Should we declare as Queue or Priority Queue while using Priority Queue in Java?
Last Updated :
21 Nov, 2022
Queue:
Queue is an Interface that extends the collection Interface in Java and this interface belongs to java.util package.
A queue is a type of data structure that follows the FIFO (first-in-first-out ) order. The queue contains ordered elements where insertion and deletion of elements are done at different ends.
Priority Queue and Linked List are some common classes that implement the Queue interface.
Syntax to declare Queue:
public interface Queue<E> extends Collection<E>
Some methods which can be performed on Queue interface are add( ), poll( ), peek( ), remove( ) and more.
Advantages:
- Queues can be used to implement other data structures like arrays and lists.
- Queues are very easy to use and implement.
- Easy to manage and operate on a huge amount of data.
Disadvantages:
- Queues have limited size as they are predefined.
- Searching for data in Queues is not easy.
PriorityQueue:
Priority Queue is a class that is defined in the java collection framework and implements the Queue interface. It also follows FIFO order but in this case elements in the queue are placed according to their priorities.
Syntax to declare Priority Queue:
public class PriorityQueue<E> extends AbstractQueue<E> implements Serializable
Advantages:
- Searching of data is easy as they are placed according to their priority.
- PriorityQueues are more efficiently organized
Disadvantages:
- Performing insertion operations on a priority queue take more time as they need to find the positions of elements according to their priority.
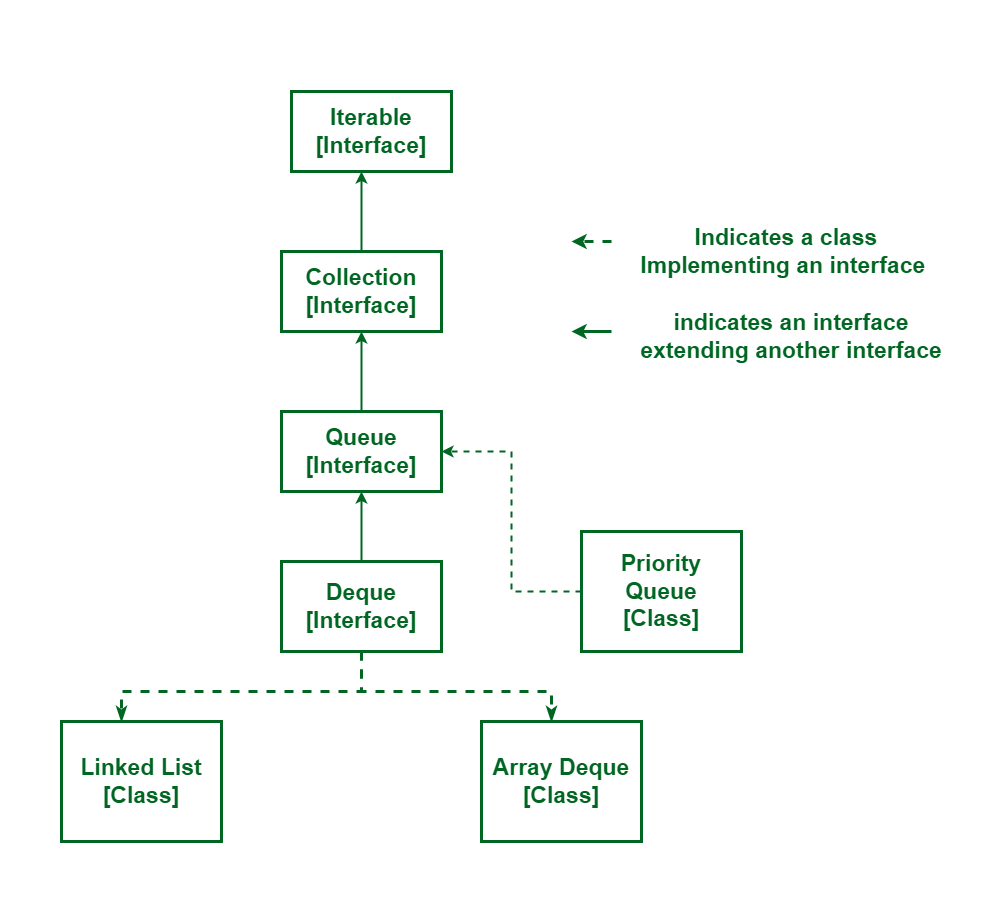
Java Framework for queue and priority queue
Here let us see whether we can use Queue or priority queue when we are using priority queue.
Case – 1:
PriorityQueue<Integer> pq = new PriorityQueue<>();
Advantages: By declaring the priority queue as priority queue we can quickly access the highest item with a time complexity of just O(1).
Disadvantages: Enqueue and dequeue operations on the priority queue are slow and also have a time complexity of O(log n).
Case – 2:
Queue<Integer> q = new PriorityQueue<>();
Advantages: By defining a priority queue as Queue we can change the actual implementation with a single line.
Disadvantages: In some cases, it will generate errors if our code includes priority functionalities where prioritization methods are absent because of declaring the priority queue as Queue.
Conclusion:
In many cases, the first case is mostly used but while writing our code if Queue do not use any functionality of prioritization then we can use Queue. In that way, we can change the actual implementation with one line. But if our code uses any priority functionalities then we must use the first case instead of the second. If we use the second case in the usage of priority features then it will produce errors as it will call for prioritization methods. In general, the first case should be used to avoid errors.
The efficient way of declaring PriorityQueue is case 1 which is to declare a priority queue as priorityQueue because it improves code readability and avoids causing errors.
Share your thoughts in the comments
Please Login to comment...