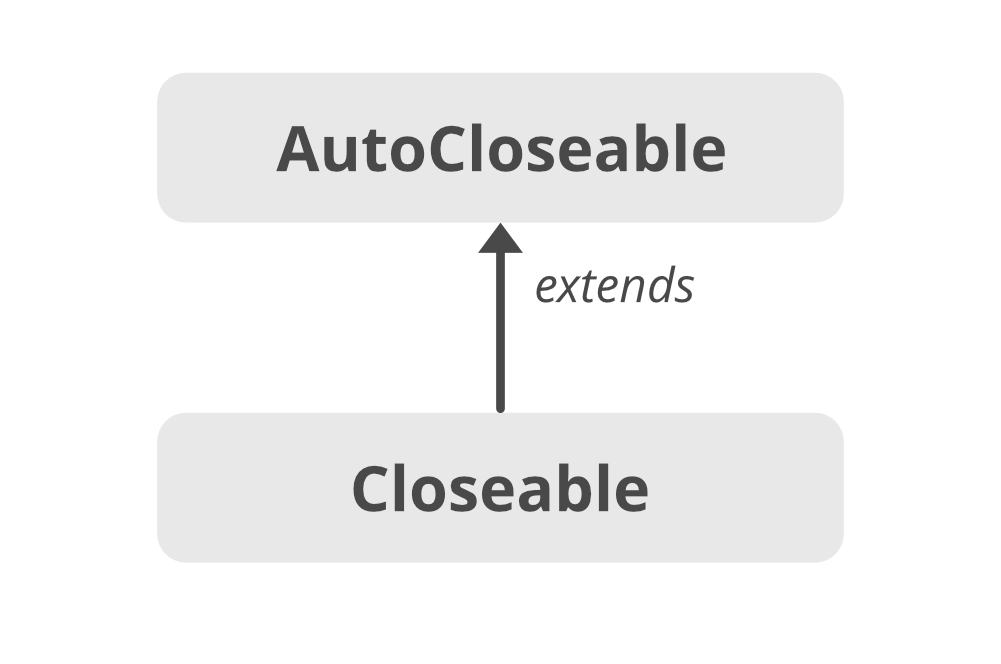
The Closeable interface extends AutoCloseable interface therefore any class that implements Closeable also implements AutoCloseable.
Declaration
public interface Closeable extends AutoCloseable
{
public void close() throws IOException;
}
Implementing the Closeable interface
import java.io.Closeable;
import java.io.IOException;
public class MyCustomCloseableClass implements Closeable {
@Override
public void close() throws IOException {
// close resource
System.out.println("Closing");
}
}
close() method of Closeable interface
The close() method is invoked to release resources that the object is holding. If the stream is already closed then invoking the close method does not have any effects.
Syntax
public void close() throws IOException
Note: Closeable is idempotent, which means calling the close() method more than once has no side effects.
Limitations of Closeable Interface
Closeable throws only IOException and it cannot be changed without breaking legacy code. Therefore, AutoCloseable was introduced as it can throw an Exception.
SuperInterface of Closeable:
SubInterfaces of Closeable:
- AsynchronousByteChannel
- AsynchronousChannel
- ByteChannel
- Channel
- ImageInputStream
- ImageOutputStream
- MulticastChannel
Implementing Classes:
- AbstractSelectableChannel
- AbstractSelector
- BufferedReader
- BufferedWriter
- BufferedInputStream
- BufferedOutputStream
- CheckedInputStream
- CheckedOutputStream
Closeable vs AutoCloseable
- Closeable was introduced with JDK 5 whereas AutoCloseable was introduced with JDK 7+.
- Closeable extends AutoCloseable and Closeable is mainly directed to IO streams.
- Closeable extends IOException whereas AutoCloseable extends Exception.
- Closeable interface is idempotent (calling close() method more than once does not have any side effects) whereas AutoCloseable does not provide this feature.
- AutoCloseable was specially introduced to work with try-with-resources statements. Since Closeable implements AutoCloseable, therefore any class that implements Closeable also implements AutoCloseable interface and can use the try-with resources to close the files.
try(FileInputStream fin = new FileInputStream(input)) {
// Some code here
}
Usage of try-with Block with Closeable
Since Closeable inherits the properties of AutoCloseable interface, therefore the class implementing Closeable can also use try-with-resources block. Multiple resources can be used inside a try-with-resources block and have them all automatically closed. In this case, the resources will be closed in the reverse order in which they were created inside the brackets.
Java
import java.io.*;
class Resource {
public static void main(String s[])
{
try (Demo d = new Demo(); Demo1 d1 = new Demo1()) {
int x = 10 / 0 ;
d.show();
d1.show1();
}
catch (ArithmeticException e) {
System.out.println(e);
}
}
}
class Demo implements Closeable {
void show() { System.out.println( "inside show" ); }
public void close()
{
System.out.println( "close from demo" );
}
}
class Demo1 implements Closeable {
void show1() { System.out.println( "inside show1" ); }
public void close()
{
System.out.println( "close from demo1" );
}
}
|
Output
close from demo1
close from demo
java.lang.ArithmeticException: / by zero
Methods of Closeable interface
Method
|
Description
|
close() |
Closes this stream and releases any system resources associated with it. |
Share your thoughts in the comments
Please Login to comment...