Bitmask in C++
Last Updated :
11 Dec, 2023
In the world of programming, where precision and efficiency are of great importance, bitmasking is a powerful technique that utilizes the manipulation of bits. C++ also offers a platform where programmers can efficiently represent data and unlock unparalleled computational capabilities through bit masking. In this article, we will discuss the bitmasking, and bitmasking techniques in C++.
What is a bit?
A bit is the smallest unit of data. We know that a computer system only understands binary language which consists of 0s and 1s. These 0s and 1s single-handedly are known as – ‘BIT’.
Bitmasking in C++
Bitmasking is a technique used in programming to perform operations more efficiently on binary data. Bitmasking is a frequently employed technique in algorithms to enhance performance in terms of time complexity, utilizing bitwise operators for efficient operations at the bit level.
Bitmasking in C++ involves manipulating individual bits of a number to achieve the desired output. It is achieved by generating a bit mask and is used very often for the following operations:
- Bit Toggle: If a bit is set to 0, it can be toggled to 1 and vice-versa.
- Bit Setting: If a bit is set to 0 then it’s called ‘bit is NOT set’. We can set it by performing a toggle operation and change it to 1. This is known as bit setting.
- Bit Clearing: If a bit is set to 1 then it’s called a ‘SET-BIT’. We can change it to 0 by performing a toggle operation this is called a – ‘Bit-clearing’ operation.
- Checking specific bit is on or off: A bit is said to be on if it’s 1 and off if it’s 0. For example, an integer can contain multiple bits and we can check if, in that integer, a specific bit is set or not by utilizing bitwise operators.
What is a Bit Mask?
A bit mask is the fundamental technique to achieve bit masking. It is basically a binary pattern used to perform various bit-level operations like set, clear, toggle or checking if a bit is set or not.
The bit mask is created in the following way:
Assuming that we have the set of numbers, whose binary representation has 8 bits in it, we will use this as a reference in this article.
We know that 1 has only a single bit set to 1 which is the right-most bit otherwise known as the least significant bit. The remaining 7 bits are set to 0. So it would look something like this :
Shifting the set bit (the LSB) to 3 places to the left using expression (1<<3) would look something like this:
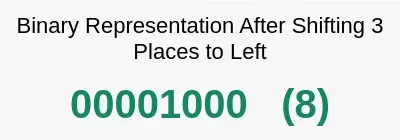
Shifting 1 to 3 places
After shifting, the set bit to 3 places, it becomes 8. Similarly, we can do this for any place. We’ll use this shifting property in following bitwise techniques to create a bit mask and then perform various bitwise operations with it.
Performing Bitmasking in C++
Bitmasking is done by creating a bit mask for the operation that we need to perform. This bit mask will then be followed by a bitwise operation to achieve the desired output.
Bitmasking is done by putting a mask (hiding some unnecessary bits based on some criteria) and setting or clearing the remaining bits. This masking of certain bits helps us in performing the desired operation more efficiently thereby improving our algorithm’s performance and optimizing memory. Before diving into examples for a better understanding, let us go through the bitwise operators which are fundamental for understanding the examples.
Following are the bitwise operators in C++ used to perform bitmasking in C++ :
- Bitwise AND (&) – return true only if both the bits are set
- Bitwise OR (|) – returns true if either of the bits is set.
- Bitwise XOR (^) – returns true if two bits are different.
- Bitwise NOT (~) – negates the bit.
- Bitwise Left Shift (<<) – Shifts all the bits to the left by 1 place.
- Bitwise Right Shift (>>) – Shifts all the bits to the right by 1 place.
Let’s look at common bitwise operations and their examples.
Bitmasking Operations in C++
1. Setting a Specific Bit
Setting a specific bit basically means changing it from 0 to 1. It can be done by utilizing the Bitwise OR because of its property to give 1 if either of the bits is set to 1 and the bitwise left shift operator.
We will shift the LSB bit of 1 to the specified position that we want to set and then perform a bitwise OR Operation.
Syntax
integer | (1 << bit_position_to_be_set)
Here, the bit position to be set will be the place of the bit that we want to change to 1.
Example
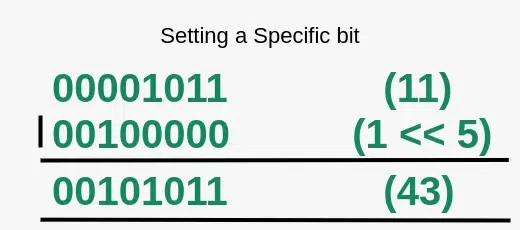
Implementation
C++
#include <iostream>
using namespace std;
int main()
{
int x = 11;
x = x | 1 << 5;
cout << "Result after setting the fifth bit: " << x ;
return 0;
}
|
Output
Result after setting the fifth bit: 43
2. Clearing a Bit
Clearing a bit means we set it to 0 if it is 1 without touching or affecting any other bits. This is done by using Bitwise AND and the negation operator (Bitwise NOT). The Bitwise NOT flips all the bits that are 1 to 0 and 0 to 1. This property of the bitwise NOT helps us in clearing a set bit.
Syntax
integer & ~(1 << bit_position_to_clear)
Example
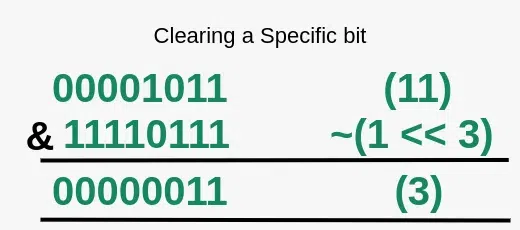
Implementation
C++
#include <iostream>
using namespace std;
int main()
{
int x = 11;
x = x & ~(1 << 3);
cout << "Result after clearing the 3rd bit: " << x;
return 0;
}
|
Output
Result after clearing the 3rd bit: 3
3. Toggle a Bit
In this operation, we flip a bit. If it’s set to 1 we make it 0 and if it’s set to 0 then we flip it to 1. This is easily achievable by the Bitwise XOR operator (^) and the left shift (<<). We will utilize the property of the XOR operator to flip the bits if the bits of 2 different numbers are not the same.
The same approach is used that is, by shifting 1 to a specific position which we want to flip.
Syntax
Integer ^ (1 << bit_position_to_toggle)
Example
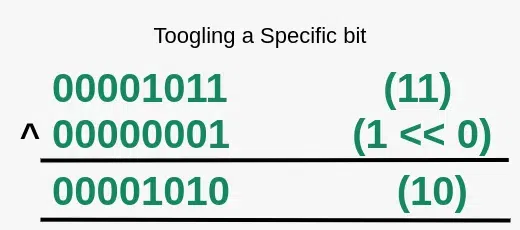
Implementation
C++
#include <iostream>
using namespace std;
int main()
{
int x = 11;
x = x ^ 1 << 0;
cout << "Result after toggling the zeroth bit: " << x;
return 0;
}
|
Output
Result after toggling the zeroth bit: 10
4. Check if a Bit is Set or not
In this operation, we check if a bit at a specific position is set or not. This is done by using the bitwise AND (&) and the Left shift operator. We basically left shift the set bit of 1 to the specified position for which we want to perform a check and then perform a bitwise AND Operation.
If the bit is set then the answer will be – 2(bit_position) For example, if the bit position is 3, then the answer will be 23 = 8. Else if the bit is 0 (not set) then the answer will be 0.
Syntax
Integer & (1 << bit_position_to_check)
Example
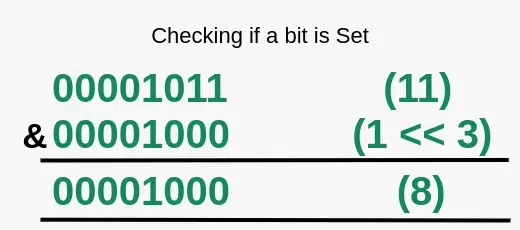
Implementation
C++
#include <iostream>
using namespace std;
int main()
{
int x = 11;
if (x & (1 << 3)) {
cout << "Third bit is set\n" ;
}
else {
cout << "Third bit is not set\n" ;
}
return 0;
}
|
Conclusion
Bit masks in C++ are a great way to manipulate the bits of the number and alter their actual value. Performing operations at the bit level is efficient and is done to optimize the algorithm.
FAQs on Bitmasking in C++
Q1. What is Bitmasking and basic operations using a bitmask?
Answer:
Bit masking is a technique to optimize the algorithm by using a bit mask to manipulate the bits of an integer or some binary data. Using bit masking we can perform some basic manipulations on the bits of a number:
- Setting a Bit
- Clearing a Bit
- Toggling a Bit
- Checking if a Bit is Set or Not
Q2. What are the applications of Bit Masking?
Answer:
Bit masking have following applications :
- Compression: Bit masking is highly useful in case of compression that allows reducing the size of data. Ex : JPEG image stores the compression parameters as bit mask.
- Filters on Images: When we apply filters to the images we’re uploading, what’s happening under the hood is pixels of our original image are merging with some special bits. We can also call this procedure a mix of bit masking and bit merging.
- Cryptograph: It is used in cryptography to add/remove redundant bits and encrypt and decrypt the data.
- Optimization: It is used to optimize the algorithm making them more time and space efficient.
Share your thoughts in the comments
Please Login to comment...