Program to construct DFA accepting odd number of 0s and odd number of 1s
Last Updated :
07 Jun, 2021
Given a binary string S, the task is to write a program for DFA Machine that accepts a string with odd numbers of 0s and 1s.
Examples:
Input: S = “010011”
Output: Accepted
Explanation:
The given string S contains odd number of zeros and ones.
Input: S = “00000”
Output: Not Accepted
Explanation:
The given string S doesn’t contains odd number of zeros and ones.
Approach: Below is the designed DFA Machine for the given problem. Construct a transition table for DFA states and analyze the transitions between each state. Below are the steps:
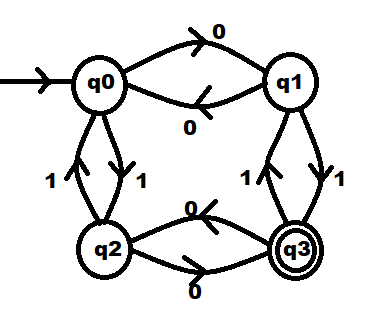
- There are 4 states q0, q1, q2, q3 where q0 is the initial state and q3 is the final state.
- The transition table of the above DFA is as follows:
Current state |
Final state |
0 |
1 |
q0 |
q1 |
q2 |
q1 |
q0 |
q3 |
q2 |
q3 |
q0 |
q3 |
q2 |
q1 |
- Through this table, understand the transitions in the DFA.
- If the final state(q3) is reached after reading the whole string, then the string is accepted otherwise not-accepted.
Below is the implementation of the above approach:
C++
#include <bits/stdc++.h>
using namespace std;
void checkValidDFA(string s)
{
int initial_state = 0;
int final_state;
int previous_state = 0;
for ( int i = 0; i < s.length(); i++) {
if ((s[i] == '0'
&& previous_state == 0)
|| (s[i] == '1'
&& previous_state == 3)) {
final_state = 1;
}
else if ((s[i] == '0'
&& previous_state == 3)
|| (s[i] == '1'
&& previous_state == 0)) {
final_state = 2;
}
else if ((s[i] == '0'
&& previous_state == 1)
|| (s[i] == '1'
&& previous_state == 2)) {
final_state = 0;
}
else if ((s[i] == '0'
&& previous_state == 2)
|| (s[i] == '1'
&& previous_state == 1)) {
final_state = 3;
}
previous_state = final_state;
}
if (final_state == 3) {
cout << "Accepted" << endl;
}
else {
cout << "Not Accepted" << endl;
}
}
int main()
{
string s = "010011" ;
checkValidDFA(s);
return 0;
}
|
Python3
def checkValidDFA(s):
initial_state = 0
final_state = 0
previous_state = 0
for i in range ( len (s)):
if ((s[i] = = '0' and previous_state = = 0 ) or
(s[i] = = '1' and previous_state = = 3 )):
final_state = 1
elif ((s[i] = = '0' and previous_state = = 3 ) or
(s[i] = = '1' and previous_state = = 0 )):
final_state = 2
elif ((s[i] = = '0' and previous_state = = 1 ) or
(s[i] = = '1' and previous_state = = 2 )):
final_state = 0
elif ((s[i] = = '0' and previous_state = = 2 ) or
(s[i] = = '1' and previous_state = = 1 )):
final_state = 3
previous_state = final_state
if (final_state = = 3 ):
print ( "Accepted" )
else :
print ( "Not Accepted" )
if __name__ = = '__main__' :
s = "010011"
checkValidDFA(s)
|
Java
import java.util.*;
class GFG{
static void checkValidDFA(String s)
{
int initial_state = 0 ;
int final_state = 0 ;
int previous_state = 0 ;
for ( int i = 0 ; i < s.length(); i++)
{
if ((s.charAt(i) == '0' && previous_state == 0 ) ||
(s.charAt(i) == '1' && previous_state == 3 ))
{
final_state = 1 ;
}
else if ((s.charAt(i) == '0' && previous_state == 3 ) ||
(s.charAt(i) == '1' && previous_state == 0 ))
{
final_state = 2 ;
}
else if ((s.charAt(i) == '0' && previous_state == 1 ) ||
(s.charAt(i) == '1' && previous_state == 2 ))
{
final_state = 0 ;
}
else if ((s.charAt(i) == '0' && previous_state == 2 ) ||
(s.charAt(i) == '1' && previous_state == 1 ))
{
final_state = 3 ;
}
previous_state = final_state;
}
if (final_state == 3 )
{
System.out.println( "Accepted" );
}
else
{
System.out.println( "Not Accepted" );
}
}
public static void main(String args[])
{
String s = "010011" ;
checkValidDFA(s);
}
}
|
C#
using System;
class GFG{
static void checkValidDFA( string s)
{
int final_state = 0;
int previous_state = 0;
for ( int i = 0; i < s.Length; i++)
{
if ((s[i] == '0' && previous_state == 0) ||
(s[i] == '1' && previous_state == 3))
{
final_state = 1;
}
else if ((s[i] == '0' && previous_state == 3) ||
(s[i] == '1' && previous_state == 0))
{
final_state = 2;
}
else if ((s[i] == '0' && previous_state == 1) ||
(s[i] == '1' && previous_state == 2))
{
final_state = 0;
}
else if ((s[i] == '0' && previous_state == 2) ||
(s[i] == '1' && previous_state == 1))
{
final_state = 3;
}
previous_state = final_state;
}
if (final_state == 3)
{
Console.WriteLine( "Accepted" );
}
else
{
Console.WriteLine( "Not Accepted" );
}
}
public static void Main()
{
string s = "010011" ;
checkValidDFA(s);
}
}
|
Javascript
<script>
function checkValidDFA(s) {
var final_state = 0;
var previous_state = 0;
for ( var i = 0; i < s.length; i++) {
if (
(s[i] === "0" && previous_state === 0) ||
(s[i] === "1" && previous_state === 3)
) {
final_state = 1;
} else if (
(s[i] === "0" && previous_state === 3) ||
(s[i] === "1" && previous_state === 0)
) {
final_state = 2;
} else if (
(s[i] === "0" && previous_state === 1) ||
(s[i] === "1" && previous_state === 2)
) {
final_state = 0;
} else if (
(s[i] === "0" && previous_state === 2) ||
(s[i] === "1" && previous_state === 1)
) {
final_state = 3;
}
previous_state = final_state;
}
if (final_state === 3) {
document.write( "Accepted" );
}
else {
document.write( "Not Accepted" );
}
}
var s = "010011" ;
checkValidDFA(s);
</script>
|
Time Complexity: O(N)
Auxiliary Space: O(1)
Share your thoughts in the comments
Please Login to comment...