How to change Bootstrap Checkbox size ?
Last Updated :
02 May, 2024
Changing Bootstrap Checkbox size involves applying classes like form-check-input-lg for larger checkboxes or form-check-input-sm for smaller ones. Adjustments can be made through additional CSS styling as required.
Syntax:
<div class="form-check">
<input class="form-check-input"
type="checkbox" value="..." id="...">
<label class="form-check-label" for="...">
Content
</label>
</div>
Using input[type=”checkbox”]
- Make a basic structure of the web page using HTML. Add CDN link of Bootstrap from the official Bootstrap website.
- Here, we have used four check boxes and used various bootstrap classes to give them styling.
- Then, write <style> element inside <head> element to change the size of the Bootstrap checkbox.
- Then, the value of the width is 4.2em and the height is 4.2em inside <style> element for changing the size of the checkbox.
Example: The example used approach input[type=”checkbox”] for changing the Bootstrap checkbox size.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Bootstrap 5 Checks and radios Checks</title>
<link href=
"https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css"
rel="stylesheet"
integrity=
"sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC"
crossorigin="anonymous">
<style>
input[type="checkbox"] {
width: 4.2em;
height: 4.2em;
}
</style>
</head>
<body>
<div class="container">
<h2 class="text-center">
Bootstrap checkbox size
</h2>
<h3>Select Technology</h3>
<div class="form-check">
<input class="form-check-input"
type="checkbox"
value=""
id="html">
<label class="form-check-label"
for="html">
HTML
</label>
</div>
<br /><br />
<div class="form-check">
<input class="form-check-input"
type="checkbox"
value=""
id="css">
<label class="form-check-label"
for="css">
CSS
</label>
</div>
<br /><br />
<div class="form-check">
<input class="form-check-input"
type="checkbox"
value=""
id="js">
<label class="form-check-label"
for="js">
JavaScript
</label>
</div>
<br /><br />
<div class="form-check">
<input class="form-check-input"
type="checkbox"
value=""
id="bootstrap"
checked>
<label class="form-check-label"
for="bootstrap">
Bootstrap
</label>
</div>
</div>
</body>
</html>
Output:
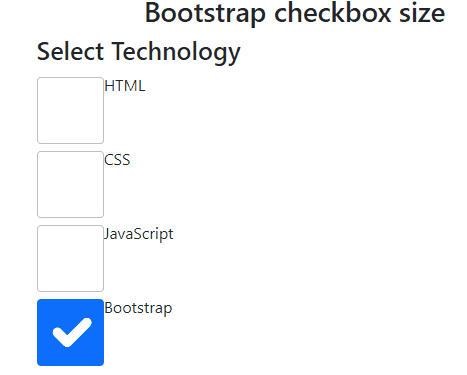
Bootstrap Checkbox Example
Using transform and scale
- Make a basic structure of the web page using HTML. Add CDN link of Bootstrap from the official Bootstrap website.
- Here, we have used four check boxes and used various bootstrap classes to give them styling.
- Then, write <style> element inside <head> element to change the size of the Bootstrap checkbox.
- There is the property transform having value scale(2) inside <style> element for changing the size of the checkbox.
Example: The example used the approach transform and scale for changing the Bootstrap checkbox size.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Bootstrap 5 Checks and radios Checks</title>
<link href=
"https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css"
rel="stylesheet"
integrity=
"sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC"
crossorigin="anonymous">
<style>
input[type="checkbox"] {
transform: scale(2);
}
</style>
</head>
<body>
<div class="container">
<h2 class="text-center">
Bootstrap checkbox size
</h2>
<h3>Select Technology</h3>
<div class="form-check">
<input class="form-check-input"
type="checkbox"
value=""
id="html">
<label class="form-check-label"
for="html">
HTML
</label>
</div>
<br /><br />
<div class="form-check">
<input class="form-check-input"
type="checkbox"
value=""
id="css">
<label class="form-check-label"
for="css">
CSS
</label>
</div>
<br /><br />
<div class="form-check">
<input class="form-check-input"
type="checkbox"
value=""
id="js">
<label class="form-check-label"
for="js">
JavaScript
</label>
</div>
<br /><br />
<div class="form-check">
<input class="form-check-input"
type="checkbox"
value=""
id="bootstrap" checked>
<label class="form-check-label"
for="bootstrap">
Bootstrap
</label>
</div>
</div>
</body>
</html>
Output:
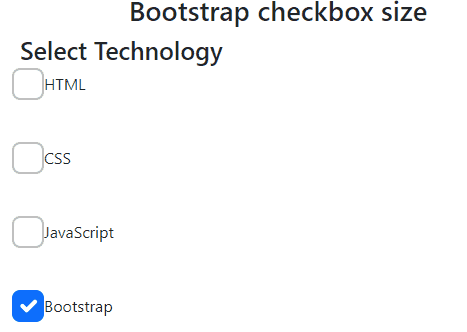
Bootstrap Checkbox Example Output
Using Inline CSS
- Make a basic structure of the web page using HTML. Add CDN link of Bootstrap from the official Bootstrap website.
- Here, we have used four check boxes and used various bootstrap classes to give them styling.
- Use Inline CSS to change the Bootstrap checkbox size.
- Then, the value of the width is 50px and the height is 50px for changing the size of the checkbox.
Example: The example used the approach Inline CSS for changing the Bootstrap checkbox size.
HTML
<!DOCTYPE html>
<html>
<head>
<title>Bootstrap 5 Checks and radios Checks</title>
<link href=
"https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css"
rel="stylesheet"
integrity=
"sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC"
crossorigin="anonymous">
</head>
<body>
<div class="container">
<h2 class="text-center">
Bootstrap checkbox
</h2>
<h3>Select Technology</h3>
<div class="form-check">
<input class="form-check-input"
type="checkbox"
value=""
id="html"
style="width:50px;height:50px">
<label class="form-check-label"
for="html">
Large HTML
</label>
</div>
<br /><br />
<div class="form-check">
<input class="form-check-input"
type="checkbox"
value=""
id="css">
<label class="form-check-label"
for="css">
Small CSS
</label>
</div>
<br /><br />
<div class="form-check">
<input class="form-check-input"
type="checkbox"
value=""
id="js"
style="width:70px;height:70px">
<label class="form-check-label"
for="js">
Large JavaScript
</label>
</div>
<br /><br />
<div class="form-check">
<input class="form-check-input"
type="checkbox"
value=""
id="bootstrap" checked>
<label class="form-check-label"
for="bootstrap">
Small Bootstrap
</label>
</div>
</div>
</body>
</html>
Output:
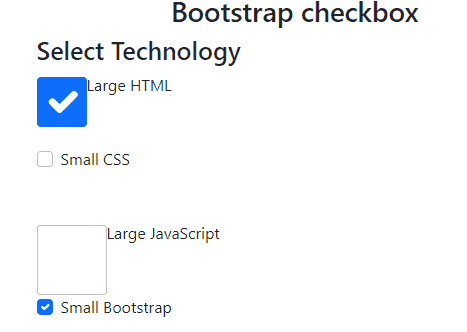
Bootstrap Checkbox size Example Output
Share your thoughts in the comments
Please Login to comment...