Angular PrimeNG Table Stack Layout
Last Updated :
07 Sep, 2022
Angular PrimeNG is an open-source framework with a rich set of native Angular UI components that are used for great styling and this framework is used to make responsive websites with very much ease. In this article, we will see Angular PrimeNG Table Stack Layout.
The Table Component shows some data to the user in tabular form. In the stack layout of the table, when the width of the table is less than a certain value (default is 960px), the columns of the table will be displayed as stacked. This feature can be enabled by setting the responsiveLayout property of the table to “stack” and by adding an element with the class “p-column-title” to all the body cells.
Syntax:
<p-table [value]="persons" responsiveLayout="stack">
<ng-template pTemplate="header">
<tr>
<th>Name</th>
<th>Age</th>
...
</tr>
</ng-template>
<ng-template pTemplate="body" let-person>
<tr>
<td><span class="p-column-title"> ... </span>
{{ person.name }}
</td>
<td> <span class="p-column-title"> ... </span>
{{ person.age }}
</td>
...
</tr>
</ng-template>
</p-table>
Creating Angular application and Installing the Modules:
Step 1: Create an Angular application using the following command.
ng new myapp
Step 2: After creating your project folder i.e. myapp, move to it using the following command.
cd myapp
Step 3: Install PrimeNG in your given directory.
npm install primeng --save
npm install primeicons --save
Project Structure: After completing the above steps the project structure will look like the following.
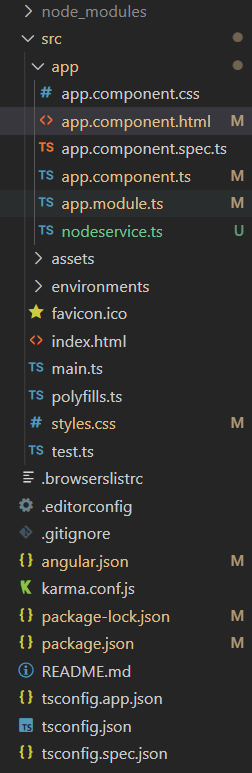
Project Structure
Example 1: This example shows the basic use of the stacked layout of the table component when the width of the table is less than 960px.
app.component.html
< div style = "text-align: center" >
< h2 style = "color: green" >GeeksforGeeks</ h2 >
< h4 >Angular PrimeNG Table Stacked Layout</ h4 >
< p-table [value]="persons" responsiveLayout = "stack" >
< ng-template pTemplate = "header" >
< tr >
< th >Name</ th >
< th >Age</ th >
< th >Profession</ th >
< th >Mobile</ th >
< th >Gender</ th >
</ tr >
</ ng-template >
< ng-template pTemplate = "body" let-person>
< tr >
< td >
< span class = "p-column-title" >
Name
</ span >
{{person.name}}
</ td >
< td >
< span class = "p-column-title" >
Age
</ span >
{{person.age}}
</ td >
< td >
< span class = "p-column-title" >
Profession
</ span >
{{person.profession}}
</ td >
< td >
< span class = "p-column-title" >
Mobile
</ span >
{{person.mobile}}
</ td >
< td >
< span class = "p-column-title" >
Gender
</ span >
{{person.gender}}
</ td >
</ tr >
</ ng-template >
< ng-template pTemplate = "emptymessage" >
< tr >
< td >No Persons Found.</ td >
</ tr >
</ ng-template >
</ p-table >
</ div >
|
app.component.ts
import { Component } from '@angular/core' ;
interface Person {
name: String,
age: Number,
profession: String,
mobile: String,
gender: String
}
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html' ,
})
export class AppComponent {
persons: Person[] = [
{
name: "James" ,
age: 21,
profession: "Doctor" ,
mobile: "8731******90" ,
gender: "Male"
},
{
name: "Sam" ,
age: 18,
profession: "Marketer" ,
mobile: "8989******98" ,
gender: "Male"
},
{
name: "Thomas" ,
age: 27,
profession: "Businessman" ,
mobile: "9645******11" ,
gender: "Male"
},
{
name: "Kate" ,
age: 24,
profession: "Teacher" ,
mobile: "9345******46" ,
gender: "Female"
},
{
name: "Kumar" ,
age: 17,
profession: "Student" ,
mobile: "7824******78" ,
gender: "Male"
},
{
name: "Ethan" ,
age: 22,
profession: "Delivery Boy" ,
mobile: "9090******90" ,
gender: "Male"
},
];
}
|
app.module.ts
import { NgModule } from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { BrowserAnimationsModule }
from '@angular/platform-browser/animations' ;
import { AppComponent } from './app.component' ;
import { TableModule } from 'primeng/table' ;
import { ButtonModule } from 'primeng/button' ;
@NgModule({
imports: [
BrowserModule,
BrowserAnimationsModule,
TableModule,
ButtonModule
],
declarations: [AppComponent],
bootstrap: [AppComponent],
})
export class AppModule { }
|
Output:
Example 2: This example illustrates the use of the table stack layout using a striped table.
app.component.html
< div style = "text-align: center" >
< h2 style = "color: green" >GeeksforGeeks</ h2 >
< h4 >Angular PrimeNG Table Stacked Layout</ h4 >
< p-table [value]="persons" responsiveLayout = "stack" >
< ng-template pTemplate = "header" >
< tr >
< th >Name</ th >
< th >Age</ th >
< th >Profession</ th >
< th >Mobile</ th >
< th >Gender</ th >
</ tr >
</ ng-template >
< ng-template pTemplate = "body" let-person>
< tr >
< td >
< span class = "p-column-title" >
Name
</ span >
{{person.name}}
</ td >
< td class = "even" >
< span class = "p-column-title" >
Age
</ span >
{{person.age}}
</ td >
< td >
< span class = "p-column-title" >
Profession
</ span >
{{person.profession}}
</ td >
< td class = "even" >
< span class = "p-column-title" >
Mobile
</ span >
{{person.mobile}}
</ td >
< td >
< span class = "p-column-title" >
Gender
</ span >
{{person.gender}}
</ td >
</ tr >
</ ng-template >
< ng-template pTemplate = "emptymessage" >
< tr >
< td >No Persons Found.</ td >
</ tr >
</ ng-template >
</ p-table >
</ div >
|
app.component.ts
import { Component } from '@angular/core' ;
interface Person {
name: String,
age: Number,
profession: String,
mobile: String,
gender: String
}
@Component({
selector: 'app-root' ,
templateUrl: './app.component.html' ,
styles: [
`.even{
background-color: green;
color: white;
}
`
]
})
export class AppComponent {
persons: Person[] = [
{
name: "James" ,
age: 21,
profession: "Doctor" ,
mobile: "8731******90" ,
gender: "Male"
},
{
name: "Sam" ,
age: 18,
profession: "Marketer" ,
mobile: "8989******98" ,
gender: "Male"
},
{
name: "Thomas" ,
age: 27,
profession: "Businessman" ,
mobile: "9645******11" ,
gender: "Male"
},
{
name: "Kate" ,
age: 24,
profession: "Teacher" ,
mobile: "9345******46" ,
gender: "Female"
},
{
name: "Kumar" ,
age: 17,
profession: "Student" ,
mobile: "7824******78" ,
gender: "Male"
},
{
name: "Ethan" ,
age: 22,
profession: "Delivery Boy" ,
mobile: "9090******90" ,
gender: "Male"
},
];
}
|
app.module.ts
import { NgModule } from '@angular/core' ;
import { BrowserModule }
from '@angular/platform-browser' ;
import { BrowserAnimationsModule }
from '@angular/platform-browser/animations' ;
import { AppComponent } from './app.component' ;
import { TableModule } from 'primeng/table' ;
import { ButtonModule } from 'primeng/button' ;
@NgModule({
imports: [
BrowserModule,
BrowserAnimationsModule,
TableModule,
ButtonModule
],
declarations: [AppComponent],
bootstrap: [AppComponent],
})
export class AppModule { }
|
Output:
Reference: http://primefaces.org/primeng/table
Share your thoughts in the comments
Please Login to comment...