Backbone.js Collections
Last Updated :
25 Jul, 2022
A Backbone.js Collections are a group of related models. It is useful when the model is loading to the server or saving to the server. Collections also provide a helper function for performing computation against a list of models. Aside from their own events collections also proxy through all of the events that occur to the model within them, allowing you to listen in one place for any change that might happen to any model in the collection.
Now we will see the working of collections.
Syntax: We can create a collection with extending syntax as follows
let collection = Backbone.Collection.extend ( Properties , [ ClassProperties ] );
Properties:
- Properties: These are the properties that are assigned to the instance of collection when initiated.
- Class-Properties: These are the properties that are attached to the collection’s constructor function.
Example 1: In this example, we will create a collection and pass the argument as a list of models creating the array. The following code shows how we can add a list of models at the creation of the Collection.
HTML
<!DOCTYPE html>
< html >
< head >
< meta charset = "UTF-8" >
< title >Backbone.js Collections</ title >
< script src =
< script src =
type = "text/javascript" ></ script >
< script src =
type = "text/javascript" ></ script >
</ head >
< body >
< script type = "text/javascript" >
var car = Backbone.Model.extend();
var collection = Backbone.Collection.extend({
model: car,
});
let l = [new car({ Brand: "BMW", color: "blue" }),
new car({ Brand: "Audi", color: " red" })]
var Coll_car = new collection(l);
console.log(JSON.stringify(Coll_car));
</ script >
</ body >
</ html >
|
Output:
Example 2: In this example, we will create a collection and add the model to the collection with the add method.
HTML
<!DOCTYPE html>
< html >
< head >
< meta charset = "UTF-8" >
< title >Backbone.js Collections</ title >
type = "text/javascript" ></ script >
< script src =
type = "text/javascript" ></ script >
< script src =
type = "text/javascript" ></ script >
</ head >
< body >
< script type = "text/javascript" >
var car = Backbone.Model.extend();
var collection = Backbone.Collection.extend({
model: car,
});
var Coll_car = new collection();
Coll_car.add(new car({ Brand: "BMW", color: "blue" }))
Coll_car.add(new car({ Brand: "Audi", color: " red" }))
Coll_car.add(new car({ Brand: "Verna", color: "black" }))
Coll_car.add(new car({ Brand: "Tata nano", color: "green" }))
console.log(Coll_car);
</ script >
</ body >
</ html >z
|
Output:
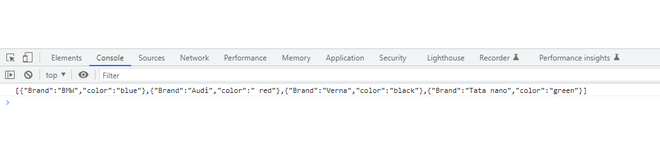
ADD MODEL WITH ADD METHOD
Reference: https://backbonejs.org/#Collection
Share your thoughts in the comments
Please Login to comment...