Pick’s Theorem for Competitive Programming
Last Updated :
28 Apr, 2024
Pick’s Theorem is a mathematical theorem used in geometry, particularly in the field of computational geometry. It provides a method to calculate the area of lattice polygons, which are polygons whose vertices are points on a regular grid (lattice points).
Pick’s Theorem:
According to Pick’s Theorem We can calculate the area of any polygon by just counting the number of Interior and Boundary lattice points of that polygon. If number of interior points are I and number of boundary lattice points are B then Area (A) of polygon will be:
Area = I + B/2 – 1
- I : stands for the number of points in the interior of the shape,
- B : stands for the number of points on the boundary of the shape.
Take the polygon below as an example:
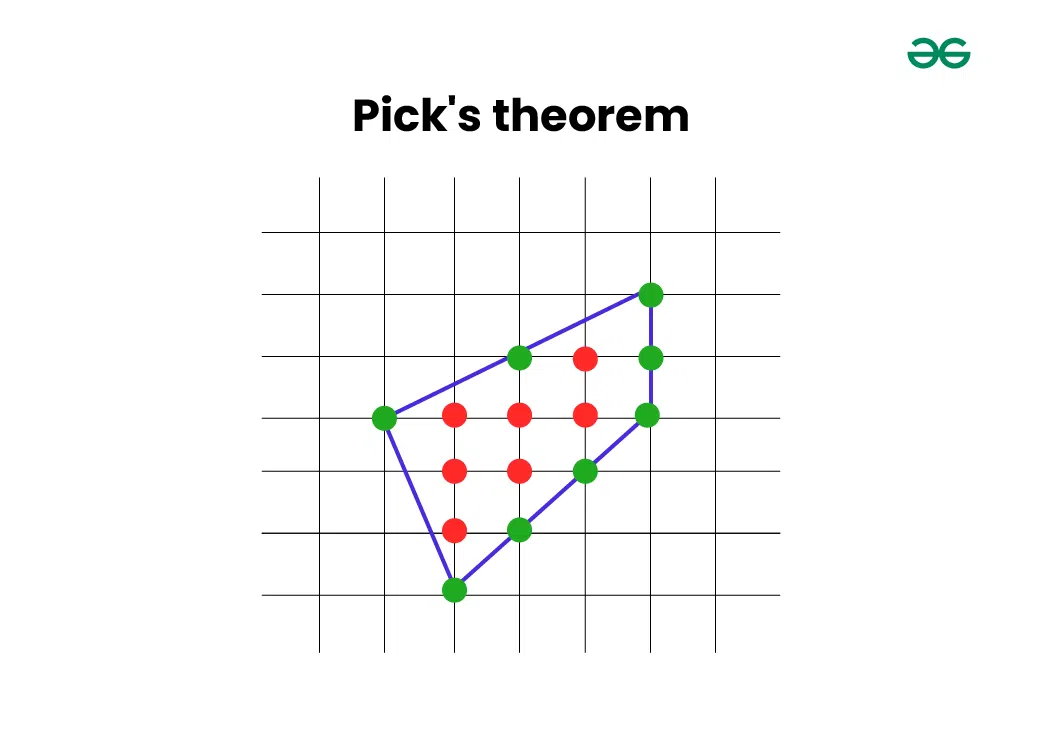
Picks Theorem
In this polygon:
According to Pick’s Theorem:
Area = 7 + (8)/2 – 1
= 10.
 which is the correct area of the polygon.
Idea Behind Pick’s Theorem:
- Counting Boundary Points: Traverse along the boundary of the polygon and count the number of lattice points lying on the edges.
- Counting Interior Points: Use a point-in-polygon test to determine the number of lattice points contained within the polygon.
- The theorem states that the area A of a lattice polygon with I interior lattice points and B boundary lattice points is given by the formula:
The idea behind Pick’s Theorem is to count the number of lattice points inside the polygon (I), the number of lattice points on the boundary (B), and then apply the formula to calculate the area.
Proof of PICK’s Algorithm:
The proof is based on the concept of triangulation. Any simple polygon can be divided into triangles, and the area of the polygon is the sum of the areas of these triangles. Since PICK’s Algorithm efficiently counts lattice points on triangles and the lattice points on the edges, it can accurately calculate the area of the polygon.
Example Problem:
Let’s take a right-angled triangle with vertices at (0, 0), (2, 0), and (0, 2),calculate and validate its area.
- Count of Boundary Points: There are 6 boundary lattice points: (0, 0), (1, 0), (2, 0), (0, 1), (0, 2), (1, 1)
- Count Interior Points: There are 0 interior lattice points.
- Apply PICK’s Algorithm:
- A = I + B/2 – 1 = 0 + 6/2 – 1 = 2.
also we know that, Mathematical formula for area of triangle = (1/2)*Base*Height = (1/2)*2*2 = 2.
Implementation:
Implementation of Pick’s Theorem involves counting the number of lattice points inside the polygon and on its boundary. This can be done using various algorithms such as the ray casting algorithm or the winding number algorithm. Once the counts are obtained, the area can be calculated using the formula mentioned above.
Below is the implementation of the pick’s algorithm:
C++
#include <cmath>
#include <iostream>
using namespace std;
// Function to calculate the area of a lattice polygon using
// Pick's Theorem
int pickArea(int i, int b) { return i + (b / 2) - 1; }
int main()
{
int interiorPoints, boundaryPoints;
// Input the number of interior and boundary lattice
// points
interiorPoints = 8;
boundaryPoints = 16;
// Calculate the area using Pick's Theorem
int area = pickArea(interiorPoints, boundaryPoints);
// Output the result
cout << "The area of the lattice polygon is: " << area
<< endl;
return 0;
}
Java
public class LatticePolygonArea {
// Function to calculate the area of a lattice polygon
// using Pick's Theorem
static int pickArea(int i, int b)
{
return i + (b / 2) - 1;
}
public static void main(String[] args)
{
int interiorPoints, boundaryPoints;
// Input the number of interior and boundary lattice
// points
interiorPoints = 8;
boundaryPoints = 16;
// Calculate the area using Pick's Theorem
int area = pickArea(interiorPoints, boundaryPoints);
// Output the result
System.out.println(
"The area of the lattice polygon is: " + area);
}
}
// This code is contributed by shivamgupta0987654321
Python3
import math
# Function to calculate the area of a lattice polygon using Pick's Theorem
def pick_area(i, b):
return i + (b // 2) - 1
def main():
# Input the number of interior and boundary lattice points
interior_points = 8
boundary_points = 16
# Calculate the area using Pick's Theorem
area = pick_area(interior_points, boundary_points)
# Output the result
print(f"The area of the lattice polygon is: {area}")
if __name__ == "__main__":
main()
JavaScript
// Function to calculate the area of a lattice polygon using Pick's Theorem
function pickArea(i, b) {
return i + Math.floor(b / 2) - 1;
}
// Main function
function main() {
let interiorPoints, boundaryPoints;
// Input the number of interior and boundary lattice points
interiorPoints = 8;
boundaryPoints = 16;
// Calculate the area using Pick's Theorem
let area = pickArea(interiorPoints, boundaryPoints);
// Output the result
console.log("The area of the lattice polygon is: " + area);
}
// Invoke the main function
main();
OutputThe area of the lattice polygon is: 15
Use Cases:
- Pick’s Theorem is used in various computational geometry problems where you need to calculate the area of lattice polygons efficiently.
- It can be used in problems involving counting lattice points in polygons or determining properties of lattice polygons.
Practice Problems:
Some practice problems involving Pick’s Theorem include:
Share your thoughts in the comments
Please Login to comment...