Web API URL
Last Updated :
26 Dec, 2023
The Web API URL is used to access and manipulate URLs. URL stands for Uniform Resource Locator. A URL is a unique address which is pointing to the resource. The URL is essential for making HTTP requests for making interactions with the API and for getting or sending data.
We will explore the above topics with their description and understand them with suitable examples.
Concepts and Usage
The structuring and parsing of the URL follow the URL standard. A URL is composed of different parts. Some of the important parts are described below.
Syntax
https://www.index.html:443/file1/file2/resouce.html?id=val&password=val2#anchor
Components
Scheme |
The HTTP part of the URL is the schema which indicates the protocol that the browser must follow to get the URL resource. |
Domain Name |
The www.index.html part is the domain name. It indicates the server that is being requested in place of the domain name it can be an IP address. |
Port |
The 443 part is the port number which is the internal gate to access resources. |
Path to resource |
The path /file1/file2/resouce.html is the path to the resource on the server. |
Parameters |
The ?id=val&password=val2 is the parameter part that is provided to a web server. |
Anchor |
The #anchor is the anchor part. It indicates the part of the resource we want to access. |
Accessing components of URLÂ
For a given URL, the object can be created & parsing can be done for the URL, along with facilitating access to its constituent portions through its properties quickly.
Syntax
// Creating the URL Object
let address = new URL("https://geeksforgeeks.com/login/username=val1&password=val2");
// Accessing the Host & Path Address
let host = address.host;
let path = address.pathname;
Here, we are trying to access the URL Object, Host, and Path Address for the given URL.
URL Modifications
To modify the value of the URLs that are represented by the object, we can simply add new values for them. For instance, from the below URL, we will modify the username and password, which will update the overall URL. Thus, the properties of the URL can be settable.
Syntax
// Changes the username and password attribute of the URL component.
let name = "geeks1";
let pswd = 'geek@123';
let url = new URL("https://geeksforgeeks.com/login");
url.username = name;
url.password = pswd;
Implementing the Queries
The search property of the URL component defines the portion of the query string. It allows one to look up the values of parameters. For instance, considering the below URL given, the search property of the URL component will be “username=val1&password=val2”. To search for the individual parameters, the URLSearchParams object’s get() method can be used.
Syntax
// The parameter username and password
// value which is pass with url.
let url = new URL("https://geeksforgeeks.com/login?username=val1&password=val2");
console.log( url.searchParams.get('username');
console.lgo(url.serachParams.get('password');
Example 1: In this example, we will parse the URL and see different parts of the URL. We will see the pathname, port number, and protocol.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Web API URL</ title >
</ head >
< body style = "text-align: center;" >
< h1 style = "color:green" >Welcome To GFG</ h1 >
< h2 >URL details</ h2 >
< h2 id = "ele" ></ h2 >
</ body >
< script >
const url = new URL(document.location.href);
document.getElementById("ele").innerHTML =
'< h3 >Path Name: ' + url.pathname +
'</ h3 >< h3 >Port: ' + url.port +
'</ h3 >' + '< h3 > Protocol ' +
url.protocol + '</ h3 >'
</ script >
</html
|
Output:
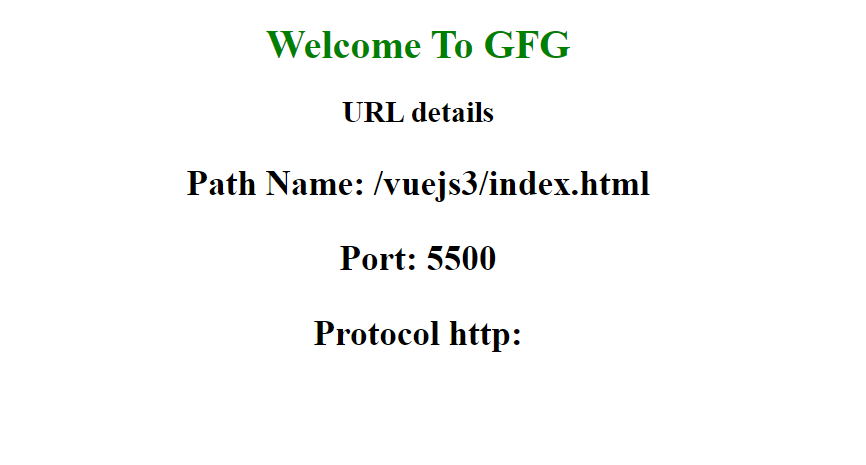
Â
Example 2: In this example, we change the URL component attribute, see host, hostname, and origin attribute of url component.
HTML
<!DOCTYPE html>
< html >
< head >
< title >Web API URL</ title >
</ head >
< body style = "text-align: center;" >
< h1 style = "color:green" >Welcome To GFG</ h1 >
< p >
< button onclick = "fun()" >Get host</ button >
< button onclick = "fun2()" >Get hostName</ button >
< button onclick = "fun3()" >Get Full URL</ button >
< button onclick = "fun4()" >Get UserName</ button >
</ p >
< h2 id = "ele" ></ h2 >
</ body >
< script >
const url = new URL(document.location.href);
url.username = 'geeks1';
url.password = 'geek@123';
function fun() {
document.getElementById('ele').innerHTML =
'< h3 >Host is : ' + url.host;
}
function fun2() {
document.getElementById('ele').innerHTML =
'< h3 >Host Name is : ' + url.hostname;
}
function fun3() {
document.getElementById('ele').innerHTML =
'< h3 >Full Url is : ' + url.origin;
}
function fun4() {
document.getElementById('ele').innerHTML =
'< h3 >User Name is: ' + url.username;
}
</ script >
</ html >
|
Output:
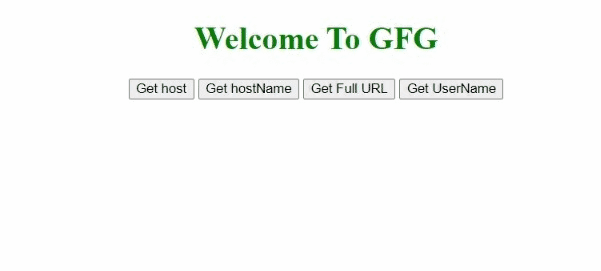
Browser Support
- Chrome 32
- Edge 12
- Firefox 19
- Opera 19
- Safari 7
Share your thoughts in the comments
Please Login to comment...