Vector setSize() method in Java with Example
Last Updated :
13 Jun, 2022
The Java.util.Vector.setSize() is a method of Vector class that is used to set the new size of the vector. If the new size of the vector is greater than the current size then null elements are added to the vector is new size is less than the current size then all higher-order elements are deleted.
This method modified the size of the Vector to the newSize and does not return anything.
Syntax:
public void setSize(int newSize)
Parameters: This method accepts a mandatory parameter newSize of the vector.
Return Type: NA
Exceptions: ArrayIndexOutOfBoundsException
Note: If new size is negative then it will throw Runtime Error
Example 1:
Java
import java.util.*;
public class GFG {
public static void main(String[] args)
{
Vector<String> v = new Vector<String>();
v.add( "Geeks" );
v.add( "for" );
v.add( "Geeks" );
v.add( "Computer" );
v.add( "Science" );
v.add( "Portal" );
System.out.println( "Initially" );
System.out.println( "Vector: " + v);
System.out.println( "Size: " + v.size());
v.setSize( 8 );
System.out.println( "\nAfter using setSize()" );
System.out.println( "Vector: " + v);
System.out.println( "Size: " + v.size());
}
}
|
Output
Initially
Vector: [Geeks, for, Geeks, Computer, Science, Portal]
Size: 6
After using setSize()
Vector: [Geeks, for, Geeks, Computer, Science, Portal, null, null]
Size: 8
Example 2: When new size is positive
Java
import java.util.*;
public class GFG {
public static void main(String[] args)
{
Vector<String> v = new Vector<String>();
v.add( "Geeks" );
v.add( "for" );
v.add( "Geeks" );
v.add( "Computer" );
v.add( "Science" );
v.add( "Portal" );
System.out.println( "Initially" );
System.out.println( "Vector: " + v);
System.out.println( "Size: " + v.size());
v.setSize( 4 );
System.out.println( "\nAfter using setSize()" );
System.out.println( "Vector: " + v);
System.out.println( "Size: " + v.size());
}
}
|
Output
Initially
Vector: [Geeks, for, Geeks, Computer, Science, Portal]
Size: 6
After using setSize()
Vector: [Geeks, for, Geeks, Computer]
Size: 4
Example 3: When the new size is negative
Java
import java.util.*;
public class GFG {
public static void main(String[] args)
{
Vector<String> v = new Vector<String>();
v.add( "Geeks" );
v.add( "for" );
v.add( "Geeks" );
v.add( "Computer" );
v.add( "Science" );
v.add( "Portal" );
System.out.println( "Initially" );
System.out.println( "Vector: " + v);
System.out.println( "Size: " + v.size());
try {
v.setSize(- 8 );
}
catch (Exception e) {
System.out.println( "Trying to change "
+ "size to '-8'\n" + e);
}
}
}
|
Output:
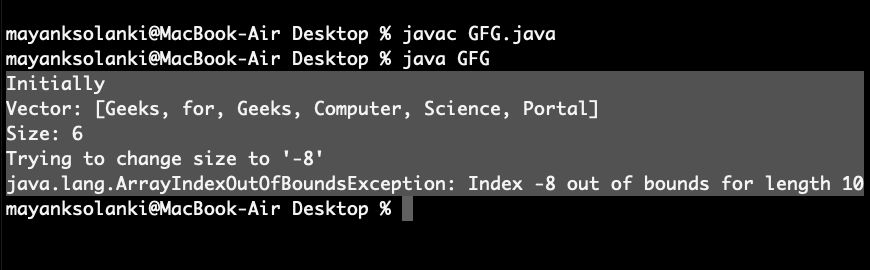
Share your thoughts in the comments
Please Login to comment...