atoi() Function in C
Last Updated :
16 Dec, 2023
In C, atoi stands for ASCII To Integer. The atoi() is a library function in C that converts the numbers in string form to their integer value. To put it simply, the atoi() function accepts a string (which represents an integer) as a parameter and yields an integer value in return.
C atoi() function is defined inside <stdlib.h> header file.
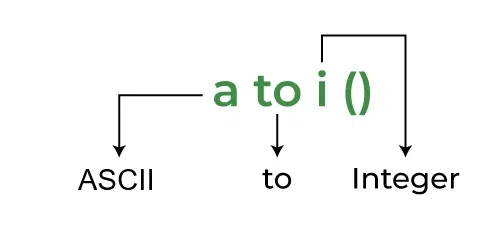
Syntax of atoi() Function
int atoi(const char *strg);
Parameters
- strg: The string that is to be converted to an integer.
Note: The atoi function takes in a string as a constant (Unchangeable) and gives back the converted integer without modifying the original string.
Return Value
The atoi() function returns the following value:
- An equivalent integer value by interpreting the input string as a number only if the input string str is Valid.
- If the conversion is not valid, the function returns 0.
Note: In the case of an overflow the returned value is undefined.
Examples of atoi() in C
Example 1:
This example shows how to convert numbers in String form to their Integer values.
C
#include <stdio.h>
#include <stdlib.h>
int main()
{
char strToConvert[] = "908475966" ;
int ConvertedStr = atoi (strToConvert);
printf ( "String to be Converted: %s\n" , strToConvert);
printf ( "Converted to Integer: %d\n" , ConvertedStr);
return 0;
}
|
Output
String to be Converted: 908475966
Converted to Integer: 908475966
Explanation: The string “99898989” is converted to the corresponding integer value, and both the original string and the returned integer value are printed.
Example 2:
This example shows if the input string contains non-numeric characters so the function returns 0.
C
#include <stdio.h>
#include <stdlib.h>
int main()
{
char strToConvert[] = "geeksforgeeks" ;
int ConvertedStr = atoi (strToConvert);
printf ( "String to be Converted: %s\n" , strToConvert);
printf ( "Converted to Integer: %d\n" , ConvertedStr);
return 0;
}
|
Output
String to be Converted: geeksforgeeks
Converted to Integer: 0
Explanation: The string “geeksforgeeks” is attempted to be converted to an integer but the string contains non-numeric characters so the function returns 0.
How to Implement Your Own atoi() in C?
The equivalent of the atoi() function is easy to implement. One of the possible method to implement is shown below:
Approach
- Initialize res to 0.
- Iterate through each character of the string and update the res by multiplying it by 10 and keep adding the numeric value of the current digit. (res = res*10+(strg[i]-‘0’))
- Continue until the end of the string.
- return res.
Implementation of atoi() in C
C
#include <stdio.h>
int atoi_Conversion( const char * strg)
{
int res = 0;
int i = 0;
while (strg[i] != '\0' ) {
res = res * 10 + (strg[i] - '0' );
i++;
}
return res;
}
int main()
{
const char strg[] = "12345" ;
int value = atoi_Conversion(strg);
printf ( "String to be Converted: %s\n" , strg);
printf ( "Converted to Integer: %d\n" , value);
return 0;
}
|
Output
String to be Converted: 12345
Converted to Integer: 12345
To learn more about different approaches, refer to the article – Write your own atoi()
Properties of atoi() Function
The atoi() function have different output for different input types. The following examples shown the behaviors of the atoi() function for different input values.
1. The atoi() function does not recognize decimal points or exponents.
C
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main()
{
int res_val;
char inp_str[30];
strcpy (inp_str, "12.56" );
res_val = atoi (inp_str);
printf ( "Input String = %s\nResulting Integer = %d\n" ,
inp_str, res_val);
return 0;
}
|
Output
Input String = 12.56
Resulting Integer = 12
2. Passing invalid string to atoi() returns 0.
C
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main()
{
int res_val;
char inp_str[30];
strcpy (inp_str, "geeksforgeeks" );
res_val = atoi (inp_str);
printf ( "Input String = %s\nResulting Integer = %d\n" ,
inp_str, res_val);
return 0;
}
|
Output
Input String = geeksforgeeks
Resulting Integer = 0
3. Passing partially valid string results in conversion of only integer part. (If non-numerical values are at the beginning then it returns 0)
C
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main()
{
int res_val;
char inp_str[30];
strcpy (inp_str, "1234adsnds" );
res_val = atoi (inp_str);
printf ( "Input String = %s\nResulting Integer = %d\n" ,
inp_str, res_val);
return 0;
}
|
Output
Input String = 1234adsnds
Resulting Integer = 1234
4. Passing string beginning with the character ‘+’ to atoi() then ‘+’ is ignored and only integer value is returned.
C
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main()
{
int res_val;
char inp_str[30];
strcpy (inp_str, "+23234" );
res_val = atoi (inp_str);
printf ( "Input String = %s\nResulting Integer = %d\n" ,
inp_str, res_val);
return 0;
}
|
Output
Input String = +23234
Resulting Integer = 23234
5. Passing string beginning with the character ‘-‘ to atoi() then the result includes ‘-‘ at the beginning.
C
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
int main()
{
int res_val;
char inp_str[30];
strcpy (inp_str, "-23234" );
res_val = atoi (inp_str);
printf ( "Input String = %s\nResulting Integer = %d\n" ,
inp_str, res_val);
return 0;
}
|
Output
Input String = -23234
Resulting Integer = -23234
Conclusion
- atoi() function converts numeric string to its corresponding integer value.
- To use the atoi() function in your C program include the stdlib.h header file.
- atoi() accepts only one string parameter as input.
- If an invalid string is passed as parameter, the function returns 0.
Share your thoughts in the comments
Please Login to comment...